
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
upap.h
00001 /* 00002 * upap.h - User/Password Authentication Protocol definitions. 00003 * 00004 * Copyright (c) 1984-2000 Carnegie Mellon University. All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * 1. Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * 2. Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in 00015 * the documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * 3. The name "Carnegie Mellon University" must not be used to 00019 * endorse or promote products derived from this software without 00020 * prior written permission. For permission or any legal 00021 * details, please contact 00022 * Office of Technology Transfer 00023 * Carnegie Mellon University 00024 * 5000 Forbes Avenue 00025 * Pittsburgh, PA 15213-3890 00026 * (412) 268-4387, fax: (412) 268-7395 00027 * tech-transfer@andrew.cmu.edu 00028 * 00029 * 4. Redistributions of any form whatsoever must retain the following 00030 * acknowledgment: 00031 * "This product includes software developed by Computing Services 00032 * at Carnegie Mellon University (http://www.cmu.edu/computing/)." 00033 * 00034 * CARNEGIE MELLON UNIVERSITY DISCLAIMS ALL WARRANTIES WITH REGARD TO 00035 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00036 * AND FITNESS, IN NO EVENT SHALL CARNEGIE MELLON UNIVERSITY BE LIABLE 00037 * FOR ANY SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00038 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00039 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00040 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00041 * 00042 * $Id: upap.h,v 1.8 2002/12/04 23:03:33 paulus Exp $ 00043 */ 00044 00045 #include "netif/ppp/ppp_opts.h" 00046 #if PPP_SUPPORT && PAP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00047 00048 #ifndef UPAP_H 00049 #define UPAP_H 00050 00051 #include "ppp.h" 00052 00053 /* 00054 * Packet header = Code, id, length. 00055 */ 00056 #define UPAP_HEADERLEN 4 00057 00058 00059 /* 00060 * UPAP codes. 00061 */ 00062 #define UPAP_AUTHREQ 1 /* Authenticate-Request */ 00063 #define UPAP_AUTHACK 2 /* Authenticate-Ack */ 00064 #define UPAP_AUTHNAK 3 /* Authenticate-Nak */ 00065 00066 00067 /* 00068 * Client states. 00069 */ 00070 #define UPAPCS_INITIAL 0 /* Connection down */ 00071 #define UPAPCS_CLOSED 1 /* Connection up, haven't requested auth */ 00072 #define UPAPCS_PENDING 2 /* Connection down, have requested auth */ 00073 #define UPAPCS_AUTHREQ 3 /* We've sent an Authenticate-Request */ 00074 #define UPAPCS_OPEN 4 /* We've received an Ack */ 00075 #define UPAPCS_BADAUTH 5 /* We've received a Nak */ 00076 00077 /* 00078 * Server states. 00079 */ 00080 #define UPAPSS_INITIAL 0 /* Connection down */ 00081 #define UPAPSS_CLOSED 1 /* Connection up, haven't requested auth */ 00082 #define UPAPSS_PENDING 2 /* Connection down, have requested auth */ 00083 #define UPAPSS_LISTEN 3 /* Listening for an Authenticate */ 00084 #define UPAPSS_OPEN 4 /* We've sent an Ack */ 00085 #define UPAPSS_BADAUTH 5 /* We've sent a Nak */ 00086 00087 00088 /* 00089 * Timeouts. 00090 */ 00091 #if 0 /* moved to ppp_opts.h */ 00092 #define UPAP_DEFTIMEOUT 3 /* Timeout (seconds) for retransmitting req */ 00093 #define UPAP_DEFREQTIME 30 /* Time to wait for auth-req from peer */ 00094 #endif /* moved to ppp_opts.h */ 00095 00096 /* 00097 * Each interface is described by upap structure. 00098 */ 00099 #if PAP_SUPPORT 00100 typedef struct upap_state { 00101 const char *us_user; /* User */ 00102 u8_t us_userlen; /* User length */ 00103 const char *us_passwd; /* Password */ 00104 u8_t us_passwdlen; /* Password length */ 00105 u8_t us_clientstate; /* Client state */ 00106 #if PPP_SERVER 00107 u8_t us_serverstate; /* Server state */ 00108 #endif /* PPP_SERVER */ 00109 u8_t us_id; /* Current id */ 00110 u8_t us_transmits; /* Number of auth-reqs sent */ 00111 } upap_state; 00112 #endif /* PAP_SUPPORT */ 00113 00114 00115 void upap_authwithpeer(ppp_pcb *pcb, const char *user, const char *password); 00116 #if PPP_SERVER 00117 void upap_authpeer(ppp_pcb *pcb); 00118 #endif /* PPP_SERVER */ 00119 00120 extern const struct protent pap_protent; 00121 00122 #endif /* UPAP_H */ 00123 #endif /* PPP_SUPPORT && PAP_SUPPORT */
Generated on Sun Jul 17 2022 08:25:32 by
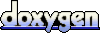