
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_specification.h
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #ifndef UTEST_SPECIFICATION_H 00020 #define UTEST_SPECIFICATION_H 00021 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 #include <stdio.h> 00025 #include "utest/utest_types.h" 00026 #include "utest/utest_case.h" 00027 #include "utest/utest_default_handlers.h" 00028 00029 00030 namespace utest { 00031 /** \addtogroup frameworks */ 00032 /** @{*/ 00033 namespace v1 { 00034 00035 /** Test specification containing the setup and teardown handlers and test cases. 00036 * 00037 * This class simply holds the test cases and allows you to specify default handlers, and 00038 * override setup and teardown handlers. 00039 * The order of arguments is: 00040 * - test setup handler (optional) 00041 * - array of test cases (required) 00042 * - test teardown handler (optional) 00043 * - default handlers (optional) 00044 * 00045 * @note You cannot set the size of the test case array dynamically, it is template deducted at compile 00046 * time. Creating test specifications for unittests at runtime is explicitly not supported. 00047 */ 00048 class Specification 00049 { 00050 public: 00051 template< size_t N, typename CaseType > 00052 Specification(const CaseType (&cases)[N], 00053 const handlers_t defaults = default_handlers) : 00054 setup_handler(default_handler), teardown_handler(default_handler), failure_handler(default_handler), 00055 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00056 defaults(defaults) 00057 { 00058 MBED_STATIC_ASSERT( 00059 sizeof(CaseType) == sizeof(Case), 00060 "CaseType and Case should have the same size" 00061 ); 00062 } 00063 00064 template< size_t N, typename CaseType > 00065 Specification(const CaseType (&cases)[N], 00066 const test_failure_handler_t failure_handler, 00067 const handlers_t defaults = default_handlers) : 00068 setup_handler(default_handler), teardown_handler(default_handler), failure_handler(failure_handler), 00069 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00070 defaults(defaults) 00071 { 00072 MBED_STATIC_ASSERT( 00073 sizeof(CaseType) == sizeof(Case), 00074 "CaseType and Case should have the same size" 00075 ); 00076 } 00077 00078 template< size_t N, typename CaseType > 00079 Specification(const CaseType (&cases)[N], 00080 const test_teardown_handler_t teardown_handler, 00081 const handlers_t defaults = default_handlers) : 00082 setup_handler(default_handler), teardown_handler(teardown_handler), failure_handler(default_handler), 00083 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00084 defaults(defaults) 00085 { 00086 MBED_STATIC_ASSERT( 00087 sizeof(CaseType) == sizeof(Case), 00088 "CaseType and Case should have the same size" 00089 ); 00090 } 00091 00092 template< size_t N, typename CaseType > 00093 Specification(const CaseType (&cases)[N], 00094 const test_teardown_handler_t teardown_handler, 00095 const test_failure_handler_t failure_handler, 00096 const handlers_t defaults = default_handlers) : 00097 setup_handler(default_handler), teardown_handler(teardown_handler), failure_handler(failure_handler), 00098 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00099 defaults(defaults) 00100 { 00101 MBED_STATIC_ASSERT( 00102 sizeof(CaseType) == sizeof(Case), 00103 "CaseType and Case should have the same size" 00104 ); 00105 } 00106 00107 template< size_t N, typename CaseType > 00108 Specification(const test_setup_handler_t setup_handler, 00109 const CaseType (&cases)[N], 00110 const handlers_t defaults = default_handlers) : 00111 setup_handler(setup_handler), teardown_handler(default_handler), failure_handler(default_handler), 00112 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00113 defaults(defaults) 00114 {} 00115 00116 template< size_t N, typename CaseType > 00117 Specification(const test_setup_handler_t setup_handler, 00118 const CaseType (&cases)[N], 00119 const test_failure_handler_t failure_handler, 00120 const handlers_t defaults = default_handlers) : 00121 setup_handler(setup_handler), teardown_handler(default_handler), failure_handler(failure_handler), 00122 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00123 defaults(defaults) 00124 { 00125 MBED_STATIC_ASSERT( 00126 sizeof(CaseType) == sizeof(Case), 00127 "CaseType and Case should have the same size" 00128 ); 00129 } 00130 00131 template< size_t N, typename CaseType > 00132 Specification(const test_setup_handler_t setup_handler, 00133 const CaseType (&cases)[N], 00134 const test_teardown_handler_t teardown_handler, 00135 const handlers_t defaults = default_handlers) : 00136 setup_handler(setup_handler), teardown_handler(teardown_handler), failure_handler(default_handler), 00137 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00138 defaults(defaults) 00139 { 00140 MBED_STATIC_ASSERT( 00141 sizeof(CaseType) == sizeof(Case), 00142 "CaseType and Case should have the same size" 00143 ); 00144 } 00145 00146 template< size_t N, typename CaseType > 00147 Specification(const test_setup_handler_t setup_handler, 00148 const CaseType (&cases)[N], 00149 const test_teardown_handler_t teardown_handler, 00150 const test_failure_handler_t failure_handler, 00151 const handlers_t defaults = default_handlers) : 00152 setup_handler(setup_handler), teardown_handler(teardown_handler), failure_handler(failure_handler), 00153 cases(static_cast<const Case*>(static_cast<const CaseType*>(cases))), length(N), 00154 defaults(defaults) 00155 { 00156 MBED_STATIC_ASSERT( 00157 sizeof(CaseType) == sizeof(Case), 00158 "CaseType and Case should have the same size" 00159 ); 00160 } 00161 00162 Specification(const test_setup_handler_t setup_handler, 00163 const Case *cases, 00164 const size_t length, 00165 const test_teardown_handler_t teardown_handler, 00166 const test_failure_handler_t failure_handler, 00167 const handlers_t defaults = default_handlers) : 00168 setup_handler(setup_handler), teardown_handler(teardown_handler), failure_handler(failure_handler), 00169 cases(cases), length(length), 00170 defaults(defaults) 00171 { 00172 } 00173 00174 private: 00175 const test_setup_handler_t setup_handler; 00176 const test_teardown_handler_t teardown_handler; 00177 const test_failure_handler_t failure_handler; 00178 const Case *const cases; 00179 const size_t length; 00180 const handlers_t defaults; 00181 00182 friend class Harness; 00183 }; 00184 00185 } // namespace v1 00186 } // namespace utest 00187 00188 #endif // UTEST_SPECIFICATION_H 00189 00190 /** @}*/
Generated on Sun Jul 17 2022 08:25:33 by
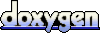