
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
test_db.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2014 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 00017 Author: Przemyslaw Wirkus <Przemyslaw.Wirkus@arm.com> 00018 """ 00019 00020 import re 00021 import json 00022 00023 00024 class BaseDBAccess (): 00025 """ Class used to connect with test database and store test results 00026 """ 00027 def __init__(self): 00028 self.db_object = None 00029 self.db_type = None 00030 # Connection credentials 00031 self.host = None 00032 self.user = None 00033 self.passwd = None 00034 self.db = None 00035 00036 # Test Suite DB scheme (table names) 00037 self.TABLE_BUILD_ID = 'mtest_build_id' 00038 self.TABLE_BUILD_ID_STATUS = 'mtest_build_id_status' 00039 self.TABLE_BUILD_ID_TYPE = 'mtest_build_id_type' 00040 self.TABLE_TARGET = 'mtest_target' 00041 self.TABLE_TEST_ENTRY = 'mtest_test_entry' 00042 self.TABLE_TEST_ID = 'mtest_test_id' 00043 self.TABLE_TEST_RESULT = 'mtest_test_result' 00044 self.TABLE_TEST_TYPE = 'mtest_test_type' 00045 self.TABLE_TOOLCHAIN = 'mtest_toolchain' 00046 # Build ID status PKs 00047 self.BUILD_ID_STATUS_STARTED = 1 # Started 00048 self.BUILD_ID_STATUS_IN_PROGRESS = 2 # In Progress 00049 self.BUILD_ID_STATUS_COMPLETED = 3 #Completed 00050 self.BUILD_ID_STATUS_FAILED = 4 # Failed 00051 # Build ID type PKs 00052 self.BUILD_ID_TYPE_TEST = 1 # Test 00053 self.BUILD_ID_TYPE_BUILD_ONLY = 2 # Build Only 00054 00055 def get_hostname (self): 00056 """ Useful when creating build_id in database 00057 Function returns (hostname, uname) which can be used as (build_id_name, build_id_desc) 00058 """ 00059 # Get hostname from socket 00060 import socket 00061 hostname = socket.gethostbyaddr(socket.gethostname())[0] 00062 # Get uname from platform resources 00063 import platform 00064 uname = json.dumps(platform.uname()) 00065 return (hostname, uname) 00066 00067 def get_db_type (self): 00068 """ Returns database type. E.g. 'mysql', 'sqlLite' etc. 00069 """ 00070 return self.db_type 00071 00072 def detect_database (self, verbose=False): 00073 """ detect database and return VERION data structure or string (verbose=True) 00074 """ 00075 return None 00076 00077 def parse_db_connection_string (self, str): 00078 """ Parsing SQL DB connection string. String should contain: 00079 - DB Name, user name, password, URL (DB host), name 00080 Function should return tuple with parsed (db_type, username, password, host, db_name) or None if error 00081 00082 (db_type, username, password, host, db_name) = self.parse_db_connection_string(db_url) 00083 00084 E.g. connection string: 'mysql://username:password@127.0.0.1/db_name' 00085 """ 00086 result = None 00087 if type(str) == type(''): 00088 PATTERN = '^([\w]+)://([\w]+):([\w]*)@(.*)/([\w]+)' 00089 result = re.match(PATTERN, str) 00090 if result is not None: 00091 result = result.groups() # Tuple (db_name, host, user, passwd, db) 00092 return result # (db_type, username, password, host, db_name) 00093 00094 def is_connected (self): 00095 """ Returns True if we are connected to database 00096 """ 00097 pass 00098 00099 def connect (self, host, user, passwd, db): 00100 """ Connects to DB and returns DB object 00101 """ 00102 pass 00103 00104 def connect_url (self, db_url): 00105 """ Connects to database using db_url (database url parsing), 00106 store host, username, password, db_name 00107 """ 00108 pass 00109 00110 def reconnect (self): 00111 """ Reconnects to DB and returns DB object using stored host name, 00112 database name and credentials (user name and password) 00113 """ 00114 pass 00115 00116 def disconnect (self): 00117 """ Close DB connection 00118 """ 00119 pass 00120 00121 def escape_string (self, str): 00122 """ Escapes string so it can be put in SQL query between quotes 00123 """ 00124 pass 00125 00126 def select_all (self, query): 00127 """ Execute SELECT query and get all results 00128 """ 00129 pass 00130 00131 def insert (self, query, commit=True): 00132 """ Execute INSERT query, define if you want to commit 00133 """ 00134 pass 00135 00136 def get_next_build_id (self, name, desc='', location='', type=None, status=None): 00137 """ Insert new build_id (DB unique build like ID number to send all test results) 00138 """ 00139 pass 00140 00141 def get_table_entry_pk (self, table, column, value, update_db=True): 00142 """ Checks for entries in tables with two columns (<TABLE_NAME>_pk, <column>) 00143 If update_db is True updates table entry if value in specified column doesn't exist 00144 """ 00145 pass 00146 00147 def update_table_entry (self, table, column, value): 00148 """ Updates table entry if value in specified column doesn't exist 00149 Locks table to perform atomic read + update 00150 """ 00151 pass 00152 00153 def update_build_id_info (self, build_id, **kw): 00154 """ Update additional data inside build_id table 00155 Examples: 00156 db.update_build_is(build_id, _status_fk=self.BUILD_ID_STATUS_COMPLETED, _shuffle_seed=0.0123456789): 00157 """ 00158 pass 00159 00160 def insert_test_entry (self, build_id, target, toolchain, test_type, test_id, test_result, test_time, test_timeout, test_loop, test_extra=''): 00161 """ Inserts test result entry to database. All checks regarding existing 00162 toolchain names in DB are performed. 00163 If some data is missing DB will be updated 00164 """ 00165 pass
Generated on Sun Jul 17 2022 08:25:32 by
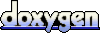