
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_nvm_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2013-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 /** 00015 * \file net_nvm_api.h 00016 * \brief Library PANA NVM API for Client and Server. 00017 * 00018 * \section server-api Server NVM API 00019 * 00020 * - pana_server_nvm_callback_set(), Initialize PANA server NVM functionality. 00021 * - pana_server_restore_from_nvm(), Load PANA server base and security material from NVM. 00022 * - pana_server_nvm_client_session_load(), Load client session from NVM. 00023 * 00024 * \section client-api Client NVM API 00025 * 00026 * nw_nvm.c use already this API and the application can just use net_nvm_api.h. 00027 * 00028 * - pana_client_nvm_callback_set(), Initialize PANA session NVM. 00029 * 00030 */ 00031 #ifndef PANA_NVM_API_H_ 00032 #define PANA_NVM_API_H_ 00033 00034 #include "ns_types.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 00041 /** 00042 * \brief Pana client and common data structure for get / Update callback's 00043 * 00044 * Client: [session address 16-bytes] + [session data 70-bytes] , Total 86 bytes 00045 * 00046 * Server: [offset 2-bytes] + [session address 16-bytes] + [session port 2-bytes] + [session id 4-bytes] + [session data 92-bytes], Total 116 bytes 00047 */ 00048 00049 /** 00050 * Size of Pana client session data without session address 00051 */ 00052 #define PANA_CLIENT_NVM_SESSION_BUF_SIZE 70 00053 /** 00054 * Size of Pana client session data with session address 00055 */ 00056 #define PANA_CLIENT_NVM_SESSION_BUF_SIZE_WITH_ADDRESS 86 00057 00058 /** 00059 * Size of data for Server client Uodate and get callback 00060 * 00061 */ 00062 #define PANA_SERVER_CLIENT_NVM_SESSION_BUF_SIZE 116 00063 00064 /** 00065 * Size of data for Server material update and restore operation 00066 * 00067 */ 00068 #define PANA_SERVER_MATERIAL_BUF_SIZE 90 00069 /*! 00070 * \enum pana_nvm_update_process_t 00071 * \brief PANA server NVM update states. 00072 */ 00073 typedef enum pana_nvm_update_process_t { 00074 PANA_SERVER_MATERIAL_UPDATE, /**< PANA server security material update. */ 00075 PANA_SERVER_CLIENT_SESSION_UPDATE, /**< PANA client session update. */ 00076 PANA_SERVER_CLIENT_SESSION_REMOVE_UPDATE, /**< PANA client session remove. */ 00077 } pana_nvm_update_process_t; 00078 00079 /*! 00080 * \enum pana_client_nvm_update_process_t 00081 * \brief PANA client NVM update states. 00082 */ 00083 typedef enum pana_client_nvm_update_process_t { 00084 PANA_CLIENT_SESSION_UPDATE, /**< PANA client session update. */ 00085 PANA_CLIENT_SESSION_REMOVE, /**< PANA client session remove. */ 00086 } pana_client_nvm_update_process_t; 00087 00088 /*! 00089 * \struct wpan_nvm_params_t 00090 * \brief Network nvm parameters. 00091 */ 00092 typedef struct wpan_nvm_params { 00093 uint16_t pan_id; /**< WPAN Pan-id. */ 00094 uint32_t mac_security_frame_counter; /**< Mac security counter. */ 00095 uint32_t mle_securit_counter; /**< MLE security counter. */ 00096 } wpan_nvm_params_t; 00097 00098 /** 00099 * \brief NVM memory user callback for updated parameters 00100 * 00101 * \param parameters Updated wpan parameters 00102 */ 00103 typedef void wpan_params_updated(wpan_nvm_params_t *parameters); 00104 00105 /** 00106 * \brief NVM memory user for get parameter->pan_id network setup 00107 * 00108 * \param parameters Pointer for request parameters parameter->pan_id is configured for proper setup 00109 * 00110 * \return true when configure is loaded to buffer 00111 * \return false when there is no proper data for current wpan 00112 */ 00113 typedef bool wpan_params_get(wpan_nvm_params_t *parameters); 00114 00115 /** 00116 * \brief Pana client session update callback 00117 * 00118 * \param pan_id define which pan session is 00119 * \param process PANA_CLIENT_SESSION_UPDATE or PANA_CLIENT_SESSION_REMOVE 00120 */ 00121 typedef void pana_client_session_update_cb(uint16_t pan_id, pana_client_nvm_update_process_t process); 00122 00123 /** 00124 * \brief Pana client discover session from NVM user based on pan id 00125 * 00126 * \param pan_id define which pan session is 00127 * 00128 * \return true When Session is stored to behind given session buffer 00129 * \return false NVM can't detect session for given pan id 00130 */ 00131 typedef bool pana_client_session_get_cb(uint16_t pan_id); 00132 00133 /** 00134 * \brief Pana server call this when it create /Update / delete client session or update Server material 00135 * 00136 * \param operation Pana server NVM update 00137 * 00138 * \return session offset . Requirement for operation PANA_SERVER_CLIENT_SESSION_UPDATE 00139 */ 00140 typedef uint16_t pana_server_update_cb(pana_nvm_update_process_t operation); 00141 00142 /** 00143 * \brief Pana server call this when discover client session from NVM for given address 00144 * 00145 * \param linklocal_address Link local address for sesion 00146 * 00147 * \return true When Session is stored to behind given session buffer 00148 * \return false NVM can't detect session for given address 00149 */ 00150 typedef bool pana_server_session_get_cb(uint8_t *linklocal_address); 00151 00152 /** 00153 * \brief Pana server call this when discover client session from NVM for pana session 00154 * 00155 * \param session_id Pana session Id 00156 * 00157 * \return true When Session is stored to behind given session buffer 00158 * \return false NVM can't detect session for given session id 00159 */ 00160 typedef bool pana_server_session_get_by_id_cb(uint32_t session_id); 00161 00162 /* NVM API PART */ 00163 /** 00164 * \brief PANA server NVM functionality initialization. 00165 * 00166 * \param update_cb A function pointer to NVM update process. 00167 * \param nvm_get A function pointer for discover session data from NVM by link local address 00168 * \param nvm_session_get A function pointer for discover session data from NVM by pana session id 00169 * \param nvm_static_buffer A pointer to application allocated static memory, minimum size PANA_SERVER_CLIENT_NVM_SESSION_BUF_SIZE (116 bytes). 00170 * 00171 * 00172 * \return 0, Init OK. 00173 * \return -1, Null parameter detect. 00174 * 00175 */ 00176 extern int8_t pana_server_nvm_callback_set(pana_server_update_cb *update_cb, pana_server_session_get_cb *nvm_get, pana_server_session_get_by_id_cb *nvm_session_get, uint8_t *nvm_static_buffer); 00177 /** 00178 * \brief PANA server base restore from NVM. 00179 * 00180 * \param nvm_data A pointer to PANA server base data. 00181 * \param interface_id Interface ID. 00182 * 00183 * \return 0, Restore OK. 00184 * \return -1, Memory allocation fail. 00185 * 00186 */ 00187 extern int8_t pana_server_restore_from_nvm(uint8_t *nvm_data, int8_t interface_id); 00188 /** 00189 * \brief PANA client session load from NVM API. 00190 * 00191 * \param nvm_pointer A pointer PANA client session. 00192 * 00193 * \return 0, Restore OK. 00194 * \return -1, Memory allocation fail. 00195 * 00196 */ 00197 extern int8_t pana_server_nvm_client_session_load(uint8_t *nvm_pointer); 00198 00199 /** 00200 * \brief PANA client NVM functionality init. 00201 * 00202 * \param nvm_update A function pointer to NVM update process. 00203 * \param nvm_get A function pointer for discover session from NVM for given Pan-id 00204 * \param nvm_static_buffer A pointer to application allocated static memory, minimum size for session 86 bytes + 16-bit pan-id. 00205 * 00206 * 00207 * \return 0, Init OK. 00208 * \return -1, Null parameter detect. 00209 * 00210 */ 00211 extern int8_t pana_client_nvm_callback_set(pana_client_session_update_cb *nvm_update, pana_client_session_get_cb *nvm_get,uint8_t *nvm_static_buffer); 00212 00213 /** 00214 * \brief Clean node persistent data and all PANA client sessions from the stack. 00215 * 00216 * This function disables the network ID filter, sets EUID-16 to 0xffff and removes PANA client sessions. It is only for client purposes. 00217 * 00218 * 00219 * \return 0, Clean OK. 00220 * \return -1, Stack is active. 00221 * 00222 */ 00223 extern int8_t net_nvm_data_clean(int8_t interface_id); 00224 00225 /** 00226 * \brief Enable and init network NVM parameter automatic update. 00227 * 00228 * This function enables MAC and MLE protocol critical components update process to NVM memory user. 00229 * 00230 * \param interface_id Interface ID. 00231 * \param nvm_update_cb Function pointer for update NVM 00232 * \param nvm_get_cb Function for stack to request setup from NVM. 00233 * 00234 * 00235 * \return 0, Init OK. 00236 * \return -1, Unknown Interface. 00237 * \return -2, Memory allocation fail. 00238 * 00239 */ 00240 extern int8_t net_nvm_wpan_params_storage_enable(int8_t interface_id, wpan_params_updated *nvm_update_cb, wpan_params_get *nvm_get_cb); 00241 00242 /** 00243 * \brief Reset stored WPAN parameter's at interface . 00244 * 00245 * This function clean state at WPAN params at stack. Force Request from NVM 00246 * 00247 *\param interface_id Interface ID. 00248 * 00249 * \return 0, reset OK. 00250 * \return -1, Unknown Interface. 00251 * 00252 */ 00253 extern int8_t net_nvm_wpan_params_storage_reset(int8_t interface_id); 00254 00255 00256 /** 00257 * \brief Disable stored WPAN parameter's to interface . 00258 * 00259 * 00260 *\param interface_id Interface ID. 00261 * 00262 * \return 0, Disable OK. 00263 * \return -1, Unknown Interface. 00264 * 00265 */ 00266 extern int8_t net_nvm_wpan_params_storage_disable(int8_t interface_id); 00267 00268 00269 #ifdef __cplusplus 00270 } 00271 #endif 00272 #endif /* PANA_NVM_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
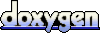