
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
randLIB_stub.c
00001 /* 00002 * Copyright (c) 2014-2017 ARM Limited. All Rights Reserved. 00003 */ 00004 #include <stdint.h> 00005 #include <stdlib.h> 00006 #include <stdbool.h> 00007 #include <limits.h> 00008 #include "randLIB.h" 00009 #include "platform/arm_hal_random.h" 00010 00011 #if ((RAND_MAX+1) & RAND_MAX) != 0 00012 #error "RAND_MAX isn't 2^n-1 :(" 00013 #endif 00014 00015 int counter = 1; 00016 00017 void randLIB_seed_random(void) 00018 { 00019 } 00020 00021 uint8_t randLIB_get_8bit(void) 00022 { 00023 return 0; 00024 } 00025 00026 uint16_t randLIB_get_16bit(void) 00027 { 00028 return 0; 00029 } 00030 00031 uint32_t randLIB_get_32bit(void) 00032 { 00033 return 0; 00034 } 00035 00036 void *randLIB_get_n_bytes_random(void *data_ptr, uint8_t count) 00037 { 00038 if(data_ptr && count > 0){ 00039 *((int*)data_ptr) = counter++%255; 00040 } 00041 return data_ptr; 00042 } 00043 00044 uint16_t randLIB_get_random_in_range(uint16_t min, uint16_t max) 00045 { 00046 return 0; 00047 } 00048 00049 uint32_t randLIB_randomise_base(uint32_t base, uint16_t min_factor, uint16_t max_factor) 00050 { 00051 return 0; 00052 }
Generated on Sun Jul 17 2022 08:25:29 by
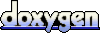