
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_pana_parameters_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 #ifndef NET_PANA_PARAMETERS_API_H_ 00016 #define NET_PANA_PARAMETERS_API_H_ 00017 00018 #include "ns_types.h" 00019 00020 /** 00021 * \file net_pana_parameters_api.h 00022 * \brief An API for setting up or changing PANA library parameters. 00023 * 00024 * \section set-pana-param Setting up PANA parameters. 00025 * - net_pana_parameter_set(), A function to set up PANA library parameters. 00026 * \section check-pana-param Checking existing PANA setup. 00027 * - net_pana_parameter_read(), A function to read current PANA library setup. 00028 */ 00029 00030 /*! 00031 * \struct pana_lib_parameters_s 00032 * \brief PANA library dynamic parameters. 00033 */ 00034 /** The structure defines PANA library parameters.*/ 00035 typedef struct pana_lib_parameters_s { 00036 uint16_t PCI_IRT; /**< Initial PCI timeout in seconds, default 10. */ 00037 uint16_t PCI_MRT; /**< Max PCI timeout value in seconds, default 60. */ 00038 uint8_t PCI_MRC; /**< PCI_MRC Max PCI retransmission attempts, default 5. */ 00039 uint16_t REQ_IRT; /**< PCI_MRC Initial request timeout in seconds, default 20. */ 00040 uint16_t REQ_MRT; /**< Max request timeout value, default 60. */ 00041 uint16_t REQ_MRC; /**< Max request retransmission attempts, default 4. */ 00042 uint16_t AUTHENTICATION_TIMEOUT; /**< Max timeout for authencication, default 100 seconds. */ 00043 uint16_t KEY_UPDATE_THRESHOLD; /**< Gap in seconds before the server starts to send a new network key, default 10. */ 00044 uint8_t KEY_ID_MAX_VALUE; /**< Define resolution for key ID [1-KEY_ID_MAX_VALUE], default 255. MIN accepted value is 3.*/ 00045 uint16_t EAP_FRAGMENT_SIZE; /**< Define EAP fragment slot size. Fragmentation is activated when EAP payload is more than 920. Default 296. */ 00046 uint8_t AUTH_COUNTER_MAX; /**< Define PANA session re-authentication limit. When the MAX value is reached the server does not respond to the PANA notify request. Default 0xff. */ 00047 } pana_lib_parameters_s; 00048 00049 /** 00050 * \brief A function to set PANA library parameters. 00051 * 00052 * Note: This function should be called after net_init_core() and definitely 00053 * before creating any 6LoWPAN interface. 00054 * 00055 * For future compatibility, to support extensions to this structure, read 00056 * the current parameters using net_pana_parameter_read(), 00057 * modify known fields, then set. 00058 * 00059 * \param parameter_ptr A pointer for PANA parameters. 00060 * 00061 * \return 0, Change OK. 00062 * \return -1, Invalid values. 00063 * \return -2, PANA not supported. 00064 * 00065 */ 00066 extern int8_t net_pana_parameter_set(const pana_lib_parameters_s *parameter_ptr); 00067 00068 00069 /** 00070 * \brief A function to read existing PANA library parameters. 00071 * 00072 * \param parameter_ptr An output pointer for PANA parameters. 00073 * 00074 * \return 0, Read OK. 00075 * \return -1, PANA not supported. 00076 */ 00077 extern int8_t net_pana_parameter_read(pana_lib_parameters_s *parameter_ptr); 00078 00079 #endif /* NET_PANA_PARAMETERS_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
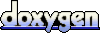