
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
net_ipv6_api.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** 00016 * \file net_ipv6_api.h 00017 * \brief IPv6 configuration API. 00018 */ 00019 00020 #ifndef NET_IPV6_API_H_ 00021 #define NET_IPV6_API_H_ 00022 00023 #include "ns_types.h" 00024 00025 /** 00026 * \brief Set maximum IPv6 fragmented datagram reception size. 00027 * 00028 * Set the maximum size limit for fragmented datagram reception. 00029 * 00030 * RFC 2460 requires this to be at least 1500. It should also be at least 00031 * as large as the MTU of each attached link. 00032 * 00033 * \param frag_mru The fragmented Maximum Receive Unit in octets. 00034 * \return 0 Change OK - actual MRU is at least the requested value. 00035 * \return <0 Change invalid - unable to set the specified MRU. 00036 */ 00037 int8_t arm_nwk_ipv6_frag_mru(uint16_t frag_mru); 00038 00039 /** 00040 * \brief Configure automatic flow label calculation. 00041 * 00042 * Enable or disable automatic generation of IPv6 flow labels for outgoing 00043 * packets. 00044 * 00045 * \param auto_flow_label True to enable auto-generation. 00046 */ 00047 void arm_nwk_ipv6_auto_flow_label(bool auto_flow_label); 00048 00049 /** 00050 * \brief Set the key for opaque IPv6 interface identifiers 00051 * 00052 * This call sets the secret key used to generate opaque interface identifiers, 00053 * as per RFC 7217. Once this has been set, all interfaces will use opaque 00054 * interface identifiers by default. If secret_key is NULL, opaque interface 00055 * identifiers will be disabled. 00056 * 00057 * Correct implementation of RFC 7217 would require that this key be 00058 * randomly generated at first bootstrap, and thereafter remain constant, which 00059 * would require non-volatile storage. The next closest alternative would be 00060 * to base this on a MAC address. 00061 * 00062 * \param secret_key A pointer to secret key (will be copied by call). 00063 * \param key_len The length of the key. 00064 * 00065 * \return 0 key set okay. 00066 * \return <0 key set failed (for example due to memory allocation). 00067 */ 00068 int8_t arm_nwk_ipv6_opaque_iid_key(const void *secret_key, uint8_t key_len); 00069 00070 /** 00071 * \brief Enable/disable opaque IPv6 interface identifiers by interface 00072 * 00073 * Enable or disable RFC 7217 opaque IIDs generated by SLAAC, per interface. 00074 * By default opaque IIDs are enabled if the opaque key is set. If disabled, 00075 * SLAAC IIDs will be EUI-64-based as per RFC 4291. 00076 * 00077 * \param interface_id Interface ID. 00078 * \param enable True to enable. 00079 * \return 0 enabled/disabled OK. 00080 * \return <0 failed (for example invalid interface ID). 00081 * 00082 */ 00083 int8_t arm_nwk_ipv6_opaque_iid_enable(int8_t interface_id, bool enable); 00084 00085 #endif /* NET_IPV6_API_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
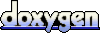