Enhanced EEPROM 25LCxxx driver
Fork of 25LCxxx_SPI by
Embed:
(wiki syntax)
Show/hide line numbers
Ser25lcxxx.h
00001 /* 00002 * Ser25lcxxx library 00003 * Copyright (c) 2010 Hendrik Lipka 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef __SER25LCXXX_H__ 00025 #define __SER25LCXXX_H__ 00026 00027 #include "mbed.h" 00028 00029 00030 #define EEPROM_CMD_READ 0x03 00031 #define EEPROM_CMD_WRITE 0x02 00032 #define EEPROM_CMD_WRDI 0x04 00033 #define EEPROM_CMD_WREN 0x06 00034 #define EEPROM_CMD_RDSR 0x05 00035 #define EEPROM_CMD_WRSR 0x01 00036 00037 00038 #define EEPROM_SPI_SPEED 1000000 00039 00040 #define EEPROM_CLEAN_BYTE 0xff 00041 00042 00043 /** 00044 A class to read and write all 25* serial SPI eeprom devices from Microchip 00045 (from 25xx010 to 25xx1024). 00046 00047 One needs to provide total size and page size, since this cannot be read from 00048 the devices, and the page size differs even by constant size (look up the data 00049 sheet for your part!) 00050 */ 00051 class Ser25LCxxx 00052 { 00053 public: 00054 00055 /** 00056 Create the handler class 00057 00058 @param sck clock pin of the spi where the eeprom is connected 00059 @param si input pin of the spi where the eeprom is connected 00060 @param so output pin of the spi where the eeprom is connected 00061 @param enable the pin name for the port where /CS is connected 00062 @param pagenumber number of pages 00063 @param pagesize the size of each page 00064 */ 00065 Ser25LCxxx(PinName sck, PinName si, PinName so, PinName enable, 00066 int pagenumber, int pagesize); 00067 00068 /** 00069 Destroy the handler 00070 */ 00071 ~Ser25LCxxx() {}; 00072 00073 /** 00074 Read from the eeprom memory 00075 00076 @param startAdr the adress where to start reading 00077 @param len the number of bytes to read 00078 @param buf destination buffer 00079 @return number of read bytes 00080 */ 00081 unsigned int read(unsigned int startAdr, unsigned int len, 00082 unsigned char* buf); 00083 00084 /** 00085 Writes the give buffer into the eeprom memory 00086 00087 @param startAdr the eeprom adress where to start writing (it 00088 doesn't have to match a page boundary) 00089 @param len number of bytes to write 00090 @param data data to write 00091 @return the number of written bytes 00092 */ 00093 unsigned int write(unsigned int startAdr, unsigned int len, 00094 const unsigned char* data); 00095 00096 /** 00097 Fills the given page with EEPROM_CLEAN_BYTE 00098 00099 @param pageNum the page to clear 00100 @return the number of written bytes (it should be the page size) 00101 */ 00102 unsigned int clearPage(unsigned int pageNum); 00103 00104 /** 00105 Fills the whole eeprom with EEPROM_CLEAN_BYTE 00106 00107 @return the number of written bytes (it should be the eeprom size) 00108 */ 00109 unsigned int clearMem(); 00110 00111 /** 00112 Return TRUE if a write is performing 00113 */ 00114 bool isWriteInProgress() { 00115 return (readStatus()&0x01)!=0x00; 00116 } 00117 00118 /** 00119 Return TRUE if the entire eeprom memory is writable 00120 */ 00121 bool isFullyWritable() { 00122 return (readStatus()&0x0C)==0x00; 00123 } 00124 00125 /** 00126 Return TRUE if only the first half of the eeprom memory is writable 00127 */ 00128 bool isHalfWritable() { 00129 return (readStatus()&0x0C)==0x08; 00130 } 00131 00132 /** 00133 Return TRUE if the eeprom memory is not writable 00134 */ 00135 bool isNotWritable() { 00136 return (readStatus()&0x0C)==0x0C; 00137 } 00138 00139 /** 00140 Set the eeprom memory not writable 00141 */ 00142 void setNotWritable () { 00143 writeStatus(0x0C); 00144 } 00145 00146 /** 00147 Set the eeprom memory fully writable 00148 */ 00149 void setFullyWritable () { 00150 writeStatus(0x00); 00151 } 00152 00153 /** 00154 Set only the first half of the eeprom memory as writable 00155 */ 00156 void setHalfWritable() { 00157 writeStatus(0x08); 00158 } 00159 00160 private: 00161 00162 /** 00163 Write data within an eeprom page 00164 00165 @param startAdr destination address of the eeprm memory 00166 @param len number of bytes to write 00167 @param data data to be written in the eeprom memory 00168 @return number of written bytes 00169 */ 00170 unsigned int writePage(unsigned int startAdr, unsigned int len, 00171 const unsigned char* data); 00172 00173 /** 00174 Read the status register of the eeprom memory 00175 00176 @return status register 00177 */ 00178 int readStatus(); 00179 00180 /** 00181 Write the status register 00182 00183 @param val status register 00184 */ 00185 void writeStatus(unsigned char val); 00186 00187 /** 00188 Wait until a write procedure ends or an interval of 5ms is expired 00189 00190 @return TRUE if the write procedure has successfully terminated, 00191 FALSE if the interval is expired 00192 */ 00193 bool waitForWrite(); 00194 00195 /** 00196 Enable the write procedure 00197 */ 00198 void enableWrite(); 00199 00200 SPI spi; 00201 DigitalOut cs; 00202 int size; 00203 int pageSize; 00204 int pageNumber; 00205 }; 00206 00207 #endif
Generated on Sun Jul 24 2022 01:34:27 by
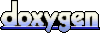