
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
settings.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2016 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 00018 from os import getenv 00019 from os.path import join, abspath, dirname, exists 00020 import logging 00021 00022 ROOT = abspath(join(dirname(__file__), "..")) 00023 00024 00025 ############################################################################## 00026 # Toolchains and Build System Settings 00027 ############################################################################## 00028 BUILD_DIR = abspath(join(ROOT, "BUILD")) 00029 00030 # ARM Compiler 5 00031 ARM_PATH = "" 00032 00033 # ARM Compiler 6 00034 ARMC6_PATH = "" 00035 00036 # GCC ARM 00037 GCC_ARM_PATH = "" 00038 00039 # GCC CodeRed 00040 GCC_CR_PATH = "" 00041 00042 # IAR 00043 IAR_PATH = "" 00044 00045 # Goanna static analyser. Please overload it in mbed_settings.py 00046 GOANNA_PATH = "" 00047 00048 # cppcheck path (command) and output message format 00049 CPPCHECK_CMD = ["cppcheck", "--enable=all"] 00050 CPPCHECK_MSG_FORMAT = ["--template=[{severity}] {file}@{line}: {id}:{message}"] 00051 00052 BUILD_OPTIONS = [] 00053 00054 # mbed.org username 00055 MBED_ORG_USER = "" 00056 00057 CLI_COLOR_MAP = { 00058 "warning": "yellow", 00059 "error" : "red" 00060 } 00061 00062 ############################################################################## 00063 # User Settings (file) 00064 ############################################################################## 00065 try: 00066 # Allow to overwrite the default settings without the need to edit the 00067 # settings file stored in the repository 00068 from mbed_settings import * 00069 except ImportError: 00070 pass 00071 00072 00073 ############################################################################## 00074 # User Settings (env vars) 00075 ############################################################################## 00076 _ENV_PATHS = ['ARM_PATH', 'GCC_ARM_PATH', 'GCC_CR_PATH', 'IAR_PATH', 00077 'ARMC6_PATH'] 00078 00079 for _n in _ENV_PATHS: 00080 if getenv('MBED_'+_n): 00081 if exists(getenv('MBED_'+_n)): 00082 globals()[_n] = getenv('MBED_'+_n) 00083 else: 00084 print "WARNING: MBED_%s set as environment variable but doesn't exist" % _n 00085 00086 00087 ############################################################################## 00088 # Test System Settings 00089 ############################################################################## 00090 SERVER_PORT = 59432 00091 SERVER_ADDRESS = "10.2.200.94" 00092 LOCALHOST = "10.2.200.94" 00093 00094 MUTs = { 00095 "1" : {"mcu": "LPC1768", 00096 "port":"COM41", "disk":'E:\\', 00097 "peripherals": ["TMP102", "digital_loop", "port_loop", "analog_loop", "SD"] 00098 }, 00099 "2": {"mcu": "LPC11U24", 00100 "port":"COM42", "disk":'F:\\', 00101 "peripherals": ["TMP102", "digital_loop", "port_loop", "SD"] 00102 }, 00103 "3" : {"mcu": "KL25Z", 00104 "port":"COM43", "disk":'G:\\', 00105 "peripherals": ["TMP102", "digital_loop", "port_loop", "analog_loop", "SD"] 00106 }, 00107 }
Generated on Sun Jul 17 2022 08:25:30 by
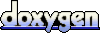