
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
thread_lowpower_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file thread_lowpower_api.h 00017 * \brief Nanostack Thread lowpower probe implementation. Responsible for tasks like 00018 * request link metrics by sending data requests. 00019 */ 00020 00021 #ifndef THREAD_LOWPOWER_API_H_ 00022 #define THREAD_LOWPOWER_API_H_ 00023 00024 #include <ns_types.h> 00025 00026 /** \brief callback function that returns the received metrics 00027 * 00028 * \param destination_address address to which the lowpower query is sent 00029 * \param interface_id interface_id of destination 00030 * \param metrics_ptr Pointer to metrics 00031 * \param metrics_len Length of metrics_ptr 00032 * 00033 * \return metrics_ptr if response received, NULL if no response is received 00034 * \return metrics_len length of the response received, 0 if NULL response is received 00035 * 00036 */ 00037 typedef int (thread_lowpower_resp_cb)(uint8_t *destination_address, int8_t interface_id, uint8_t *metrics_ptr, uint16_t metrics_len); 00038 00039 /** \brief Send lowpower data request for requesting low power metrics 00040 * 00041 * \param interface_id Interface ID of the Thread network. 00042 * \param destination_address destination ll64 address 00043 * \param metrics_ptr array of metrics to be measured 00044 * \param metrics_len length of the metrics array 00045 * \param response_cb callback function called to return values after execution 00046 * 00047 * \return 0 if data request successfully initiated. 00048 * 00049 */ 00050 int thread_lowpower_test_probe_send(int8_t interface_id, uint8_t *destination_address, uint8_t *metrics_ptr, uint8_t metrics_len, thread_lowpower_resp_cb response_cb); 00051 00052 #endif /*THREAD_LOWPOWER_API_H_ */
Generated on Sun Jul 17 2022 08:25:32 by
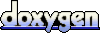