
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
wait_us_auto.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 from time import time 00019 00020 class WaitusTest (): 00021 """ This test is reading single characters from stdio 00022 and measures time between their occurrences. 00023 """ 00024 TICK_LOOP_COUNTER = 13 00025 TICK_LOOP_SUCCESSFUL_COUNTS = 10 00026 DEVIATION = 0.10 # +/-10% 00027 00028 def test(self, selftest): 00029 test_result = True 00030 # First character to start test (to know after reset when test starts) 00031 if selftest.mbed.set_serial_timeout(None) is None: 00032 return selftest.RESULT_IO_SERIAL 00033 c = selftest.mbed.serial_read(1) 00034 if c is None: 00035 return selftest.RESULT_IO_SERIAL 00036 if c == '$': # target will printout TargetID e.g.: $$$$1040e649d5c09a09a3f6bc568adef61375c6 00037 #Read additional 39 bytes of TargetID 00038 if selftest.mbed.serial_read(39) is None: 00039 return selftest.RESULT_IO_SERIAL 00040 c = selftest.mbed.serial_read(1) # Re-read first 'tick' 00041 if c is None: 00042 return selftest.RESULT_IO_SERIAL 00043 start_serial_pool = time() 00044 start = time() 00045 00046 success_counter = 0 00047 00048 for i in range(0, self.TICK_LOOP_COUNTER ): 00049 c = selftest.mbed.serial_read(1) 00050 if c is None: 00051 return selftest.RESULT_IO_SERIAL 00052 delta = time() - start 00053 deviation = abs(delta - 1) 00054 # Round values 00055 delta = round(delta, 2) 00056 deviation = round(deviation, 2) 00057 # Check if time measurements are in given range 00058 deviation_ok = True if delta > 0 and deviation <= self.DEVIATION else False 00059 success_counter = success_counter+1 if deviation_ok else 0 00060 msg = "OK" if deviation_ok else "FAIL" 00061 selftest.notify("%s in %.2f sec (%.2f) [%s]"% (c, delta, deviation, msg)) 00062 start = time() 00063 if success_counter >= self.TICK_LOOP_SUCCESSFUL_COUNTS : 00064 break 00065 measurement_time = time() - start_serial_pool 00066 selftest.notify("Consecutive OK timer reads: %d"% success_counter) 00067 selftest.notify("Completed in %.2f sec" % (measurement_time)) 00068 test_result = True if success_counter >= self.TICK_LOOP_SUCCESSFUL_COUNTS else False 00069 return selftest.RESULT_SUCCESS if test_result else selftest.RESULT_FAILURE
Generated on Sun Jul 17 2022 08:25:33 by
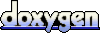