
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
utest_default_handlers.cpp
00001 /**************************************************************************** 00002 * Copyright (c) 2015, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 **************************************************************************** 00017 */ 00018 00019 #include "utest/utest_default_handlers.h" 00020 #include "utest/utest_case.h" 00021 #include "utest/utest_stack_trace.h" 00022 #include "utest/utest_serial.h" 00023 00024 using namespace utest::v1; 00025 00026 static void test_failure_handler(const failure_t failure); 00027 00028 const handlers_t utest::v1::verbose_continue_handlers = { 00029 verbose_test_setup_handler, 00030 verbose_test_teardown_handler, 00031 test_failure_handler, 00032 verbose_case_setup_handler, 00033 verbose_case_teardown_handler, 00034 verbose_case_failure_handler 00035 }; 00036 00037 const handlers_t& utest::v1::default_handlers = greentea_abort_handlers; 00038 00039 // --- SPECIAL HANDLERS --- 00040 static void test_failure_handler(const failure_t failure) { 00041 UTEST_LOG_FUNCTION(); 00042 if (failure.location == LOCATION_TEST_SETUP || failure.location == LOCATION_TEST_TEARDOWN) { 00043 verbose_test_failure_handler(failure); 00044 utest_printf("{{failure}}\n{{end}}\n"); 00045 while(1) ; 00046 } 00047 } 00048 00049 // --- VERBOSE TEST HANDLERS --- 00050 utest::v1::status_t utest::v1::verbose_test_setup_handler(const size_t number_of_cases) 00051 { 00052 UTEST_LOG_FUNCTION(); 00053 utest_printf(">>> Running %u test cases...\n", number_of_cases); 00054 return STATUS_CONTINUE; 00055 } 00056 00057 void utest::v1::verbose_test_teardown_handler(const size_t passed, const size_t failed, const failure_t failure) 00058 { 00059 UTEST_LOG_FUNCTION(); 00060 utest_printf("\n>>> Test cases: %u passed, %u failed", passed, failed); 00061 if (failure.reason == REASON_NONE) { 00062 utest_printf("\n"); 00063 } else { 00064 utest_printf(" with reason '%s'\n", stringify(failure.reason)); 00065 } 00066 if (failed) utest_printf(">>> TESTS FAILED!\n"); 00067 } 00068 00069 void utest::v1::verbose_test_failure_handler(const failure_t failure) 00070 { 00071 utest_printf(">>> failure with reason '%s' during '%s'\n", stringify(failure.reason), stringify(failure.location)); 00072 00073 } 00074 00075 // --- VERBOSE CASE HANDLERS --- 00076 utest::v1::status_t utest::v1::verbose_case_setup_handler(const Case *const source, const size_t index_of_case) 00077 { 00078 UTEST_LOG_FUNCTION(); 00079 utest_printf("\n>>> Running case #%u: '%s'...\n", index_of_case + 1, source->get_description ()); 00080 return STATUS_CONTINUE; 00081 } 00082 00083 utest::v1::status_t utest::v1::verbose_case_teardown_handler(const Case *const source, const size_t passed, const size_t failed, const failure_t failure) 00084 { 00085 UTEST_LOG_FUNCTION(); 00086 utest_printf(">>> '%s': %u passed, %u failed", source->get_description (), passed, failed); 00087 if (failure.reason == REASON_NONE) { 00088 utest_printf("\n"); 00089 } else { 00090 utest_printf(" with reason '%s'\n", stringify(failure.reason)); 00091 } 00092 return STATUS_CONTINUE; 00093 } 00094 00095 utest::v1::status_t utest::v1::verbose_case_failure_handler(const Case *const /*source*/, const failure_t failure) 00096 { 00097 UTEST_LOG_FUNCTION(); 00098 if (!(failure.reason & REASON_ASSERTION)) { 00099 verbose_test_failure_handler(failure); 00100 } 00101 if (failure.reason & (REASON_TEST_TEARDOWN | REASON_CASE_TEARDOWN)) return STATUS_ABORT; 00102 if (failure.reason & REASON_IGNORE) return STATUS_IGNORE; 00103 return STATUS_CONTINUE; 00104 }
Generated on Sun Jul 17 2022 08:25:33 by
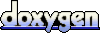