
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
stdio_auto.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 import re 00019 import random 00020 from time import time 00021 00022 class StdioTest(): 00023 PATTERN_INT_VALUE = "Your value was: (-?\d+)" 00024 re_detect_int_value = re.compile(PATTERN_INT_VALUE) 00025 00026 def test(self, selftest): 00027 test_result = True 00028 00029 c = selftest.mbed.serial_readline() # {{start}} preamble 00030 if c is None: 00031 return selftest.RESULT_IO_SERIAL 00032 selftest.notify(c) 00033 00034 for i in range(0, 10): 00035 random_integer = random.randint(-99999, 99999) 00036 selftest.notify("HOST: Generated number: " + str(random_integer)) 00037 start = time() 00038 selftest.mbed.serial_write(str(random_integer) + "\n") 00039 00040 serial_stdio_msg = selftest.mbed.serial_readline() 00041 if serial_stdio_msg is None: 00042 return selftest.RESULT_IO_SERIAL 00043 delay_time = time() - start 00044 selftest.notify(serial_stdio_msg.strip()) 00045 00046 # Searching for reply with scanned values 00047 m = self.re_detect_int_value.search(serial_stdio_msg) 00048 if m and len(m.groups()): 00049 int_value = m.groups()[0] 00050 int_value_cmp = random_integer == int(int_value) 00051 test_result = test_result and int_value_cmp 00052 selftest.notify("HOST: Number %s read after %.3f sec ... [%s]"% (int_value, delay_time, "OK" if int_value_cmp else "FAIL")) 00053 else: 00054 test_result = False 00055 break 00056 return selftest.RESULT_SUCCESS if test_result else selftest.RESULT_FAILURE
Generated on Sun Jul 17 2022 08:25:31 by
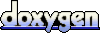