
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ticker_api.h
00001 00002 /** \addtogroup hal */ 00003 /** @{*/ 00004 /* mbed Microcontroller Library 00005 * Copyright (c) 2015 ARM Limited 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_TICKER_API_H 00020 #define MBED_TICKER_API_H 00021 00022 #include <stdint.h> 00023 #include <stdbool.h> 00024 #include "device.h" 00025 00026 /** 00027 * Legacy format representing a timestamp in us. 00028 * Given it is modeled as a 32 bit integer, this type can represent timestamp 00029 * up to 4294 seconds (71 minutes). 00030 * Prefer using us_timestamp_t which store timestamp as 64 bits integer. 00031 */ 00032 typedef uint32_t timestamp_t; 00033 00034 /** 00035 * A us timestamp stored in a 64 bit integer. 00036 * Can store timestamp up to 584810 years. 00037 */ 00038 typedef uint64_t us_timestamp_t; 00039 00040 /** Ticker's event structure 00041 */ 00042 typedef struct ticker_event_s { 00043 us_timestamp_t timestamp; /**< Event's timestamp */ 00044 uint32_t id; /**< TimerEvent object */ 00045 struct ticker_event_s *next; /**< Next event in the queue */ 00046 } ticker_event_t; 00047 00048 typedef void (*ticker_event_handler)(uint32_t id); 00049 00050 /** Information about the ticker implementation 00051 */ 00052 typedef struct { 00053 uint32_t frequency; /**< Frequency in Hz this ticker runs at */ 00054 uint32_t bits; /**< Number of bits this ticker supports */ 00055 } ticker_info_t; 00056 00057 00058 /** Ticker's interface structure - required API for a ticker 00059 */ 00060 typedef struct { 00061 void (*init)(void); /**< Init function */ 00062 uint32_t (*read)(void); /**< Read function */ 00063 void (*disable_interrupt)(void); /**< Disable interrupt function */ 00064 void (*clear_interrupt)(void); /**< Clear interrupt function */ 00065 void (*set_interrupt)(timestamp_t timestamp); /**< Set interrupt function */ 00066 void (*fire_interrupt)(void); /**< Fire interrupt right-away */ 00067 const ticker_info_t *(*get_info)(void); /**< Return info about this ticker's implementation */ 00068 } ticker_interface_t; 00069 00070 /** Ticker's event queue structure 00071 */ 00072 typedef struct { 00073 ticker_event_handler event_handler; /**< Event handler */ 00074 ticker_event_t *head; /**< A pointer to head */ 00075 uint32_t frequency; /**< Frequency of the timer in Hz */ 00076 uint32_t bitmask; /**< Mask to be applied to time values read */ 00077 uint32_t max_delta; /**< Largest delta in ticks that can be used when scheduling */ 00078 uint64_t max_delta_us; /**< Largest delta in us that can be used when scheduling */ 00079 uint32_t tick_last_read; /**< Last tick read */ 00080 uint64_t tick_remainder; /**< Ticks that have not been added to base_time */ 00081 us_timestamp_t present_time; /**< Store the timestamp used for present time */ 00082 bool initialized; /**< Indicate if the instance is initialized */ 00083 } ticker_event_queue_t; 00084 00085 /** Ticker's data structure 00086 */ 00087 typedef struct { 00088 const ticker_interface_t *interface; /**< Ticker's interface */ 00089 ticker_event_queue_t *queue; /**< Ticker's event queue */ 00090 } ticker_data_t; 00091 00092 #ifdef __cplusplus 00093 extern "C" { 00094 #endif 00095 00096 /** 00097 * \defgroup hal_ticker Ticker HAL functions 00098 * @{ 00099 */ 00100 00101 /** Initialize a ticker and set the event handler 00102 * 00103 * @param ticker The ticker object. 00104 * @param handler A handler to be set 00105 */ 00106 void ticker_set_handler(const ticker_data_t *const ticker, ticker_event_handler handler); 00107 00108 /** IRQ handler that goes through the events to trigger overdue events. 00109 * 00110 * @param ticker The ticker object. 00111 */ 00112 void ticker_irq_handler(const ticker_data_t *const ticker); 00113 00114 /** Remove an event from the queue 00115 * 00116 * @param ticker The ticker object. 00117 * @param obj The event object to be removed from the queue 00118 */ 00119 void ticker_remove_event(const ticker_data_t *const ticker, ticker_event_t *obj); 00120 00121 /** Insert an event to the queue 00122 * 00123 * The event will be executed in timestamp - ticker_read(). 00124 * 00125 * @warning This function does not consider timestamp in the past. If an event 00126 * is inserted with a timestamp less than the current timestamp then the event 00127 * will be executed in timestamp - ticker_read() us. 00128 * The internal counter wrap very quickly it is hard to decide weither an 00129 * event is in the past or in 1 hour. 00130 * 00131 * @note prefer the use of ticker_insert_event_us which allows registration of 00132 * absolute timestamp. 00133 * 00134 * @param ticker The ticker object. 00135 * @param obj The event object to be inserted to the queue 00136 * @param timestamp The event's timestamp 00137 * @param id The event object 00138 */ 00139 void ticker_insert_event(const ticker_data_t *const ticker, ticker_event_t *obj, timestamp_t timestamp, uint32_t id); 00140 00141 /** Insert an event to the queue 00142 * 00143 * The event will be executed in timestamp - ticker_read_us() us. 00144 * 00145 * @note If an event is inserted with a timestamp less than the current 00146 * timestamp then the event will be scheduled immediately resulting in 00147 * an instant call to event handler. 00148 * 00149 * @param ticker The ticker object. 00150 * @param obj The event object to be inserted to the queue 00151 * @param timestamp The event's timestamp 00152 * @param id The event object 00153 */ 00154 void ticker_insert_event_us(const ticker_data_t *const ticker, ticker_event_t *obj, us_timestamp_t timestamp, uint32_t id); 00155 00156 /** Read the current (relative) ticker's timestamp 00157 * 00158 * @warning Return a relative timestamp because the counter wrap every 4294 00159 * seconds. 00160 * 00161 * @param ticker The ticker object. 00162 * @return The current timestamp 00163 */ 00164 timestamp_t ticker_read(const ticker_data_t *const ticker); 00165 00166 /** Read the current (absolute) ticker's timestamp 00167 * 00168 * @warning Return an absolute timestamp counting from the initialization of the 00169 * ticker. 00170 * 00171 * @param ticker The ticker object. 00172 * @return The current timestamp 00173 */ 00174 us_timestamp_t ticker_read_us(const ticker_data_t *const ticker); 00175 00176 /** Read the next event's timestamp 00177 * 00178 * @param ticker The ticker object. 00179 * @param timestamp The timestamp object. 00180 * @return 1 if timestamp is pending event, 0 if there's no event pending 00181 */ 00182 int ticker_get_next_timestamp(const ticker_data_t *const ticker, timestamp_t *timestamp); 00183 00184 /* Private functions 00185 * 00186 * @cond PRIVATE 00187 * 00188 */ 00189 00190 int _ticker_match_interval_passed(timestamp_t prev_tick, timestamp_t cur_tick, timestamp_t match_tick); 00191 00192 /* 00193 * @endcond PRIVATE 00194 * 00195 */ 00196 00197 /**@}*/ 00198 00199 #ifdef __cplusplus 00200 } 00201 #endif 00202 00203 #endif 00204 00205 /** @}*/
Generated on Sun Jul 17 2022 08:25:32 by
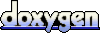