
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
wfi_auto.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import sys 00019 import uuid 00020 import time 00021 from sys import stdout 00022 00023 class WFITest(): 00024 00025 def test(self, selftest): 00026 c = selftest.mbed.serial_readline() 00027 00028 if c == None: 00029 selftest.notify("HOST: No output detected") 00030 return selftest.RESULT_IO_SERIAL 00031 00032 if c.strip() != "0": 00033 selftest.notify("HOST: Unexpected output. Expected '0' but received '%s'" % c.strip()) 00034 return selftest.RESULT_FAILURE 00035 00036 # Wait 10 seconds to allow serial prints (indicating failure) 00037 selftest.mbed.set_serial_timeout(10) 00038 00039 # If no characters received, pass the test 00040 if not selftest.mbed.serial_readline(): 00041 selftest.notify("HOST: No further output detected") 00042 return selftest.RESULT_SUCCESS 00043 else: 00044 selftest.notify("HOST: Extra output detected") 00045 return selftest.RESULT_FAILURE
Generated on Sun Jul 17 2022 08:25:33 by
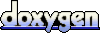