
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
project.py
00001 """ The CLI entry point for exporting projects from the mbed tools to any of the 00002 supported IDEs or project structures. 00003 """ 00004 import sys 00005 from os.path import join, abspath, dirname, exists, basename 00006 ROOT = abspath(join(dirname(__file__), "..")) 00007 sys.path.insert(0, ROOT) 00008 00009 from shutil import move, rmtree 00010 from argparse import ArgumentParser 00011 from os.path import normpath, realpath 00012 00013 from tools.paths import EXPORT_DIR, MBED_HAL, MBED_LIBRARIES, MBED_TARGETS_PATH 00014 from tools.settings import BUILD_DIR 00015 from tools.export import EXPORTERS, mcu_ide_matrix, mcu_ide_list, export_project, get_exporter_toolchain 00016 from tools.tests import TESTS, TEST_MAP 00017 from tools.tests import test_known, test_name_known, Test 00018 from tools.targets import TARGET_NAMES 00019 from tools.utils import argparse_filestring_type, argparse_profile_filestring_type, argparse_many, args_error 00020 from tools.utils import argparse_force_lowercase_type 00021 from tools.utils import argparse_force_uppercase_type 00022 from tools.utils import print_large_string 00023 from tools.utils import NotSupportedException 00024 from tools.options import extract_profile, list_profiles, extract_mcus 00025 00026 def setup_project (ide, target, program=None, source_dir=None, build=None, export_path=None): 00027 """Generate a name, if not provided, and find dependencies 00028 00029 Positional arguments: 00030 ide - IDE or project structure that will soon be exported to 00031 target - MCU that the project will build for 00032 00033 Keyword arguments: 00034 program - the index of a test program 00035 source_dir - the directory, or directories that contain all of the sources 00036 build - a directory that will contain the result of the export 00037 """ 00038 # Some libraries have extra macros (called by exporter symbols) to we need 00039 # to pass them to maintain compilation macros integrity between compiled 00040 # library and header files we might use with it 00041 if source_dir: 00042 # --source is used to generate IDE files to toolchain directly 00043 # in the source tree and doesn't generate zip file 00044 project_dir = export_path or source_dir[0] 00045 if program: 00046 project_name = TESTS[program] 00047 else: 00048 project_name = basename(normpath(realpath(source_dir[0]))) 00049 src_paths = source_dir 00050 lib_paths = None 00051 else: 00052 test = Test(program) 00053 if not build: 00054 # Substitute the mbed library builds with their sources 00055 if MBED_LIBRARIES in test.dependencies: 00056 test.dependencies.remove(MBED_LIBRARIES) 00057 test.dependencies.append(MBED_HAL) 00058 test.dependencies.append(MBED_TARGETS_PATH) 00059 00060 00061 src_paths = [test.source_dir] 00062 lib_paths = test.dependencies 00063 project_name = "_".join([test.id, ide, target]) 00064 project_dir = join(EXPORT_DIR, project_name) 00065 00066 return project_dir, project_name, src_paths, lib_paths 00067 00068 00069 def export (target, ide, build=None, src=None, macros=None, project_id=None, 00070 zip_proj=False, build_profile=None, export_path=None, silent=False, 00071 app_config=None): 00072 """Do an export of a project. 00073 00074 Positional arguments: 00075 target - MCU that the project will compile for 00076 ide - the IDE or project structure to export to 00077 00078 Keyword arguments: 00079 build - to use the compiled mbed libraries or not 00080 src - directory or directories that contain the source to export 00081 macros - extra macros to add to the project 00082 project_id - the name of the project 00083 clean - start from a clean state before exporting 00084 zip_proj - create a zip file or not 00085 00086 Returns an object of type Exporter (tools/exports/exporters.py) 00087 """ 00088 project_dir, name, src, lib = setup_project(ide, target, program=project_id, 00089 source_dir=src, build=build, export_path=export_path) 00090 00091 zip_name = name+".zip" if zip_proj else None 00092 00093 return export_project(src, project_dir, target, ide, name=name, 00094 macros=macros, libraries_paths=lib, zip_proj=zip_name, 00095 build_profile=build_profile, silent=silent, 00096 app_config=app_config) 00097 00098 00099 def main (): 00100 """Entry point""" 00101 # Parse Options 00102 parser = ArgumentParser() 00103 00104 targetnames = TARGET_NAMES 00105 targetnames.sort() 00106 toolchainlist = EXPORTERS.keys() 00107 toolchainlist.sort() 00108 00109 parser.add_argument("-m", "--mcu", 00110 metavar="MCU", 00111 type=str.upper, 00112 help="generate project for the given MCU ({})".format( 00113 ', '.join(targetnames))) 00114 00115 parser.add_argument("-i", 00116 dest="ide", 00117 type=argparse_force_lowercase_type( 00118 toolchainlist, "toolchain"), 00119 help="The target IDE: %s"% str(toolchainlist)) 00120 00121 parser.add_argument("-c", "--clean", 00122 action="store_true", 00123 default=False, 00124 help="clean the export directory") 00125 00126 group = parser.add_mutually_exclusive_group(required=False) 00127 group.add_argument( 00128 "-p", 00129 type=test_known, 00130 dest="program", 00131 help="The index of the desired test program: [0-%s]"% (len(TESTS)-1)) 00132 00133 group.add_argument("-n", 00134 type=test_name_known, 00135 dest="program", 00136 help="The name of the desired test program") 00137 00138 parser.add_argument("-b", 00139 dest="build", 00140 default=False, 00141 action="store_true", 00142 help="use the mbed library build, instead of the sources") 00143 00144 group.add_argument("-L", "--list-tests", 00145 action="store_true", 00146 dest="list_tests", 00147 default=False, 00148 help="list available programs in order and exit") 00149 00150 group.add_argument("-S", "--list-matrix", 00151 dest="supported_ides", 00152 default=False, 00153 const="matrix", 00154 choices=["matrix", "ides"], 00155 nargs="?", 00156 help="displays supported matrix of MCUs and IDEs") 00157 00158 parser.add_argument("-E", 00159 action="store_true", 00160 dest="supported_ides_html", 00161 default=False, 00162 help="writes tools/export/README.md") 00163 00164 parser.add_argument("--source", 00165 action="append", 00166 type=argparse_filestring_type, 00167 dest="source_dir", 00168 default=[], 00169 help="The source (input) directory") 00170 00171 parser.add_argument("-D", 00172 action="append", 00173 dest="macros", 00174 help="Add a macro definition") 00175 00176 parser.add_argument("--profile", dest="profile", action="append", 00177 type=argparse_profile_filestring_type, 00178 help="Build profile to use. Can be either path to json" \ 00179 "file or one of the default one ({})".format(", ".join(list_profiles())), 00180 default=[]) 00181 00182 parser.add_argument("--update-packs", 00183 dest="update_packs", 00184 action="store_true", 00185 default=False) 00186 parser.add_argument("--app-config", 00187 dest="app_config", 00188 default=None) 00189 00190 options = parser.parse_args() 00191 00192 # Print available tests in order and exit 00193 if options.list_tests is True: 00194 print '\n'.join([str(test) for test in sorted(TEST_MAP.values())]) 00195 sys.exit() 00196 00197 # Only prints matrix of supported IDEs 00198 if options.supported_ides: 00199 if options.supported_ides == "matrix": 00200 print_large_string(mcu_ide_matrix()) 00201 elif options.supported_ides == "ides": 00202 print mcu_ide_list() 00203 exit(0) 00204 00205 # Only prints matrix of supported IDEs 00206 if options.supported_ides_html: 00207 html = mcu_ide_matrix(verbose_html=True) 00208 try: 00209 with open("./export/README.md", "w") as readme: 00210 readme.write("Exporter IDE/Platform Support\n") 00211 readme.write("-----------------------------------\n") 00212 readme.write("\n") 00213 readme.write(html) 00214 except IOError as exc: 00215 print "I/O error({0}): {1}".format(exc.errno, exc.strerror) 00216 except: 00217 print "Unexpected error:", sys.exc_info()[0] 00218 raise 00219 exit(0) 00220 00221 if options.update_packs: 00222 from tools.arm_pack_manager import Cache 00223 cache = Cache(True, True) 00224 cache.cache_descriptors() 00225 00226 # Target 00227 if not options.mcu: 00228 args_error(parser, "argument -m/--mcu is required") 00229 00230 # Toolchain 00231 if not options.ide: 00232 args_error(parser, "argument -i is required") 00233 00234 # Clean Export Directory 00235 if options.clean: 00236 if exists(EXPORT_DIR): 00237 rmtree(EXPORT_DIR) 00238 00239 zip_proj = not bool(options.source_dir) 00240 00241 if (options.program is None) and (not options.source_dir): 00242 args_error(parser, "one of -p, -n, or --source is required") 00243 exporter, toolchain_name = get_exporter_toolchain(options.ide) 00244 mcu = extract_mcus(parser, options)[0] 00245 if not exporter.is_target_supported(mcu): 00246 args_error(parser, "%s not supported by %s"%(mcu,options.ide)) 00247 profile = extract_profile(parser, options, toolchain_name, fallback="debug") 00248 if options.clean: 00249 rmtree(BUILD_DIR) 00250 try: 00251 export(mcu, options.ide, build=options.build, 00252 src=options.source_dir, macros=options.macros, 00253 project_id=options.program, zip_proj=zip_proj, 00254 build_profile=profile, app_config=options.app_config) 00255 except NotSupportedException as exc: 00256 print "[ERROR] %s" % str(exc) 00257 00258 if __name__ == "__main__": 00259 main()
Generated on Sun Jul 17 2022 08:25:29 by
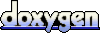