
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
nd6.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * ND6 protocol definitions 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 #ifndef LWIP_HDR_PROT_ND6_H 00038 #define LWIP_HDR_PROT_ND6_H 00039 00040 #include "lwip/arch.h" 00041 #include "lwip/ip6_addr.h" 00042 #include "lwip/prot/ip6.h" 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /** Neighbor solicitation message header. */ 00049 #ifdef PACK_STRUCT_USE_INCLUDES 00050 # include "arch/bpstruct.h" 00051 #endif 00052 PACK_STRUCT_BEGIN 00053 struct ns_header { 00054 PACK_STRUCT_FLD_8(u8_t type); 00055 PACK_STRUCT_FLD_8(u8_t code); 00056 PACK_STRUCT_FIELD(u16_t chksum); 00057 PACK_STRUCT_FIELD(u32_t reserved); 00058 PACK_STRUCT_FLD_S(ip6_addr_p_t target_address); 00059 /* Options follow. */ 00060 } PACK_STRUCT_STRUCT; 00061 PACK_STRUCT_END 00062 #ifdef PACK_STRUCT_USE_INCLUDES 00063 # include "arch/epstruct.h" 00064 #endif 00065 00066 /** Neighbor advertisement message header. */ 00067 #ifdef PACK_STRUCT_USE_INCLUDES 00068 # include "arch/bpstruct.h" 00069 #endif 00070 PACK_STRUCT_BEGIN 00071 struct na_header { 00072 PACK_STRUCT_FLD_8(u8_t type); 00073 PACK_STRUCT_FLD_8(u8_t code); 00074 PACK_STRUCT_FIELD(u16_t chksum); 00075 PACK_STRUCT_FLD_8(u8_t flags); 00076 PACK_STRUCT_FLD_8(u8_t reserved[3]); 00077 PACK_STRUCT_FLD_S(ip6_addr_p_t target_address); 00078 /* Options follow. */ 00079 } PACK_STRUCT_STRUCT; 00080 PACK_STRUCT_END 00081 #ifdef PACK_STRUCT_USE_INCLUDES 00082 # include "arch/epstruct.h" 00083 #endif 00084 #define ND6_FLAG_ROUTER (0x80) 00085 #define ND6_FLAG_SOLICITED (0x40) 00086 #define ND6_FLAG_OVERRIDE (0x20) 00087 00088 /** Router solicitation message header. */ 00089 #ifdef PACK_STRUCT_USE_INCLUDES 00090 # include "arch/bpstruct.h" 00091 #endif 00092 PACK_STRUCT_BEGIN 00093 struct rs_header { 00094 PACK_STRUCT_FLD_8(u8_t type); 00095 PACK_STRUCT_FLD_8(u8_t code); 00096 PACK_STRUCT_FIELD(u16_t chksum); 00097 PACK_STRUCT_FIELD(u32_t reserved); 00098 /* Options follow. */ 00099 } PACK_STRUCT_STRUCT; 00100 PACK_STRUCT_END 00101 #ifdef PACK_STRUCT_USE_INCLUDES 00102 # include "arch/epstruct.h" 00103 #endif 00104 00105 /** Router advertisement message header. */ 00106 #define ND6_RA_FLAG_MANAGED_ADDR_CONFIG (0x80) 00107 #define ND6_RA_FLAG_OTHER_CONFIG (0x40) 00108 #define ND6_RA_FLAG_HOME_AGENT (0x20) 00109 #define ND6_RA_PREFERENCE_MASK (0x18) 00110 #define ND6_RA_PREFERENCE_HIGH (0x08) 00111 #define ND6_RA_PREFERENCE_MEDIUM (0x00) 00112 #define ND6_RA_PREFERENCE_LOW (0x18) 00113 #define ND6_RA_PREFERENCE_DISABLED (0x10) 00114 #ifdef PACK_STRUCT_USE_INCLUDES 00115 # include "arch/bpstruct.h" 00116 #endif 00117 PACK_STRUCT_BEGIN 00118 struct ra_header { 00119 PACK_STRUCT_FLD_8(u8_t type); 00120 PACK_STRUCT_FLD_8(u8_t code); 00121 PACK_STRUCT_FIELD(u16_t chksum); 00122 PACK_STRUCT_FLD_8(u8_t current_hop_limit); 00123 PACK_STRUCT_FLD_8(u8_t flags); 00124 PACK_STRUCT_FIELD(u16_t router_lifetime); 00125 PACK_STRUCT_FIELD(u32_t reachable_time); 00126 PACK_STRUCT_FIELD(u32_t retrans_timer); 00127 /* Options follow. */ 00128 } PACK_STRUCT_STRUCT; 00129 PACK_STRUCT_END 00130 #ifdef PACK_STRUCT_USE_INCLUDES 00131 # include "arch/epstruct.h" 00132 #endif 00133 00134 /** Redirect message header. */ 00135 #ifdef PACK_STRUCT_USE_INCLUDES 00136 # include "arch/bpstruct.h" 00137 #endif 00138 PACK_STRUCT_BEGIN 00139 struct redirect_header { 00140 PACK_STRUCT_FLD_8(u8_t type); 00141 PACK_STRUCT_FLD_8(u8_t code); 00142 PACK_STRUCT_FIELD(u16_t chksum); 00143 PACK_STRUCT_FIELD(u32_t reserved); 00144 PACK_STRUCT_FLD_S(ip6_addr_p_t target_address); 00145 PACK_STRUCT_FLD_S(ip6_addr_p_t destination_address); 00146 /* Options follow. */ 00147 } PACK_STRUCT_STRUCT; 00148 PACK_STRUCT_END 00149 #ifdef PACK_STRUCT_USE_INCLUDES 00150 # include "arch/epstruct.h" 00151 #endif 00152 00153 /** Link-layer address option. */ 00154 #define ND6_OPTION_TYPE_SOURCE_LLADDR (0x01) 00155 #define ND6_OPTION_TYPE_TARGET_LLADDR (0x02) 00156 #ifdef PACK_STRUCT_USE_INCLUDES 00157 # include "arch/bpstruct.h" 00158 #endif 00159 PACK_STRUCT_BEGIN 00160 struct lladdr_option { 00161 PACK_STRUCT_FLD_8(u8_t type); 00162 PACK_STRUCT_FLD_8(u8_t length); 00163 PACK_STRUCT_FLD_8(u8_t addr[NETIF_MAX_HWADDR_LEN]); 00164 } PACK_STRUCT_STRUCT; 00165 PACK_STRUCT_END 00166 #ifdef PACK_STRUCT_USE_INCLUDES 00167 # include "arch/epstruct.h" 00168 #endif 00169 00170 /** Prefix information option. */ 00171 #define ND6_OPTION_TYPE_PREFIX_INFO (0x03) 00172 #define ND6_PREFIX_FLAG_ON_LINK (0x80) 00173 #define ND6_PREFIX_FLAG_AUTONOMOUS (0x40) 00174 #define ND6_PREFIX_FLAG_ROUTER_ADDRESS (0x20) 00175 #define ND6_PREFIX_FLAG_SITE_PREFIX (0x10) 00176 #ifdef PACK_STRUCT_USE_INCLUDES 00177 # include "arch/bpstruct.h" 00178 #endif 00179 PACK_STRUCT_BEGIN 00180 struct prefix_option { 00181 PACK_STRUCT_FLD_8(u8_t type); 00182 PACK_STRUCT_FLD_8(u8_t length); 00183 PACK_STRUCT_FLD_8(u8_t prefix_length); 00184 PACK_STRUCT_FLD_8(u8_t flags); 00185 PACK_STRUCT_FIELD(u32_t valid_lifetime); 00186 PACK_STRUCT_FIELD(u32_t preferred_lifetime); 00187 PACK_STRUCT_FLD_8(u8_t reserved2[3]); 00188 PACK_STRUCT_FLD_8(u8_t site_prefix_length); 00189 PACK_STRUCT_FLD_S(ip6_addr_p_t prefix); 00190 } PACK_STRUCT_STRUCT; 00191 PACK_STRUCT_END 00192 #ifdef PACK_STRUCT_USE_INCLUDES 00193 # include "arch/epstruct.h" 00194 #endif 00195 00196 /** Redirected header option. */ 00197 #define ND6_OPTION_TYPE_REDIR_HDR (0x04) 00198 #ifdef PACK_STRUCT_USE_INCLUDES 00199 # include "arch/bpstruct.h" 00200 #endif 00201 PACK_STRUCT_BEGIN 00202 struct redirected_header_option { 00203 PACK_STRUCT_FLD_8(u8_t type); 00204 PACK_STRUCT_FLD_8(u8_t length); 00205 PACK_STRUCT_FLD_8(u8_t reserved[6]); 00206 /* Portion of redirected packet follows. */ 00207 /* PACK_STRUCT_FLD_8(u8_t redirected[8]); */ 00208 } PACK_STRUCT_STRUCT; 00209 PACK_STRUCT_END 00210 #ifdef PACK_STRUCT_USE_INCLUDES 00211 # include "arch/epstruct.h" 00212 #endif 00213 00214 /** MTU option. */ 00215 #define ND6_OPTION_TYPE_MTU (0x05) 00216 #ifdef PACK_STRUCT_USE_INCLUDES 00217 # include "arch/bpstruct.h" 00218 #endif 00219 PACK_STRUCT_BEGIN 00220 struct mtu_option { 00221 PACK_STRUCT_FLD_8(u8_t type); 00222 PACK_STRUCT_FLD_8(u8_t length); 00223 PACK_STRUCT_FIELD(u16_t reserved); 00224 PACK_STRUCT_FIELD(u32_t mtu); 00225 } PACK_STRUCT_STRUCT; 00226 PACK_STRUCT_END 00227 #ifdef PACK_STRUCT_USE_INCLUDES 00228 # include "arch/epstruct.h" 00229 #endif 00230 00231 /** Route information option. */ 00232 #define ND6_OPTION_TYPE_ROUTE_INFO (24) 00233 #ifdef PACK_STRUCT_USE_INCLUDES 00234 # include "arch/bpstruct.h" 00235 #endif 00236 PACK_STRUCT_BEGIN 00237 struct route_option { 00238 PACK_STRUCT_FLD_8(u8_t type); 00239 PACK_STRUCT_FLD_8(u8_t length); 00240 PACK_STRUCT_FLD_8(u8_t prefix_length); 00241 PACK_STRUCT_FLD_8(u8_t preference); 00242 PACK_STRUCT_FIELD(u32_t route_lifetime); 00243 PACK_STRUCT_FLD_S(ip6_addr_p_t prefix); 00244 } PACK_STRUCT_STRUCT; 00245 PACK_STRUCT_END 00246 #ifdef PACK_STRUCT_USE_INCLUDES 00247 # include "arch/epstruct.h" 00248 #endif 00249 00250 /** Recursive DNS Server Option. */ 00251 #if LWIP_ND6_RDNSS_MAX_DNS_SERVERS 00252 #define LWIP_RDNSS_OPTION_MAX_SERVERS LWIP_ND6_RDNSS_MAX_DNS_SERVERS 00253 #else 00254 #define LWIP_RDNSS_OPTION_MAX_SERVERS 1 00255 #endif 00256 #define ND6_OPTION_TYPE_RDNSS (25) 00257 #ifdef PACK_STRUCT_USE_INCLUDES 00258 # include "arch/bpstruct.h" 00259 #endif 00260 PACK_STRUCT_BEGIN 00261 struct rdnss_option { 00262 PACK_STRUCT_FLD_8(u8_t type); 00263 PACK_STRUCT_FLD_8(u8_t length); 00264 PACK_STRUCT_FIELD(u16_t reserved); 00265 PACK_STRUCT_FIELD(u32_t lifetime); 00266 PACK_STRUCT_FLD_S(ip6_addr_p_t rdnss_address[LWIP_RDNSS_OPTION_MAX_SERVERS]); 00267 } PACK_STRUCT_STRUCT; 00268 PACK_STRUCT_END 00269 #ifdef PACK_STRUCT_USE_INCLUDES 00270 # include "arch/epstruct.h" 00271 #endif 00272 00273 #ifdef __cplusplus 00274 } 00275 #endif 00276 00277 #endif /* LWIP_HDR_PROT_ND6_H */
Generated on Sun Jul 17 2022 08:25:28 by
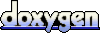