
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
svc_exports.h
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #ifndef __UVISOR_API_SVC_EXPORTS_H__ 00018 #define __UVISOR_API_SVC_EXPORTS_H__ 00019 00020 #include "api/inc/uvisor_exports.h" 00021 #include "api/inc/api.h" 00022 #include <stdint.h> 00023 00024 /* An SVCall takes a 8bit immediate, which is used as follows: 00025 * 00026 * For fast APIs: 00027 * 00028 * 7 6 5 4 3 2 1 0 00029 * .---.---.---.---.---.---.---.---. 00030 * | 1 | N | N | N | h | h | h | h | 00031 * '---'---'---'---'---'---'---'---' 00032 * | |_______| |___________| 00033 * | | | 00034 * | | | 00035 * | | | 00036 * | | (h) Handler index in the hardcoded table (max 16) 00037 * | | 00038 * | (N) Number of arguments to pass to the handler (max 4) 00039 * | 00040 * SVCall mode: Fast 00041 * 00042 * For slow APIs: 00043 * 00044 * 7 6 5 4 3 2 1 0 00045 * .---.---.---.---.---.---.---.---. 00046 * | 0 | c | c | c | c | c | c | c | 00047 * '---'---'---'---'---'---'---'---' 00048 * | |_______________________| 00049 * | | 00050 * | | 00051 * | (c) Handler index in the custom table (max 128) 00052 * | 00053 * SVCall mode: Slow 00054 */ 00055 00056 /* 1 bit of the SVCall imm8 field is used to determine the mode, fast/slow. */ 00057 #define UVISOR_SVC_MODE_FAST 1 00058 #define UVISOR_SVC_MODE_SLOW 0 00059 #define UVISOR_SVC_MODE_BIT 7 00060 #define UVISOR_SVC_MODE_MASK ((uint8_t) (1 << UVISOR_SVC_MODE_BIT)) 00061 #define UVISOR_SVC_MODE(mode) ((uint8_t) (((mode) << UVISOR_SVC_MODE_BIT) & UVISOR_SVC_MODE_MASK)) 00062 00063 /* 7 or 4 bits of the SVCall imm8 field are used to determine the table index, 00064 * depending on the mode, fast/slow. */ 00065 #define UVISOR_SVC_FAST_INDEX_MAX (1 << 4) 00066 #define UVISOR_SVC_SLOW_INDEX_MAX (1 << 7) 00067 #define UVISOR_SVC_FAST_INDEX_BIT 0 00068 #define UVISOR_SVC_FAST_INDEX_MASK ((uint8_t) (0xF << UVISOR_SVC_FAST_INDEX_BIT)) 00069 #define UVISOR_SVC_SLOW_INDEX_BIT 0 00070 #define UVISOR_SVC_SLOW_INDEX_MASK ((uint8_t) (0x7F << UVISOR_SVC_SLOW_INDEX_BIT)) 00071 #define UVISOR_SVC_FAST_INDEX(index) ((uint8_t) (((index) << UVISOR_SVC_FAST_INDEX_BIT) & UVISOR_SVC_FAST_INDEX_MASK)) 00072 #define UVISOR_SVC_SLOW_INDEX(index) ((uint8_t) (((index) << UVISOR_SVC_SLOW_INDEX_BIT) & UVISOR_SVC_SLOW_INDEX_MASK)) 00073 00074 /* When the SVC mode is "fast", the imm8 field also contains a specification of 00075 * the call interface (number of arguments). 00076 * This is needed for context switches, since the stack manipulation routines 00077 * need to know how many arguments to copy from source to destination. */ 00078 #define UVISOR_SVC_FAST_NARGS_BIT 4 00079 #define UVISOR_SVC_FAST_NARGS_MASK ((uint8_t) (0x7 << UVISOR_SVC_FAST_NARGS_BIT)) 00080 #define UVISOR_SVC_FAST_NARGS_SET(nargs) ((uint8_t) (((nargs) << UVISOR_SVC_FAST_NARGS_BIT) & UVISOR_SVC_FAST_NARGS_MASK)) 00081 #define UVISOR_SVC_FAST_NARGS_GET(svc_id) (((uint8_t) (svc_id) & UVISOR_SVC_FAST_NARGS_MASK) >> UVISOR_SVC_FAST_NARGS_BIT) 00082 00083 /* Macros to build the SVCall imm8 field. 00084 * For slow APIs only the SVC handler index is needed. 00085 * For fast APIs the SVC handler index and the number of arguments are needed. */ 00086 #define UVISOR_SVC_CUSTOM_TABLE(index) ((uint8_t) (UVISOR_SVC_MODE(UVISOR_SVC_MODE_SLOW) | \ 00087 UVISOR_SVC_SLOW_INDEX(index))) 00088 #define UVISOR_SVC_FIXED_TABLE(index, nargs) ((uint8_t) (UVISOR_SVC_MODE(UVISOR_SVC_MODE_FAST) | \ 00089 UVISOR_SVC_FAST_INDEX(index) | \ 00090 UVISOR_SVC_FAST_NARGS_SET(nargs))) 00091 00092 /* SVC immediate values for hardcoded table (call from unprivileged) */ 00093 #define UVISOR_SVC_ID_UNVIC_OUT UVISOR_SVC_FIXED_TABLE(0, 0) 00094 /* Deprecated: UVISOR_SVC_ID_CX_IN(nargs) UVISOR_SVC_FIXED_TABLE(1, nargs) */ 00095 /* Deprecated: UVISOR_SVC_ID_CX_OUT UVISOR_SVC_FIXED_TABLE(2, 0) */ 00096 #define UVISOR_SVC_ID_REGISTER_GATEWAY UVISOR_SVC_FIXED_TABLE(3, 0) 00097 #define UVISOR_SVC_ID_BOX_INIT_FIRST UVISOR_SVC_FIXED_TABLE(4, 0) 00098 #define UVISOR_SVC_ID_BOX_INIT_NEXT UVISOR_SVC_FIXED_TABLE(5, 0) 00099 00100 /* SVC immediate values for hardcoded table (call from privileged) */ 00101 #define UVISOR_SVC_ID_UNVIC_IN UVISOR_SVC_FIXED_TABLE(0, 0) 00102 00103 #endif /* __UVISOR_API_SVC_EXPORTS_H__ */
Generated on Sun Jul 17 2022 08:25:31 by
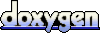