
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_scheduler.h
00001 00002 /** \addtogroup frameworks */ 00003 /** @{*/ 00004 /**************************************************************************** 00005 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00006 * SPDX-License-Identifier: Apache-2.0 00007 * 00008 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00009 * not use this file except in compliance with the License. 00010 * You may obtain a copy of the License at 00011 * 00012 * http://www.apache.org/licenses/LICENSE-2.0 00013 * 00014 * Unless required by applicable law or agreed to in writing, software 00015 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00016 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 **************************************************************************** 00020 */ 00021 00022 #ifndef UTEST_SCHEDULER_H 00023 #define UTEST_SCHEDULER_H 00024 00025 #include "mbed.h" 00026 #include <stdint.h> 00027 #include <stdbool.h> 00028 #include <stdio.h> 00029 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif 00033 00034 /** 00035 * The utest harness manages its own state and therefore does not require the scheduler to 00036 * bind any arguments to the scheduled callback. 00037 */ 00038 typedef void (*utest_v1_harness_callback_t)(void); 00039 00040 /** 00041 * utest calls this function before running the test specification. 00042 * Use this function to initialize your scheduler before the first callback is requested. 00043 * 00044 * @retval `0` if success 00045 * @retval non-zero if failure 00046 */ 00047 typedef int32_t (*utest_v1_scheduler_init_callback_t)(void); 00048 00049 /** 00050 * utest calls this function when it needs to schedule a callback with a delay in milliseconds. 00051 * `delay_ms` will only be non-zero if an asynchronous test case exists in the test specification. 00052 * @note If your scheduler cannot provide asynchronous callbacks (which probably require a hardware timer), 00053 * then this scheduler may return `NULL` as a handle and `utest` will fail the asynchronous request and move on. 00054 * Note that test cases which do not require asynchronous callback support will still work fine then. 00055 * 00056 * @warning You MUST NOT execute the callback inside this function, even for a delay of 0ms. 00057 * Buffer the callback and call it in your main loop. 00058 * @warning You MUST NOT execute the callback in an interrupt context! 00059 * Buffer the callback and call it in your main loop. 00060 * @note utest only schedules one callback at any given time. 00061 * This should make the implementation of this scheduler a lot simpler for you. 00062 * 00063 * @param callback the pointer to the callback function 00064 * @param delay_ms the delay in milliseconds after which the callback should be executed 00065 * @return A handle to identify the scheduled callback, or `NULL` for failure. 00066 */ 00067 typedef void *(*utest_v1_scheduler_post_callback_t)(const utest_v1_harness_callback_t callback, timestamp_t delay_ms); 00068 00069 /** 00070 * utest needs to cancel callbacks with a non-zero delay some time later. 00071 * Even though `utest` only schedules one callback at any given time, it can cancel a callback more than once. 00072 * You should therefore make use of the handle to make sure you do not cancel the wrong callback. 00073 * 00074 * @note If your scheduler cannot provide asynchronous callbacks, do nothing in this function and return non-zero. 00075 * 00076 * @param handle the handle returned from the `post` call to identify which callback to be cancelled. 00077 * @retval `0` if success 00078 * @retval non-zero if failure 00079 */ 00080 typedef int32_t (*utest_v1_scheduler_cancel_callback_t)(void *handle); 00081 00082 /** 00083 * utest calls this function at the end of the `Harness::run()` function, after (!) the first callback has been requested. 00084 * This function is meant to implement an optional event loop, which may very well be blocking (if your scheduler works with that). 00085 * This assumes that `Harness::run()` will be called on the main stack (ie. not in an interrupt!). 00086 * 00087 * @retval `0` if success 00088 * @retval non-zero if failure 00089 */ 00090 typedef int32_t (*utest_v1_scheduler_run_callback_t)(void); 00091 00092 /** 00093 * The scheduler interface consists out of the `post` and `cancel` functions, 00094 * which you must implement to use `utest`. 00095 */ 00096 typedef struct { 00097 utest_v1_scheduler_init_callback_t init; 00098 utest_v1_scheduler_post_callback_t post; 00099 utest_v1_scheduler_cancel_callback_t cancel; 00100 utest_v1_scheduler_run_callback_t run; 00101 } utest_v1_scheduler_t; 00102 00103 #ifdef __cplusplus 00104 } 00105 #endif 00106 00107 #endif // UTEST_SCHEDULER_H 00108 00109 /** @}*/
Generated on Sun Jul 17 2022 08:25:33 by
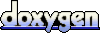