
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
pylint.py
00001 """A test that all code scores above a 9.25 in pylint""" 00002 00003 import subprocess 00004 import re 00005 import os.path 00006 00007 SCORE_REGEXP = re.compile( 00008 r'^Your\ code\ has\ been\ rated\ at\ (\-?[0-9\.]+)/10') 00009 00010 TOOLS_ROOT = os.path.dirname(os.path.dirname(__file__)) 00011 00012 00013 def parse_score (pylint_output): 00014 """Parse the score out of pylint's output as a float If the score is not 00015 found, return 0.0. 00016 """ 00017 for line in pylint_output.splitlines(): 00018 match = re.match(SCORE_REGEXP, line) 00019 if match: 00020 return float(match.group(1)) 00021 return 0.0 00022 00023 def execute_pylint (filename): 00024 """Execute a pylint process and collect it's output 00025 """ 00026 process = subprocess.Popen( 00027 ["pylint", filename], 00028 stdout=subprocess.PIPE, 00029 stderr=subprocess.PIPE 00030 ) 00031 stout, sterr = process.communicate() 00032 status = process.poll() 00033 return status, stout, sterr 00034 00035 FILES = ["build_api.py", "config.py", "colorize.py", "detect_targets.py", 00036 "hooks.py", "libraries.py", "memap.py", "options.py", "paths.py", 00037 "targets.py", "test/pylint.py"] 00038 00039 if __name__ == "__main__": 00040 for python_module in FILES: 00041 _, stdout, stderr = execute_pylint(os.path.join(TOOLS_ROOT, 00042 python_module)) 00043 score = parse_score(stdout) 00044 if score < 9.25: 00045 print(stdout) 00046 00047 00048
Generated on Sun Jul 17 2022 08:25:29 by
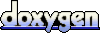