
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
udpecho_client.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from socket import socket, AF_INET, SOCK_DGRAM 00018 import string, random 00019 from time import time 00020 00021 from mbed_settings import CLIENT_ADDRESS 00022 00023 ECHO_PORT = 7 00024 00025 LEN_PACKET = 127 00026 N_PACKETS = 5000 00027 TOT_BITS = float(LEN_PACKET * N_PACKETS * 8) * 2 00028 MEGA = float(1024 * 1024) 00029 UPDATE_STEP = (N_PACKETS/10) 00030 00031 class UDP_EchoClient: 00032 s = socket(AF_INET, SOCK_DGRAM) 00033 00034 def __init__(self, host): 00035 self.host = host 00036 self.packet = ''.join(random.choice(string.ascii_uppercase + string.digits) for _ in range(LEN_PACKET)) 00037 00038 def __packet(self): 00039 # Comment out the checks when measuring the throughput 00040 # packet = ''.join(random.choice(string.ascii_uppercase + string.digits) for _ in range(LEN_PACKET)) 00041 UDP_EchoClient.s.sendto(packet, (self.host, ECHO_PORT)) 00042 data = UDP_EchoClient.s.recv(LEN_PACKET) 00043 # assert packet == data, "packet error:\n%s\n%s\n" % (packet, data) 00044 00045 def test(self): 00046 start = time() 00047 for i in range(N_PACKETS): 00048 if (i % UPDATE_STEP) == 0: print '%.2f%%' % ((float(i)/float(N_PACKETS)) * 100.) 00049 self.__packet() 00050 t = time() - start 00051 print 'Throughput: (%.2f)Mbits/s' % ((TOT_BITS / t)/MEGA) 00052 00053 while True: 00054 e = UDP_EchoClient(CLIENT_ADDRESS) 00055 e.test()
Generated on Sun Jul 17 2022 08:25:32 by
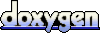