
Interrupt on user button press and timer exaple usage
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 /* 00003 * Button with interrupt example 00004 */ 00005 00006 InterruptIn mybutton(USER_BUTTON); 00007 DigitalOut myled(LED1); 00008 Timer t; 00009 00010 float delay = 5.0; // 1 sec 00011 00012 void pressed() 00013 { 00014 t.stop(); 00015 printf("You pressed after %f seconds\n", t.read()); 00016 if (delay == 5.0) 00017 delay = 0.2; // 200 ms 00018 else 00019 delay = 5.0; // 1 sec 00020 t.reset(); 00021 t.start(); 00022 } 00023 00024 int main() 00025 { 00026 t.start(); 00027 mybutton.fall(&pressed); 00028 while (1) { 00029 myled = !myled; //toggle the led 00030 wait(delay); 00031 } 00032 } 00033 00034 /* 00035 * Timeout version 00036 */ 00037 /* 00038 00039 DigitalOut led1(LED1); 00040 DigitalOut led2(LED2); 00041 Timeout timeout; 00042 00043 void flip() 00044 { 00045 led2 = !led2; 00046 } 00047 00048 int main() 00049 { 00050 led2 = 1; 00051 timeout.attach(&flip, 2.0); // setup flipper to call flip after 2 seconds 00052 00053 // spin in a main loop. flipper will interrupt it to call flip 00054 while(1) { 00055 led1 = !led1; 00056 wait(0.2); 00057 } 00058 } 00059 */
Generated on Sun Jul 17 2022 07:36:10 by
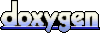