
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sw_mac.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file sw_mac.h 00016 * \brief Software MAC API. 00017 */ 00018 00019 #ifndef NS_SW_MAC_H 00020 #define NS_SW_MAC_H 00021 00022 #include "ns_types.h" 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 struct protocol_interface_rf_mac_setup; 00029 struct mac_api_s; 00030 struct mac_description_storage_size_s; 00031 struct fhss_api; 00032 00033 /** 00034 * @brief Creates 802.15.4 MAC API instance which will use RF driver given 00035 * @param rf_driver_id RF driver id. Must be valid 00036 * @param storage_sizes dynamic mac storage sizes DO NOT set any values to zero !! 00037 * @return New MAC instance if successful, NULL otherwise 00038 */ 00039 extern struct mac_api_s *ns_sw_mac_create(int8_t rf_driver_id, struct mac_description_storage_size_s *storage_sizes); 00040 00041 /** 00042 * @brief ns_sw_mac_virtual_client_register registers virtual driver to be used with 802.15.4 MAC. 00043 * This is always used with serial_mac_api 00044 * @param api API to start using virtual driver 00045 * @param virtual_driver_id 00046 * @return 0 if success, -1 if api or driver is invalid 00047 */ 00048 extern int8_t ns_sw_mac_virtual_client_register(struct mac_api_s *api, int8_t virtual_driver_id); 00049 00050 /** 00051 * @brief ns_sw_mac_virtual_client_unregister Unregisters virtual driver from 802.15.4 MAC 00052 * @param api API from which to unregister virtual driver 00053 * @return 0 if success, -1 if api is invalid 00054 */ 00055 extern int8_t ns_sw_mac_virtual_client_unregister(struct mac_api_s *api); 00056 00057 /** 00058 * @brief Registers created FHSS API instance to given software MAC instance. 00059 * @param mac_api MAC instance. 00060 * @param fhss_api FHSS instance. 00061 * @return 0 on success, -1 on fail. 00062 */ 00063 extern int ns_sw_mac_fhss_register(struct mac_api_s *mac_api, struct fhss_api *fhss_api); 00064 00065 00066 #ifdef __cplusplus 00067 } 00068 #endif 00069 00070 #endif // NS_SW_MAC_H
Generated on Sun Jul 17 2022 08:25:31 by
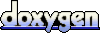