
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
wifi_tests.h
00001 #ifndef WIFI_TESTS_H 00002 #define WIFI_TESTS_H 00003 00004 #include "WiFiInterface.h" 00005 00006 /** Get WiFiInterface based on provided 00007 * app_json. */ 00008 WiFiInterface *get_interface(void); 00009 00010 /* 00011 * Test cases 00012 */ 00013 00014 /** Test that constructor of the driver works. */ 00015 void wifi_constructor(void); 00016 00017 /** This test case is to test whether the driver accepts valid credentials and reject ones that are not valid. */ 00018 void wifi_set_credential(void); 00019 00020 /** Test validity of WiFiInterface::set_channel(). */ 00021 void wifi_set_channel(void); 00022 00023 /** Test WiFiInterface::get_rssi() API. 00024 * When connected, it should return valid RSSI value. When unconnected it should return 0. */ 00025 void wifi_get_rssi(void); 00026 00027 /** Test WiFiInterface::connect(ssid, pass, security, channel) with NULL parameters */ 00028 void wifi_connect_params_null(void); 00029 00030 /** Test WiFiInterface::connect(ssid, pass, security) with valid parameters for unsecure network */ 00031 void wifi_connect_params_valid_unsecure(void); 00032 00033 /** Test WiFiInterface::connect(ssid, pass, security) with valid parameters for secure network */ 00034 void wifi_connect_params_valid_secure(void); 00035 00036 /** Test WiFiInterface::connect(ssid, pass, security, channel) with valid parameters for secure network using channel specified. */ 00037 void wifi_connect_params_channel(void); 00038 00039 /** Test WiFiInterface::connect(ssid, pass, security, channel) with valid parameters for secure network using wrong channel number. */ 00040 void wifi_connect_params_channel_fail(void); 00041 00042 /** Test WiFiInterface::connect() without parameters. Use set_credentials() for setting parameters. */ 00043 void wifi_connect(void); 00044 00045 /** Test WiFiInterface::connect() without parameters. Don't set parameters with set_credentials() */ 00046 void wifi_connect_nocredentials(void); 00047 00048 /** Test WiFiInterface::connect() without parameters. Use secure settings for set_credentials. */ 00049 void wifi_connect_secure(void); 00050 00051 /** Test WiFiInterface::connect() failing with wrong password. */ 00052 void wifi_connect_secure_fail(void); 00053 00054 /** Test WiFiInterface::connect() - disconnect() repeatition works. */ 00055 void wifi_connect_disconnect_repeat(void); 00056 00057 /** Call WiFiInterface::scan() with null parameters to get number of networks available. */ 00058 void wifi_scan_null(void); 00059 00060 /** Call WiFiInterface::scan() with valid accesspoint list allocated */ 00061 void wifi_scan(void); 00062 00063 #endif //WIFI_TESTS_H
Generated on Sun Jul 17 2022 08:25:33 by
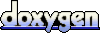