
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
serial_mac_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file serial_mac_api.h 00016 * \brief Serial MAC API. 00017 */ 00018 00019 #ifndef SERIAL_MAC_API_H_ 00020 #define SERIAL_MAC_API_H_ 00021 00022 #include <inttypes.h> 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 struct virtual_data_req_s; 00029 00030 typedef struct serial_mac_api_s serial_mac_api_t; 00031 00032 /** 00033 * Create serial MAC 00034 * @param serial_driver_id PHY driver ID. 00035 * @return Serial MAC callback structure on success. 00036 * @return NULL on failure. 00037 */ 00038 extern serial_mac_api_t *serial_mac_create(int8_t serial_driver_id); 00039 00040 /** 00041 * @brief data_request data request call 00042 * @param api API to handle the request 00043 * @param data Data to be sent 00044 * @param data_length Length of the data 00045 */ 00046 typedef int8_t data_request(const serial_mac_api_t* api, const uint8_t *data_ptr, uint16_t data_length); 00047 00048 00049 /** 00050 * @brief data_indication Data indication is called when MAC layer has received data 00051 * @param api The API which handled the response 00052 * @param data Data to be sent 00053 * @param data_length Length of the data 00054 */ 00055 typedef void data_indication(const serial_mac_api_t *api, const uint8_t *data_ptr, uint16_t data_length); 00056 00057 /** 00058 * * @brief serial_mac_api_initialize Initialises Serial MAC layer into use 00059 * @param api API to initialize 00060 * @param ind_cb Callback to call when data is received. 00061 * @return 0 if success, -1 if api is invalid 00062 */ 00063 typedef int8_t serial_mac_api_initialize(serial_mac_api_t *api, data_indication *ind_cb); 00064 00065 /** 00066 * @brief serial_mac_virtual_initialize Initialises MAC to use virtual driver 00067 * @param api API to start using virtual driver 00068 * @param driver_id Virtual driver ID. Must be valid. 00069 * @return 0 if success, -1 if api or driver is invalid 00070 */ 00071 typedef int8_t serial_mac_virtual_initialize(const serial_mac_api_t *api, int8_t driver_id); 00072 00073 /** 00074 * Serial MAC callback structure. 00075 */ 00076 struct serial_mac_api_s { 00077 serial_mac_api_initialize *mac_initialize; /**< Inititilize data callback */ 00078 serial_mac_virtual_initialize * virtual_initilize; /**< Enable bridge to virtual driver */ 00079 data_request *data_request_cb; /**< Data request callback */ 00080 data_indication *data_ind_cb; /**< Data indication callback */ 00081 }; 00082 00083 #ifdef __cplusplus 00084 } 00085 #endif 00086 00087 #endif /* SERIAL_MAC_API_H_ */
Generated on Sun Jul 17 2022 08:25:29 by
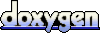