
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_net_config_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_net_config_api.h 00017 * \brief Public API to handle the Thread network services and configuration. 00018 */ 00019 00020 #ifndef _THREAD_NET_CONFIG_API_H_ 00021 #define _THREAD_NET_CONFIG_API_H_ 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 #include "ns_types.h" 00028 00029 /** 00030 * \brief function callback type for nd_data_request. 00031 * 00032 * \param inteface_id Network interface ID where request was made. 00033 * \param status 0 when response is received from destination, -1 otherwise. 00034 * \param data_ptr ND_data options encoded according to RFC6106. Is NULL if destination was unreachable or didn't have the requested data. 00035 * \param data_len Length of data in bytes. 00036 */ 00037 typedef void thread_net_config_nd_data_req_cb(int8_t interface_id, int8_t status, uint8_t *data_ptr, uint16_t data_len); 00038 00039 /** 00040 * \brief Request ND options (as in RFC6106) from given destination. 00041 * Response data will be provided in callback function. 00042 * 00043 * \param interface_id network interface ID. 00044 * \param destination IPv6 address where request is sent. 00045 * \param options requested option type identifiers according to RFC6106. 00046 * \param options_len number of options requested. 00047 * \param callback Function that will be called once information is available. 00048 * 00049 * \return 0 on success. A callback will be called with/without response data. 00050 * \return <0 in error cases. Callback will not be called. 00051 */ 00052 int thread_net_config_nd_data_request(int8_t interface_id, const uint8_t destination[16], const uint8_t *options, uint8_t options_len, thread_net_config_nd_data_req_cb *callback); 00053 00054 #ifdef __cplusplus 00055 } 00056 #endif 00057 #endif /* _THREAD_NET_CONFIG_API_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
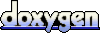