
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ns_mdns_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 #ifndef _NS_MDNS_API_H_ 00016 #define _NS_MDNS_API_H_ 00017 00018 /** 00019 * \file ns_mdns_api.h 00020 * \brief Nanostack mDNS-SD API. 00021 */ 00022 00023 /*! 00024 * \struct ns_mdns_t 00025 * \brief Structure for Nanostack mDNS instance 00026 */ 00027 typedef struct ns_mdns *ns_mdns_t; /**< Instance */ 00028 00029 /*! 00030 * \struct ns_mdns_service_t 00031 * \brief Structure for Nanostack mDNS service instance 00032 */ 00033 typedef struct ns_mdns_service *ns_mdns_service_t; /**< Service instance */ 00034 00035 /*! 00036 * \struct ns_mdns_service_param_t 00037 * \brief Structure for mDNS service parameters 00038 */ 00039 typedef struct ns_mdns_service_param 00040 { 00041 const char *service_type; /**< Null-terminated string owned by the caller */ 00042 uint16_t service_port; /**< Service Port number */ 00043 const uint8_t *(*service_get_txt)(void); /**< Call-back function, which returns a pointer to the service TXT record (null-terminated). 00044 If the service does not provide any TXT record, this parameter must be set to NULL. */ 00045 } ns_mdns_service_param_t; 00046 00047 /** 00048 * \brief Start mDNS server 00049 * 00050 * \param server_name NULL terminated string, max length 63 characters 00051 * 00052 * \param ttl time-to-live value in seconds, if set to 0 default value is used 00053 * 00054 * \param ttl_ip time-to-live in hop count, if set to 0 default value is used 00055 * 00056 * \param interface_id ID of the network interface where mDNS will operate 00057 * 00058 * \return mDNS server instace or NULL in case of failure.. 00059 */ 00060 ns_mdns_t ns_mdns_server_start(const char *server_name, uint32_t ttl, uint32_t ttl_ip, int8_t interface_id); 00061 00062 /** 00063 * \brief Stop mDNS server 00064 * 00065 * \param ns_mdns_instance Server instance received from ns_mdns_server_start 00066 * 00067 */ 00068 void ns_mdns_server_stop(ns_mdns_t ns_mdns_instance); 00069 00070 /** 00071 * \brief Register service to mDNS server 00072 * 00073 * \param ns_mdns_instance Server instance received from ns_mdns_server_start 00074 * 00075 * \param service Parameters for service 00076 * 00077 * \return mDNS Service descriptor or NULL in case of failure. 00078 * 00079 */ 00080 ns_mdns_service_t ns_mdns_service_register(ns_mdns_t ns_mdns_instance, ns_mdns_service_param_t *service); 00081 00082 /** 00083 * \brief Unregister service from mDNS server 00084 * 00085 * \param service_desc mDNS Service descriptor received from call to ns_mdns_service_register. 00086 */ 00087 00088 void ns_mdns_service_unregister(ns_mdns_service_t service_desc); 00089 00090 /** 00091 * \brief Send mDNS announcement. Application should call this method once application 00092 * advertised parameters has changed. 00093 * 00094 * \param ns_mdns_instance Server instance received from ns_mdns_server_start 00095 */ 00096 00097 void ns_mdns_announcement_send(ns_mdns_t ns_mdns_instance); 00098 00099 #endif /* _NS_MDNS_API_H_ */ 00100
Generated on Sun Jul 17 2022 08:25:28 by
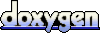