
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
pppcrypt.h
00001 /* 00002 * pppcrypt.c - PPP/DES linkage for MS-CHAP and EAP SRP-SHA1 00003 * 00004 * Extracted from chap_ms.c by James Carlson. 00005 * 00006 * Copyright (c) 1995 Eric Rosenquist. All rights reserved. 00007 * 00008 * Redistribution and use in source and binary forms, with or without 00009 * modification, are permitted provided that the following conditions 00010 * are met: 00011 * 00012 * 1. Redistributions of source code must retain the above copyright 00013 * notice, this list of conditions and the following disclaimer. 00014 * 00015 * 2. Redistributions in binary form must reproduce the above copyright 00016 * notice, this list of conditions and the following disclaimer in 00017 * the documentation and/or other materials provided with the 00018 * distribution. 00019 * 00020 * 3. The name(s) of the authors of this software must not be used to 00021 * endorse or promote products derived from this software without 00022 * prior written permission. 00023 * 00024 * THE AUTHORS OF THIS SOFTWARE DISCLAIM ALL WARRANTIES WITH REGARD TO 00025 * THIS SOFTWARE, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY 00026 * AND FITNESS, IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY 00027 * SPECIAL, INDIRECT OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES 00028 * WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN 00029 * AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING 00030 * OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE. 00031 */ 00032 00033 #include "netif/ppp/ppp_opts.h" 00034 #if PPP_SUPPORT /* don't build if not configured for use in lwipopts.h */ 00035 00036 /* This header file is included in all PPP modules needing hashes and/or ciphers */ 00037 00038 #ifndef PPPCRYPT_H 00039 #define PPPCRYPT_H 00040 00041 /* 00042 * If included PolarSSL copy is not used, user is expected to include 00043 * external libraries in arch/cc.h (which is included by lwip/arch.h). 00044 */ 00045 #include "lwip/arch.h" 00046 00047 /* 00048 * Map hashes and ciphers functions to PolarSSL 00049 */ 00050 #if !LWIP_USE_EXTERNAL_MBEDTLS 00051 00052 #include "netif/ppp/polarssl/md4.h" 00053 #define lwip_md4_context md4_context 00054 #define lwip_md4_init(context) 00055 #define lwip_md4_starts md4_starts 00056 #define lwip_md4_update md4_update 00057 #define lwip_md4_finish md4_finish 00058 #define lwip_md4_free(context) 00059 00060 #include "netif/ppp/polarssl/md5.h" 00061 #define lwip_md5_context md5_context 00062 #define lwip_md5_init(context) 00063 #define lwip_md5_starts md5_starts 00064 #define lwip_md5_update md5_update 00065 #define lwip_md5_finish md5_finish 00066 #define lwip_md5_free(context) 00067 00068 #include "netif/ppp/polarssl/sha1.h" 00069 #define lwip_sha1_context sha1_context 00070 #define lwip_sha1_init(context) 00071 #define lwip_sha1_starts sha1_starts 00072 #define lwip_sha1_update sha1_update 00073 #define lwip_sha1_finish sha1_finish 00074 #define lwip_sha1_free(context) 00075 00076 #include "netif/ppp/polarssl/des.h" 00077 #define lwip_des_context des_context 00078 #define lwip_des_init(context) 00079 #define lwip_des_setkey_enc des_setkey_enc 00080 #define lwip_des_crypt_ecb des_crypt_ecb 00081 #define lwip_des_free(context) 00082 00083 #include "netif/ppp/polarssl/arc4.h" 00084 #define lwip_arc4_context arc4_context 00085 #define lwip_arc4_init(context) 00086 #define lwip_arc4_setup arc4_setup 00087 #define lwip_arc4_crypt arc4_crypt 00088 #define lwip_arc4_free(context) 00089 00090 #endif /* !LWIP_USE_EXTERNAL_MBEDTLS */ 00091 00092 /* 00093 * Map hashes and ciphers functions to mbed TLS 00094 */ 00095 #if LWIP_USE_EXTERNAL_MBEDTLS 00096 00097 #define lwip_md4_context mbedtls_md4_context 00098 #define lwip_md4_init mbedtls_md4_init 00099 #define lwip_md4_starts mbedtls_md4_starts 00100 #define lwip_md4_update mbedtls_md4_update 00101 #define lwip_md4_finish mbedtls_md4_finish 00102 #define lwip_md4_free mbedtls_md4_free 00103 00104 #define lwip_md5_context mbedtls_md5_context 00105 #define lwip_md5_init mbedtls_md5_init 00106 #define lwip_md5_starts mbedtls_md5_starts 00107 #define lwip_md5_update mbedtls_md5_update 00108 #define lwip_md5_finish mbedtls_md5_finish 00109 #define lwip_md5_free mbedtls_md5_free 00110 00111 #define lwip_sha1_context mbedtls_sha1_context 00112 #define lwip_sha1_init mbedtls_sha1_init 00113 #define lwip_sha1_starts mbedtls_sha1_starts 00114 #define lwip_sha1_update mbedtls_sha1_update 00115 #define lwip_sha1_finish mbedtls_sha1_finish 00116 #define lwip_sha1_free mbedtls_sha1_free 00117 00118 #define lwip_des_context mbedtls_des_context 00119 #define lwip_des_init mbedtls_des_init 00120 #define lwip_des_setkey_enc mbedtls_des_setkey_enc 00121 #define lwip_des_crypt_ecb mbedtls_des_crypt_ecb 00122 #define lwip_des_free mbedtls_des_free 00123 00124 #define lwip_arc4_context mbedtls_arc4_context 00125 #define lwip_arc4_init mbedtls_arc4_init 00126 #define lwip_arc4_setup mbedtls_arc4_setup 00127 #define lwip_arc4_crypt(context, buffer, length) mbedtls_arc4_crypt(context, length, buffer, buffer) 00128 #define lwip_arc4_free mbedtls_arc4_free 00129 00130 #endif /* LWIP_USE_EXTERNAL_MBEDTLS */ 00131 00132 void pppcrypt_56_to_64_bit_key(u_char *key, u_char *des_key); 00133 00134 #endif /* PPPCRYPT_H */ 00135 00136 #endif /* PPP_SUPPORT */
Generated on Sun Jul 17 2022 08:25:29 by
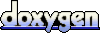