
test program
Dependencies: 25LCxxx_SPI mbed
main.cpp
00001 /** 00002 This code tests the latest version of the library Ser25lcxxx, using 00003 a 25LC256 eeprom (256KB) 00004 00005 author: Mario Bambagini 00006 */ 00007 00008 #include "mbed.h" 00009 00010 #include "Ser25lcxxx.h" 00011 00012 00013 void printMemory (unsigned char *s, int len); 00014 00015 void waitKey(); 00016 00017 00018 #define PAGESIZE 64 00019 #define PAGENUMBER 4096 00020 #define EEPROM_SIZE (PAGESIZE*PAGENUMBER) 00021 00022 #define BUFFER_SIZE (PAGESIZE*4) 00023 00024 00025 struct test_serialization { 00026 unsigned char data_1; 00027 unsigned int data_2; 00028 unsigned long data_3; 00029 }; 00030 00031 int main() 00032 { 00033 unsigned char buf[BUFFER_SIZE]; 00034 memset(buf, 0x00, BUFFER_SIZE); 00035 00036 printf("******* START *******\r\n\r\n"); 00037 00038 /**************************** INITIALIZATION ****************************/ 00039 printf("INIT\r\n"); 00040 Ser25LCxxx flash(p7, p5, p6, p9, PAGENUMBER, PAGESIZE); 00041 00042 waitKey(); 00043 00044 /****************************** CONFIG TEST ******************************/ 00045 printf("EEPROM fully writable: %s\r\n", 00046 (flash.isFullyWritable()?"YES":"NO")); 00047 printf("EEPROM half writable: %s\r\n",(flash.isHalfWritable()?"YES":"NO")); 00048 printf("EEPROM not writable: %s\r\n", (flash.isNotWritable()?"YES":"NO")); 00049 waitKey(); 00050 00051 /***************************** READING TEST *****************************/ 00052 printf("READ 2 pages:\r\n"); 00053 int num = flash.read(0, 2*PAGESIZE, buf); 00054 printMemory(buf,2*PAGESIZE); 00055 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00056 00057 waitKey(); 00058 00059 /***************************** WRITING TEST *****************************/ 00060 printf("WRITE first 20 bytes\r\n"); 00061 memset(buf, 0x01, 20); 00062 num = flash.write(0, 20, buf); 00063 printf("%d bytes: %s\r\n\r\n", num, ((num==20)?"OK":"NOT OK")); 00064 00065 printf("WRITE 10 bytes within a page (from addr 30)\r\n"); 00066 memset(buf, 0x02, 10); 00067 num = flash.write(30, 10, buf); 00068 printf("%d bytes: %s\r\n\r\n", num, ((num==10)?"OK":"NOT OK")); 00069 00070 printf("WRITE 30 bytes on two consecutive pages (from addr 50)\r\n"); 00071 memset(buf, 0x03, 30); 00072 num = flash.write(50, 30, buf); 00073 printf("%d bytes: %s\r\n\r\n", num, ((num==30)?"OK":"NOT OK")); 00074 00075 printf("READ 2 pages:\r\n"); 00076 num = flash.read(0, 2*PAGESIZE, buf); 00077 printMemory(buf,2*PAGESIZE); 00078 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00079 00080 waitKey(); 00081 00082 /*************************** ERASING PAGE TEST ***************************/ 00083 printf("ERASE second page\r\n"); 00084 num = flash.clearPage(1); 00085 printf("%d bytes: %s\r\n", num, ((num==PAGESIZE)?"OK":"NOT OK")); 00086 00087 printf("READ 2 pages:\r\n"); 00088 num = flash.read(0, 2*PAGESIZE, buf); 00089 printMemory(buf,2*PAGESIZE); 00090 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00091 00092 waitKey(); 00093 00094 /************************** SERIALIZATION TEST **************************/ 00095 struct test_serialization var_ser, var_deser; 00096 var_ser.data_1 = 0xAA; 00097 var_ser.data_2 = 0x7777; 00098 var_ser.data_3 = 0x33333333; 00099 printf("Serialize data at addr 20\r\n"); 00100 num = flash.write(20, sizeof(test_serialization), 00101 (const unsigned char*)(&var_ser)); 00102 printf("%d bytes: %s\r\n", num, 00103 ((num==sizeof(test_serialization))?"OK":"NOT OK")); 00104 var_deser.data_1 = 0; 00105 var_deser.data_2 = 0; 00106 var_deser.data_3 = 0; 00107 printf("De-serialize data from addr 20\r\n"); 00108 num = flash.read(20, sizeof(test_serialization), 00109 (unsigned char*)(&var_deser)); 00110 printf("%d bytes: %s\r\n", num, 00111 ((num==sizeof(test_serialization))?"OK":"NOT OK")); 00112 printf("Same data: %s\r\n", (((var_ser.data_1==var_deser.data_1) && 00113 (var_ser.data_2==var_deser.data_2) && 00114 (var_ser.data_3==var_deser.data_3))? 00115 "OK":"NOT OK")); 00116 printf("READ 2 pages:\r\n"); 00117 num = flash.read(0, 2*PAGESIZE, buf); 00118 printMemory(buf,2*PAGESIZE); 00119 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00120 00121 waitKey(); 00122 00123 /************************** ERASING MEMORY TEST **************************/ 00124 printf("ERASE memory\r\n"); 00125 num = flash.clearMem(); 00126 printf("%d bytes: %s\r\n", num, ((num==(EEPROM_SIZE))?"OK":"NOT OK")); 00127 00128 printf("READ 2 pages:\r\n"); 00129 num = flash.read(0, 2*PAGESIZE, buf); 00130 printMemory(buf,2*PAGESIZE); 00131 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00132 00133 waitKey(); 00134 00135 /**************************** PROTECTION TEST ****************************/ 00136 00137 printf("Setting whole EEPROM as not writable\r\n"); 00138 flash.setNotWritable(); 00139 00140 printf("EEPROM not writable: %s\r\n", (flash.isNotWritable()?"YES":"NO")); 00141 00142 printf("WRITE first page\r\n"); 00143 memset(buf, 0x00, PAGESIZE); 00144 num = flash.write(0, PAGESIZE, buf); 00145 printf("%d bytes: %s\r\n", num, ((num==(PAGESIZE))?"OK":"NOT OK")); 00146 00147 printf("READ 2 pages:\r\n"); 00148 num = flash.read(0, 2*PAGESIZE, buf); 00149 printMemory(buf,2*PAGESIZE); 00150 printf("%d bytes: %s\r\n", num, ((num==(2*PAGESIZE))?"OK":"NOT OK")); 00151 printf("DATA IS NOT CHANGED\r\n"); 00152 00153 printf("Setting whole EEPROM as writable\r\n"); 00154 flash.setFullyWritable(); 00155 00156 printf("\r\nDONE\r\n"); 00157 00158 while(1); 00159 } 00160 00161 void printMemory (unsigned char *s, int len) 00162 { 00163 int i = 0; 00164 while (i<len) { 00165 printf("%02X ", s[i]); 00166 if (((++i)%32)==0) 00167 printf("\r\n"); 00168 } 00169 } 00170 00171 void waitKey () 00172 { 00173 char a; 00174 printf("Press a key to continue...\r\n\r\n"); 00175 scanf("%c", &a); 00176 }
Generated on Sun Jul 24 2022 15:30:45 by
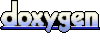