
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
options.py
00001 """ 00002 mbed SDK 00003 Copyright (c) 2011-2013 ARM Limited 00004 00005 Licensed under the Apache License, Version 2.0 (the "License"); 00006 you may not use this file except in compliance with the License. 00007 You may obtain a copy of the License at 00008 00009 http://www.apache.org/licenses/LICENSE-2.0 00010 00011 Unless required by applicable law or agreed to in writing, software 00012 distributed under the License is distributed on an "AS IS" BASIS, 00013 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 See the License for the specific language governing permissions and 00015 limitations under the License. 00016 """ 00017 from json import load 00018 from os.path import join, dirname 00019 from os import listdir 00020 from argparse import ArgumentParser, ArgumentTypeError 00021 from tools.toolchains import TOOLCHAINS 00022 from tools.targets import TARGET_NAMES, Target, update_target_data 00023 from tools.utils import argparse_force_uppercase_type, \ 00024 argparse_lowercase_hyphen_type, argparse_many, \ 00025 argparse_filestring_type, args_error, argparse_profile_filestring_type,\ 00026 argparse_deprecate 00027 00028 FLAGS_DEPRECATION_MESSAGE = "Please use the --profile argument instead.\n"\ 00029 "Documentation may be found in "\ 00030 "docs/Toolchain_Profiles.md" 00031 00032 def get_default_options_parser (add_clean=True, add_options=True, 00033 add_app_config=False): 00034 """Create a new options parser with the default compiler options added 00035 00036 Keyword arguments: 00037 add_clean - add the clean argument? 00038 add_options - add the options argument? 00039 """ 00040 parser = ArgumentParser() 00041 00042 targetnames = TARGET_NAMES 00043 targetnames.sort() 00044 toolchainlist = list(TOOLCHAINS) 00045 toolchainlist.sort() 00046 00047 parser.add_argument("-m", "--mcu", 00048 help=("build for the given MCU (%s)" % 00049 ', '.join(targetnames)), 00050 metavar="MCU") 00051 00052 parser.add_argument("-t", "--tool", 00053 help=("build using the given TOOLCHAIN (%s)" % 00054 ', '.join(toolchainlist)), 00055 metavar="TOOLCHAIN", 00056 type=argparse_many( 00057 argparse_force_uppercase_type( 00058 toolchainlist, "toolchain"))) 00059 00060 parser.add_argument("--color", 00061 help="print Warnings, and Errors in color", 00062 action="store_true", default=False) 00063 00064 parser.add_argument("--cflags", 00065 type=argparse_deprecate(FLAGS_DEPRECATION_MESSAGE), 00066 help="Deprecated. " + FLAGS_DEPRECATION_MESSAGE) 00067 00068 parser.add_argument("--asmflags", 00069 type=argparse_deprecate(FLAGS_DEPRECATION_MESSAGE), 00070 help="Deprecated. " + FLAGS_DEPRECATION_MESSAGE) 00071 00072 parser.add_argument("--ldflags", 00073 type=argparse_deprecate(FLAGS_DEPRECATION_MESSAGE), 00074 help="Deprecated. " + FLAGS_DEPRECATION_MESSAGE) 00075 00076 if add_clean: 00077 parser.add_argument("-c", "--clean", action="store_true", default=False, 00078 help="clean the build directory") 00079 00080 if add_options: 00081 parser.add_argument("--profile", dest="profile", action="append", 00082 type=argparse_profile_filestring_type, 00083 help="Build profile to use. Can be either path to json" \ 00084 "file or one of the default one ({})".format(", ".join(list_profiles())), 00085 default=[]) 00086 if add_app_config: 00087 parser.add_argument("--app-config", default=None, dest="app_config", 00088 type=argparse_filestring_type, 00089 help="Path of an app configuration file (Default is to look for 'mbed_app.json')") 00090 00091 return parser 00092 00093 def list_profiles (): 00094 """Lists available build profiles 00095 00096 Checks default profile directory (mbed-os/tools/profiles/) for all the json files and return list of names only 00097 """ 00098 return [fn.replace(".json", "") for fn in listdir(join(dirname(__file__), "profiles")) if fn.endswith(".json")] 00099 00100 def extract_profile (parser, options, toolchain, fallback="develop"): 00101 """Extract a Toolchain profile from parsed options 00102 00103 Positional arguments: 00104 parser - parser used to parse the command line arguments 00105 options - The parsed command line arguments 00106 toolchain - the toolchain that the profile should be extracted for 00107 """ 00108 profiles = [] 00109 filenames = options.profile or [join(dirname(__file__), "profiles", 00110 fallback + ".json")] 00111 for filename in filenames: 00112 contents = load(open(filename)) 00113 if toolchain not in contents: 00114 args_error(parser, ("argument --profile: toolchain {} is not" 00115 " supported by profile {}").format(toolchain, 00116 filename)) 00117 profiles.append(contents) 00118 00119 return profiles 00120 00121 def mcu_is_enabled(parser, mcu): 00122 if "Cortex-A" in TARGET_MAP[mcu].core: 00123 args_error( 00124 parser, 00125 ("%s Will be supported in mbed OS 5.6. " 00126 "To use the %s, please checkout the mbed OS 5.4 release branch. " 00127 "See https://developer.mbed.org/platforms/Renesas-GR-PEACH/#important-notice " 00128 "for more information") % (mcu, mcu)) 00129 return True 00130 00131 def extract_mcus(parser, options): 00132 try: 00133 if options.source_dir: 00134 for source_dir in options.source_dir: 00135 Target.add_extra_targets(source_dir) 00136 update_target_data() 00137 except KeyError: 00138 pass 00139 targetnames = TARGET_NAMES 00140 targetnames.sort() 00141 try: 00142 return argparse_many(argparse_force_uppercase_type(targetnames, "MCU"))(options.mcu) 00143 except ArgumentTypeError as exc: 00144 args_error(parser, "argument -m/--mcu: {}".format(str(exc))) 00145
Generated on Sun Jul 17 2022 08:25:29 by
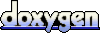