
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
sio.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 */ 00029 00030 /* 00031 * This is the interface to the platform specific serial IO module 00032 * It needs to be implemented by those platforms which need SLIP or PPP 00033 */ 00034 00035 #ifndef SIO_H 00036 #define SIO_H 00037 00038 #include "lwip/arch.h" 00039 #include "lwip/opt.h" 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* If you want to define sio_fd_t elsewhere or differently, 00046 define this in your cc.h file. */ 00047 #ifndef __sio_fd_t_defined 00048 typedef void * sio_fd_t; 00049 #endif 00050 00051 /* The following functions can be defined to something else in your cc.h file 00052 or be implemented in your custom sio.c file. */ 00053 00054 #ifndef sio_open 00055 /** 00056 * Opens a serial device for communication. 00057 * 00058 * @param devnum device number 00059 * @return handle to serial device if successful, NULL otherwise 00060 */ 00061 sio_fd_t sio_open(u8_t devnum); 00062 #endif 00063 00064 #ifndef sio_send 00065 /** 00066 * Sends a single character to the serial device. 00067 * 00068 * @param c character to send 00069 * @param fd serial device handle 00070 * 00071 * @note This function will block until the character can be sent. 00072 */ 00073 void sio_send(u8_t c, sio_fd_t fd); 00074 #endif 00075 00076 #ifndef sio_recv 00077 /** 00078 * Receives a single character from the serial device. 00079 * 00080 * @param fd serial device handle 00081 * 00082 * @note This function will block until a character is received. 00083 */ 00084 u8_t sio_recv(sio_fd_t fd); 00085 #endif 00086 00087 #ifndef sio_read 00088 /** 00089 * Reads from the serial device. 00090 * 00091 * @param fd serial device handle 00092 * @param data pointer to data buffer for receiving 00093 * @param len maximum length (in bytes) of data to receive 00094 * @return number of bytes actually received - may be 0 if aborted by sio_read_abort 00095 * 00096 * @note This function will block until data can be received. The blocking 00097 * can be cancelled by calling sio_read_abort(). 00098 */ 00099 u32_t sio_read(sio_fd_t fd, u8_t *data, u32_t len); 00100 #endif 00101 00102 #ifndef sio_tryread 00103 /** 00104 * Tries to read from the serial device. Same as sio_read but returns 00105 * immediately if no data is available and never blocks. 00106 * 00107 * @param fd serial device handle 00108 * @param data pointer to data buffer for receiving 00109 * @param len maximum length (in bytes) of data to receive 00110 * @return number of bytes actually received 00111 */ 00112 u32_t sio_tryread(sio_fd_t fd, u8_t *data, u32_t len); 00113 #endif 00114 00115 #ifndef sio_write 00116 /** 00117 * Writes to the serial device. 00118 * 00119 * @param fd serial device handle 00120 * @param data pointer to data to send 00121 * @param len length (in bytes) of data to send 00122 * @return number of bytes actually sent 00123 * 00124 * @note This function will block until all data can be sent. 00125 */ 00126 u32_t sio_write(sio_fd_t fd, u8_t *data, u32_t len); 00127 #endif 00128 00129 #ifndef sio_read_abort 00130 /** 00131 * Aborts a blocking sio_read() call. 00132 * 00133 * @param fd serial device handle 00134 */ 00135 void sio_read_abort(sio_fd_t fd); 00136 #endif 00137 00138 #ifdef __cplusplus 00139 } 00140 #endif 00141 00142 #endif /* SIO_H */
Generated on Sun Jul 17 2022 08:25:30 by
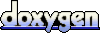