
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
pkcs11.h
Go to the documentation of this file.
00001 /** 00002 * \file pkcs11.h 00003 * 00004 * \brief Wrapper for PKCS#11 library libpkcs11-helper 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 #ifndef MBEDTLS_PKCS11_H 00026 #define MBEDTLS_PKCS11_H 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_PKCS11_C) 00035 00036 #include "x509_crt.h" 00037 00038 #include <pkcs11-helper-1.0/pkcs11h-certificate.h> 00039 00040 #if ( defined(__ARMCC_VERSION) || defined(_MSC_VER) ) && \ 00041 !defined(inline) && !defined(__cplusplus) 00042 #define inline __inline 00043 #endif 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** 00050 * Context for PKCS #11 private keys. 00051 */ 00052 typedef struct { 00053 pkcs11h_certificate_t pkcs11h_cert; 00054 int len; 00055 } mbedtls_pkcs11_context; 00056 00057 /** 00058 * Initialize a mbedtls_pkcs11_context. 00059 * (Just making memory references valid.) 00060 */ 00061 void mbedtls_pkcs11_init( mbedtls_pkcs11_context *ctx ); 00062 00063 /** 00064 * Fill in a mbed TLS certificate, based on the given PKCS11 helper certificate. 00065 * 00066 * \param cert X.509 certificate to fill 00067 * \param pkcs11h_cert PKCS #11 helper certificate 00068 * 00069 * \return 0 on success. 00070 */ 00071 int mbedtls_pkcs11_x509_cert_bind( mbedtls_x509_crt *cert, pkcs11h_certificate_t pkcs11h_cert ); 00072 00073 /** 00074 * Set up a mbedtls_pkcs11_context storing the given certificate. Note that the 00075 * mbedtls_pkcs11_context will take over control of the certificate, freeing it when 00076 * done. 00077 * 00078 * \param priv_key Private key structure to fill. 00079 * \param pkcs11_cert PKCS #11 helper certificate 00080 * 00081 * \return 0 on success 00082 */ 00083 int mbedtls_pkcs11_priv_key_bind( mbedtls_pkcs11_context *priv_key, 00084 pkcs11h_certificate_t pkcs11_cert ); 00085 00086 /** 00087 * Free the contents of the given private key context. Note that the structure 00088 * itself is not freed. 00089 * 00090 * \param priv_key Private key structure to cleanup 00091 */ 00092 void mbedtls_pkcs11_priv_key_free( mbedtls_pkcs11_context *priv_key ); 00093 00094 /** 00095 * \brief Do an RSA private key decrypt, then remove the message 00096 * padding 00097 * 00098 * \param ctx PKCS #11 context 00099 * \param mode must be MBEDTLS_RSA_PRIVATE, for compatibility with rsa.c's signature 00100 * \param input buffer holding the encrypted data 00101 * \param output buffer that will hold the plaintext 00102 * \param olen will contain the plaintext length 00103 * \param output_max_len maximum length of the output buffer 00104 * 00105 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00106 * 00107 * \note The output buffer must be as large as the size 00108 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00109 * an error is thrown. 00110 */ 00111 int mbedtls_pkcs11_decrypt( mbedtls_pkcs11_context *ctx, 00112 int mode, size_t *olen, 00113 const unsigned char *input, 00114 unsigned char *output, 00115 size_t output_max_len ); 00116 00117 /** 00118 * \brief Do a private RSA to sign a message digest 00119 * 00120 * \param ctx PKCS #11 context 00121 * \param mode must be MBEDTLS_RSA_PRIVATE, for compatibility with rsa.c's signature 00122 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00123 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00124 * \param hash buffer holding the message digest 00125 * \param sig buffer that will hold the ciphertext 00126 * 00127 * \return 0 if the signing operation was successful, 00128 * or an MBEDTLS_ERR_RSA_XXX error code 00129 * 00130 * \note The "sig" buffer must be as large as the size 00131 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00132 */ 00133 int mbedtls_pkcs11_sign( mbedtls_pkcs11_context *ctx, 00134 int mode, 00135 mbedtls_md_type_t md_alg, 00136 unsigned int hashlen, 00137 const unsigned char *hash, 00138 unsigned char *sig ); 00139 00140 /** 00141 * SSL/TLS wrappers for PKCS#11 functions 00142 */ 00143 static inline int mbedtls_ssl_pkcs11_decrypt( void *ctx, int mode, size_t *olen, 00144 const unsigned char *input, unsigned char *output, 00145 size_t output_max_len ) 00146 { 00147 return mbedtls_pkcs11_decrypt( (mbedtls_pkcs11_context *) ctx, mode, olen, input, output, 00148 output_max_len ); 00149 } 00150 00151 static inline int mbedtls_ssl_pkcs11_sign( void *ctx, 00152 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00153 int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, 00154 const unsigned char *hash, unsigned char *sig ) 00155 { 00156 ((void) f_rng); 00157 ((void) p_rng); 00158 return mbedtls_pkcs11_sign( (mbedtls_pkcs11_context *) ctx, mode, md_alg, 00159 hashlen, hash, sig ); 00160 } 00161 00162 static inline size_t mbedtls_ssl_pkcs11_key_len( void *ctx ) 00163 { 00164 return ( (mbedtls_pkcs11_context *) ctx )->len; 00165 } 00166 00167 #ifdef __cplusplus 00168 } 00169 #endif 00170 00171 #endif /* MBEDTLS_PKCS11_C */ 00172 00173 #endif /* MBEDTLS_PKCS11_H */
Generated on Sun Jul 17 2022 08:25:29 by
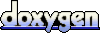