
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
uvisor_semaphore.c
00001 #include "api/inc/uvisor_semaphore_exports.h" 00002 #include "api/inc/uvisor_exports.h" 00003 #include "api/inc/halt_exports.h" 00004 #include "cmsis_os2.h" 00005 #include "rtx_lib.h" 00006 #include <string.h> 00007 00008 typedef struct uvisor_semaphore_internal { 00009 osSemaphoreId_t id; 00010 osSemaphoreAttr_t attr; 00011 osRtxSemaphore_t data; 00012 } UVISOR_ALIGN(4) uvisor_semaphore_internal_t; 00013 00014 UVISOR_STATIC_ASSERT(UVISOR_SEMAPHORE_INTERNAL_SIZE >= sizeof(UvisorSemaphore), semaphore_size_too_small); 00015 00016 int __uvisor_semaphore_init(UvisorSemaphore * s, uint32_t max_count, uint32_t initial_count) 00017 { 00018 uvisor_semaphore_internal_t * semaphore = (uvisor_semaphore_internal_t *) s; 00019 00020 memset(&semaphore->data, 0, sizeof(semaphore->data)); 00021 memset(&semaphore->attr, 0, sizeof(semaphore->attr)); 00022 semaphore->attr.name = "uvisor_semaphore"; 00023 semaphore->attr.cb_size = sizeof(semaphore->data); 00024 semaphore->attr.cb_mem = &semaphore->data; 00025 semaphore->id = osSemaphoreNew(max_count, initial_count, &semaphore->attr); 00026 00027 /* Error when semaphore->id is NULL */ 00028 return semaphore->id == NULL ? UVISOR_ERROR_OUT_OF_STRUCTURES : 0; 00029 } 00030 00031 int __uvisor_semaphore_pend(UvisorSemaphore * s, uint32_t timeout_ms) 00032 { 00033 uvisor_semaphore_internal_t * semaphore = (uvisor_semaphore_internal_t *) s; 00034 00035 osStatus_t status = osSemaphoreAcquire(semaphore->id, timeout_ms); 00036 00037 if (status == osErrorTimeout) { 00038 return UVISOR_ERROR_TIMEOUT; 00039 } else if (status == osErrorParameter) { 00040 return UVISOR_ERROR_INVALID_PARAMETERS; 00041 } else if (status != osOK) { 00042 return -1; /* Other error */ 00043 } 00044 00045 return 0; 00046 } 00047 00048 int __uvisor_semaphore_post(UvisorSemaphore * s) { 00049 uvisor_semaphore_internal_t * semaphore = (uvisor_semaphore_internal_t *) s; 00050 return osSemaphoreRelease(semaphore->id); 00051 }
Generated on Sun Jul 17 2022 08:25:33 by
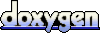