
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mlme.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2013-2017 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 00015 /** \file mlme.h 00016 * \brief MLME API 00017 */ 00018 00019 #ifndef MLME_H_ 00020 #define MLME_H_ 00021 00022 #include <stdbool.h> 00023 #include "mac_common_defines.h" 00024 #include "net_interface.h" 00025 00026 /** 00027 * @brief struct mlme_pan_descriptor_t PAN descriptor 00028 * 00029 * See IEEE standard 802.15.4-2006 (table 55) for more details 00030 */ 00031 typedef struct mlme_pan_descriptor_s { 00032 unsigned CoordAddrMode:2; /**<Coordinator address mode MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00033 uint16_t CoordPANId; /**<PAN-id */ 00034 uint8_t CoordAddress[8]; /**< Coordinator based CoordAddrMode */ 00035 uint8_t LogicalChannel; /**< Pan's Logical channel */ 00036 uint8_t ChannelPage; /**< Channel Page*/ 00037 uint8_t SuperframeSpec[2]; /**< Superframe specification */ 00038 bool GTSPermit:1; /**< true = GTS enabled false = disabled */ 00039 uint8_t LinkQuality; /**< Link quality based on received packet to Coordinator 0-0xff */ 00040 uint32_t Timestamp; /**< Time stamp for received packet */ 00041 uint8_t SecurityFailure; /**< Indicates payload security failure */ 00042 mlme_security_t Key; /**< PAN beacon used security AUX header */ 00043 } mlme_pan_descriptor_t; 00044 00045 /** 00046 * @brief struct mlme_command_type_t Command type enumeration 00047 * 00048 * See IEEE standard 802.15.4-2006 (table 82) for more details 00049 */ 00050 typedef enum { 00051 ASSOCIATION_REQUEST = 1, /**<Assocation request (Not supported)*/ 00052 ASSOCIATION_RESPONSE = 2, /**<Assocation response (Not supported)*/ 00053 DISASSOCIATION_NOTIFICATION = 3, /**<Disasocation Notification (Not supported)*/ 00054 DATA_REQUEST = 4, /**<Data Request */ 00055 PAN_ID_CONFLICT_NOTIFICATION = 5, /**<Pan ID conflict notification (Not supported)*/ 00056 ORPHAN_NOTIFICATION = 6, /**<Orphan Notification (Not supported)*/ 00057 BEACON_REQUEST = 7, /**<Beacon request */ 00058 COORDINATOR_REALIGNMENT = 8, /**<Coordinator Realignment (Not supported)*/ 00059 GTS_REQUEST = 9 /**<GTS request (Not supported)*/ 00060 //Reserved 00061 } mlme_command_type_t; 00062 00063 /** 00064 * @brief enum mlme_loss_reason_t loss reason enumeration 00065 * 00066 * See IEEE standard 802.15.4-2006 (table 75) for more details 00067 */ 00068 typedef enum { 00069 PAN_ID_CONFLICT, /**<Pan id conflict (Not supported)*/ 00070 REALIGNMENT, /**<Cordinator Realignment(Not supported)*/ 00071 BEACON_LOST /**<FHSS indicate by this when it loose synch to coordinator*/ 00072 } mlme_loss_reason_t; 00073 00074 /** 00075 * @brief struct mlme_key_usage_descriptor_t Key usage descriptor 00076 * 00077 * See IEEE standard 802.15.4-2006 (table 90) for more details 00078 */ 00079 typedef struct mlme_key_usage_descriptor_s { 00080 unsigned FrameType:3; /**<0 = Beacon Frame, 1 = Data Frame or 3 Command Frame */ 00081 unsigned CommandFrameIdentifier:4; /**< Set this part only when FrameType is 3 */ 00082 } mlme_key_usage_descriptor_t; 00083 00084 /** 00085 * @brief struct mlme_key_device_descriptor_t Key usage descriptor 00086 * 00087 * See IEEE standard 802.15.4-2006 (table 91) for more details 00088 */ 00089 typedef struct mlme_key_device_descriptor_s { 00090 uint8_t DeviceDescriptorHandle; /**< User defined unique ID to key User */ 00091 bool UniqueDevice:1; /**< true = Key description is for Key Pair Key usage only, False = group key */ 00092 bool Blacklisted:1; /**< true = Description is black listed, False = valid to use */ 00093 } mlme_key_device_descriptor_t; 00094 00095 /** 00096 * @brief enum mlme_security_type_t Security type enumeration 00097 * 00098 * See IEEE standard 802.15.4-2006 (table 95) for more details 00099 */ 00100 typedef enum { 00101 SEC_NONE = 0, /**< No payload encode and authentication */ 00102 SEC_MIC32 = 1, /**< No payload encode with 32-bit MIC authentication */ 00103 SEC_MIC64 = 2, /**< No payload encode with 64-bit MIC authentication */ 00104 SEC_MIC128 = 3, /**< No payload encode with 128-bit MIC authentication */ 00105 SEC_ENC = 4, /**< Payload encode enabled and without authentication */ 00106 SEC_ENC_MIC32 = 5, /**< Payload encode enabled with 32-bit MIC authentication */ 00107 SEC_ENC_MIC64 = 6, /**< Payload encode enabled with 64-bit MIC authentication */ 00108 SEC_ENC_MIC128 = 7 /**< Payload encode enabled with 128-bit MIC authentication */ 00109 } mlme_security_type_t; 00110 00111 /** 00112 * @brief struct mlme_security_level_descriptor_t Security level descriptor 00113 * 00114 * See IEEE standard 802.15.4-2006 (table 92) for more details 00115 */ 00116 typedef struct mlme_security_level_descriptor_s { 00117 unsigned FrameType:3; /**<0 = Beacon Frame, 1 = Data Frame or 3 Command Frame */ 00118 unsigned CommandFrameIdentifier:4; /**< Set this part only when FrameType is 3 */ 00119 unsigned SecurityMinimum:3; /**< Define Minimum acceptable security level for RX */ 00120 bool DeviceOverrideSecurityMinimum:1; /**< Set false */ 00121 } mlme_security_level_descriptor_t; 00122 00123 /** 00124 * @brief struct mlme_device_descriptor_t Device descriptor 00125 * 00126 * See IEEE standard 802.15.4-2006 (table 93) for more details 00127 */ 00128 typedef struct mlme_device_descriptor_s { 00129 uint16_t PANId; /**< Pan-id */ 00130 uint16_t ShortAddress; /**< Device 16-bit short address 0xffff means not defined */ 00131 uint8_t ExtAddress[8]; /**< Device Extended 64-bit address */ 00132 uint32_t FrameCounter; /**< Security Frame counter */ 00133 bool Exempt:1; /**< Set false */ 00134 } mlme_device_descriptor_t; 00135 00136 /** 00137 * @brief struct mlme_key_id_lookup_descriptor_t Key id lookup descriptor 00138 * 00139 * See IEEE standard 802.15.4-2006 (table 94) for more details 00140 */ 00141 typedef struct mlme_key_id_lookup_descriptor_s { 00142 uint8_t LookupData[9]; /**< Key Lookup data */ 00143 unsigned LookupDataSize:1; /**< Key Lookup data size 0= 5 1 is 9 bytes */ 00144 } mlme_key_id_lookup_descriptor_t; 00145 00146 00147 /** 00148 * @brief struct mlme_key_descriptor_entry_t Key descriptor entry 00149 * 00150 * See IEEE standard 802.15.4-2006 (table 89) for more details 00151 */ 00152 typedef struct mlme_key_descriptor_entry_s { 00153 mlme_key_id_lookup_descriptor_t *KeyIdLookupList; /**< List of Key lookup data for this descriptor*/ 00154 uint8_t KeyIdLookupListEntries; /**< Number of entries in KeyIdLookupList*/ 00155 mlme_key_device_descriptor_t *KeyDeviceList; /**< List of descriptor user entries indicating which devices are valid or blacklisted */ 00156 uint8_t KeyDeviceListEntries; /**< Number of entries in KeyDeviceList*/ 00157 mlme_key_usage_descriptor_t *KeyUsageList; /**< List of descriptor entries indicating which frame types this key may be used with*/ 00158 uint8_t KeyUsageListEntries; /**< Number of entries in KeyUsageList*/ 00159 uint8_t Key[16]; /**< Actual value of Security key*/ 00160 }mlme_key_descriptor_entry_t; 00161 00162 /** 00163 * @brief MLME primitive error statuses 00164 * 00165 * See IEEE standard 802.15.4-2006 for more details 00166 */ 00167 #define MLME_SUCCESS 0x00 /**< The requested operation was completed successfully*/ 00168 #define MLME_BUSY_CHAN 0xe1 /**< CSMA-CA fail*/ 00169 #define MLME_BUSY_RX 0x01 /**< The radio is asked to change its state while receiving */ 00170 #define MLME_BUSY_TX 0x02 /**< The radio is asked to change its state while transmitting. */ 00171 #define MLME_FORCE_TRX_OFF 0x03 /**< The radio is to be switched off immediately */ 00172 #define MLME_IDLE 0x04 /**< The CCA attempt has detected an idle channel */ 00173 #define MLME_RX_ON 0x06 /**< The radio is in or is to be configured into the receiver enabled state. */ 00174 #define MLME_TRX_OFF 0x08 /**< The radio is in or is to be configured into the receiver enabled state. */ 00175 #define MLME_TX_ON 0x09 /**< The radio is in or is to be configured into the receiver enabled state. */ 00176 #define MLME_COUNTER_ERROR 0xdb /**< Originated messages security counter is not valid */ 00177 #define MLME_IMPROPER_KEY_TYPE 0xdc /**< Received Messages key used is agains't key usage policy */ 00178 #define MLME_IMPROPER_SECURITY_LEVEL 0xdd /**< Received Messages security level does not meet minimum security level */ 00179 #define MLME_UNSUPPORTED_LEGACY 0xde /**< The received frame was purportedly secured using security based on IEEE Std 802.15.4-2003, and such security is not supported by this standard. */ 00180 #define MLME_UNSUPPORTED_SECURITY 0xdf /**< The received frame security is not supported */ 00181 #define MLME_SECURITY_FAIL 0xe4 /**< Cryptographic processing of the received secured frame failed. */ 00182 #define MLME_FRAME_TOO_LONG 0xe5 /**< Either a frame resulting from processing has a length that is greater than aMaxPHYPacketSize */ 00183 #define MLME_INVALID_HANDLE 0xe7 /**< Status for Purge request when Mac not detect proper queued message*/ 00184 #define MLME_INVALID_PARAMETER 0xe8 /**< A parameter in the primitive is either not supported or is out of the valid range */ 00185 #define MLME_TX_NO_ACK 0xe9 /**< No ack was received after macMaxFrameRetries */ 00186 #define MLME_NO_BEACON 0xea /**< A scan operation failed to find any network beacons */ 00187 #define MLME_NO_DATA 0xeb /**< No response data were available following a request */ 00188 #define MLME_NO_SHORT_ADDRESS 0xec /**< Operation fail because 16-bit address is not allocated */ 00189 #define MLME_PAN_ID_CONFLICT 0xee /**< A PAN identifier conflict has been detected and communicated to the PAN coordinator. */ 00190 #define MLME_TRANSACTION_EXPIRED 0xf0 /**< The transaction has expired and its information was discarded */ 00191 #define MLME_TRANSACTION_OVERFLOW 0xf1 /**< MAC have no capacity to store the transaction */ 00192 #define MLME_UNAVAILABLE_KEY 0xf3 /**< Received message use unknown key, or the originating device is unknown or is blacklisted with that particular key */ 00193 #define MLME_UNSUPPORTED_ATTRIBUTE 0xf4 /**< A SET/GET request was issued with the unsupported identifier */ 00194 #define MLME_INVALID_ADDRESS 0xf5 /**< A request to send data was unsuccessful because neither the source address parameters nor the destination address parameters were present.*/ 00195 #define MLME_INVALID_INDEX 0xf9 /**< An attempt to write to a MAC PIB attribute that is in a table failed because the specified table index was out of range. */ 00196 #define MLME_LIMIT_REACHED 0xfa /**< A scan operation terminated prematurely because the number of PAN descriptors stored reached an implementation- specified maximum */ 00197 #define MLME_READ_ONLY 0xfb /**< A SET request was issued with the identifier of an attribute that is read only.*/ 00198 #define MLME_SCAN_IN_PROGRESS 0xfc /**< Request scan request fail when scan is already active */ 00199 //NOT-standard 00200 #define MLME_DATA_POLL_NOTIFICATION 0xff /**< Thread requirement feature COMM status status for indicate for successfully data poll event to refresh neighbour data */ 00201 00202 /** 00203 * @brief enum mac_scan_type_t MAC scan type 00204 * 00205 * See IEEE standard 802.15.4-2006 (table 67) for more details 00206 */ 00207 typedef enum { 00208 MAC_ED_SCAN_TYPE = 0, /**< Energy detection scan operation */ 00209 MAC_ACTIVE_SCAN = 1, /**< Active scan operation */ 00210 MAC_PASSIVE_SCAN = 2, /**< Passive scan operation */ 00211 MAC_ORPHAN_SCAN = 3 /**< Orphan scan operation (Not supported) */ 00212 } mac_scan_type_t; 00213 00214 /** 00215 * @brief enum mlme_attr_t MLME attributes used with GET and SET primitives 00216 * 00217 * See IEEE standard 802.15.4-2006 (table 86) for more details 00218 */ 00219 typedef enum { 00220 phyCurrentChannel = 0x00, /*<Current RF channel*/ 00221 macAckWaitDuration = 0x40, /*<Integer, n. of symbols*/ 00222 macAssociatedPANCoord = 0x56, /*<Boolean, associated to PAN coordinator*/ 00223 macAssociationPermit = 0x41, /*<Boolean, if association is allowed (in coordinator)*/ 00224 macAutoRequest = 0x42, /*<Boolean, if device automatically sends data request on beacon*/ 00225 macBattLifeExt = 0x43, /*<Boolean, if BLE is enabled*/ 00226 macBattLifeExtPeriods = 0x44, /*<Integer 6-41, BLE back off periods */ 00227 macBeaconPayload = 0x45, /*<Set of bytes, beacon payload*/ 00228 macBeaconPayloadLength = 0x46, /*<Integer 0-MaxPayLoadLen*/ 00229 macBeaconOrder = 0x47, /*<Integer 0–15, Beacon tx period, 15 = no periodic beacon*/ 00230 macBeaconTxTime = 0x48, /*<Integer 0x000000–0xffffff, symbols, when last beacon was transmitted*/ 00231 macBSN = 0x49, /*<Integer 0x00–0xff, Beacon sequence number*/ 00232 macCoordExtendedAddress = 0x4a, /*<64-bit IEEE of coordinator*/ 00233 macCoordShortAddress = 0x4b, /*<16-bit addr of coordinator*/ 00234 macDSN = 0x4c, /*<Integer 0x00–0xff, Data frame sequence number*/ 00235 macGTSPermit = 0x4d, /*<Boolean, GTS allowed?*/ 00236 macMaxBE = 0x57, /*<Integer 3–8, max value of back off exponent*/ 00237 macMaxCSMABackoffs = 0x4e, /*<Integer 0–5*/ 00238 macMaxFrameTotalWaitTime = 0x58,/*<Integer, max of CAP symbols while waiting for data requested by DREQ or PEND*/ 00239 macMaxFrameRetries = 0x59, /*<Integer 0–7*/ 00240 macMinBE = 0x4f, /*<Integer 0–macMaxBE*/ 00241 macPANId = 0x50, /*<PAN ID, 16 bits*/ 00242 macPromiscuousMode = 0x51, /*<Boolean*/ 00243 macResponseWaitTime = 0x5a, /*<Integer 2–64 The maximum time in SuperFrameDurations to wait for responses*/ 00244 macRxOnWhenIdle = 0x52, /*<Boolean*/ 00245 macSecurityEnabled = 0x5d, /*<Boolean*/ 00246 macShortAddress = 0x53, /*<Short address, 16 bits*/ 00247 macSuperframeOrder = 0x54, /*<Integer 0–15, The length of the active portion of the outgoing super frame, 15 = none*/ 00248 macSyncSymbolOffset = 0x5b, /*<Integer 0x000–0x100 (symbols) time stamp offset*/ 00249 macTimestampSupported = 0x5c, /*<Boolean*/ 00250 macTransactionPersistenceTime = 0x55, /*<Integer 0x0000–0xffff (unit periods)*/ 00251 macKeyTable = 0x71, /*<A table of KeyDescriptor entries, each containing keys and related information required for secured communications.*/ 00252 macKeyTableEntries = 0x72, /*<The number of entries in macKeyTable.*/ 00253 macDeviceTable = 0x73, /*<List of Descriptor entries, each indicating a remote device*/ 00254 macDeviceTableEntries = 0x74, /*<The number of entries in macDeviceTable.*/ 00255 macSecurityLevelTable = 0x75, /*<A table of SecurityLevelDescriptor entries*/ 00256 macSecurityLevelTableEntries = 0x76, /*<The number of entries in macSecurityLevelTable*/ 00257 macFrameCounter = 0x77, /*<The outgoing frame counter*/ 00258 macAutoRequestSecurityLevel = 0x78, /*<0x00–0x07 The security level used for automatic data requests.*/ 00259 macAutoRequestKeyIdMode = 0x79, /*< The key identifier mode used for automatic data requests.*/ 00260 macAutoRequestKeySource = 0x7a, /*<Key source for automatic data*/ 00261 macAutoRequestKeyIndex = 0x7b, /*<The index of the key used for automatic data*/ 00262 macDefaultKeySource = 0x7c, /*<Default key source*/ 00263 //NON standard extension 00264 macLoadBalancingBeaconTx = 0xfd, /*< Trig Beacon from load balance module periodic */ 00265 macLoadBalancingAcceptAnyBeacon = 0xfe, /*<Beacon accept state control from other network. Value size bool, data true=Enable, false=disable .*/ 00266 macThreadForceLongAddressForBeacon = 0xff /*<Thread standard force beacon source address for extended 64-bit*/ 00267 } mlme_attr_t; 00268 00269 /** 00270 * @brief struct mlme_beacon_pending_address_spec_t Pending address specification field 00271 * 00272 * See IEEE standard 802.15.4-2006 (figure 51) for more details 00273 */ 00274 typedef struct mlme_beacon_pending_address_spec_s{ 00275 unsigned short_address_count:3; /**< Number of short address count */ 00276 unsigned extended_address_count:3; /**< Number of extended address count */ 00277 }mlme_beacon_pending_address_spec_t; 00278 00279 /** 00280 * @brief struct mlme_beacon_gts_spec_t Format of GTS specification field 00281 * 00282 * See IEEE standard 802.15.4-2006 (figure 48) for more details 00283 */ 00284 typedef struct mlme_beacon_gts_spec_s{ 00285 unsigned description_count:3; /**< Number of GTS description count */ 00286 unsigned gts_permit:1; /**< 1= GTS request accepted 0= not accepted */ 00287 }mlme_beacon_gts_spec_t; 00288 00289 /** 00290 * @brief struct mlme_beacon_ind_t Beacon notify structure 00291 * 00292 * See IEEE standard 802.15.4-2006 (table 54) for more details 00293 */ 00294 typedef struct mlme_beacon_ind_s { 00295 uint8_t BSN; /**< Beacon sequence number */ 00296 mlme_pan_descriptor_t PANDescriptor; /**< Beacon parsed Pan description */ 00297 mlme_beacon_pending_address_spec_t PendAddrSpec; /**< Address pending field */ 00298 uint8_t *AddrList; /**< Address pending list */ 00299 uint16_t beacon_data_length; /**< Length of beacon payload */ 00300 uint8_t *beacon_data; /**< Pointer to beacon payload */ 00301 } mlme_beacon_ind_t; 00302 00303 /** 00304 * @brief struct mlme_scan_t Scan request structure 00305 * 00306 * See IEEE standard 802.15.4-2006 (table 67) for more details 00307 */ 00308 typedef struct mlme_scan_s { 00309 mac_scan_type_t ScanType; /**< ED=0, active=1, passive=2, orphan=3*/ 00310 channel_list_s ScanChannels; /**<bit field, low 27 bits used*/ 00311 uint8_t ScanDuration; /**<0-14, scan duration/channel*/ 00312 uint8_t ChannelPage; /**<0-31*/ 00313 mlme_security_t Key; /**< Security parameters for active scan process */ 00314 } mlme_scan_t; 00315 00316 /** 00317 * @brief struct mlme_set_t Set request structure 00318 * 00319 * See IEEE standard 802.15.4-2006 (table 70) for more details 00320 */ 00321 typedef struct mlme_set_s { 00322 mlme_attr_t attr; /**<PIB attribute for operation*/ 00323 uint8_t attr_index; /**< attribute index to to table (use only for PIB attributes which are tables)*/ 00324 const void *value_pointer; /**< Pointer to value*/ 00325 uint8_t value_size; /**< define data length in bytes behind pointer*/ 00326 } mlme_set_t; 00327 00328 /** 00329 * @brief struct mlme_get_t Get request structure 00330 * 00331 * See IEEE standard 802.15.4-2006 (table 56) for more details 00332 */ 00333 typedef struct mlme_get_s { 00334 mlme_attr_t attr; /**<PIB attribute for operation*/ 00335 uint8_t attr_index; /**< attribute index to to table (use only for PIB attributes which are tables)*/ 00336 } mlme_get_t; 00337 00338 /** 00339 * @brief struct mlme_get_conf_t Get confirm structure 00340 * 00341 * See IEEE standard 802.15.4-2006 (table 57) for more details 00342 */ 00343 typedef struct mlme_get_conf_s { 00344 uint8_t status; /**< status of operation*/ 00345 mlme_attr_t attr; /**<PIB attribute for operation*/ 00346 uint8_t attr_index; /**< attribute index to to table (valid only for PIB attributes which are tables)*/ 00347 void *value_pointer; /**< Pointer to data when status is MLME_SUCCESS */ 00348 uint8_t value_size; /**< define data length in bytes behind pointer*/ 00349 } mlme_get_conf_t; 00350 00351 /** 00352 * @brief struct mlme_set_conf_t Set confirm structure 00353 * 00354 * See IEEE standard 802.15.4-2006 (table 71) for more details 00355 */ 00356 typedef struct mlme_set_conf_s { 00357 uint8_t status; /**< status of operation*/ 00358 mlme_attr_t attr; /**<PIB attribute for operation*/ 00359 uint8_t attr_index; /**< attribute index to to table (valid only for PIB attributes which are tables)*/ 00360 } mlme_set_conf_t; 00361 00362 00363 #define MLME_MAC_RES_SIZE_MAX 16 /**< Mac scan response max supported list size */ 00364 00365 /** 00366 * @brief struct mlme_scan_conf_t Scan confirm structure 00367 * 00368 * See IEEE standard 802.15.4-2006 (table 68) for more details 00369 */ 00370 typedef struct mlme_scan_conf_s { 00371 uint8_t status; /**< status of operation*/ 00372 unsigned ScanType:2; /**< Finished Scan type*/ 00373 uint8_t ChannelPage; /**< Operated Channel Page*/ 00374 channel_list_s UnscannedChannels; /**< Channel mask for unscanned channels*/ 00375 uint8_t ResultListSize; /**< Result list size*/ 00376 uint8_t *ED_values; /**< Energy scan result types Check only when scan type is 0*/ 00377 mlme_pan_descriptor_t *PAN_values[MLME_MAC_RES_SIZE_MAX]; /**< List of scanned Pan description's*/ 00378 } mlme_scan_conf_t; 00379 00380 /** 00381 * @brief struct mlme_reset_t Reset request structure 00382 * 00383 * See IEEE standard 802.15.4-2006 (table 63) for more details 00384 */ 00385 typedef struct mlme_reset_s { 00386 bool SetDefaultPIB; /**< true= Set standard default values, false= Mac sub layer will be reset but it retain configured MAC PIB values */ 00387 } mlme_reset_t; 00388 00389 /** 00390 * @brief struct mlme_reset_conf_t Reset confirm structure 00391 * 00392 * See IEEE standard 802.15.4-2006 (table 64) for more details 00393 */ 00394 typedef struct mlme_reset_conf_s { 00395 uint8_t status; /**< Status of reset operation */ 00396 } mlme_reset_conf_t; 00397 00398 /** 00399 * @brief struct mlme_rx_enable_t Rx enable request structure (Not supported) 00400 * 00401 * See IEEE standard 802.15.4-2006 (table 65) for more details 00402 */ 00403 typedef struct mlme_rx_enable_s { 00404 bool DeferPermit; /**< This will be ignored at nonbeacon-enabled PAN*/ 00405 uint32_t RxOnTime; /**< This will be ignored at nonbeacon-enabled PAN*/ 00406 uint32_t RxOnDuration; /**< Number of symbols which receiver is enabled, 0 receiver is not disabled*/ 00407 } mlme_rx_enable_t; 00408 00409 /** 00410 * @brief struct mlme_rx_enable_conf_t Rx enable confirm structure (Not supported) 00411 * 00412 * See IEEE standard 802.15.4-2006 (table 66) for more details 00413 */ 00414 typedef struct mlme_rx_enable_conf_s { 00415 uint8_t status; /**< Status of operation */ 00416 } mlme_rx_enable_conf_t; 00417 00418 /** 00419 * @brief struct mlme_comm_status_t Comm status indication structure 00420 * 00421 * See IEEE standard 802.15.4-2006 (table 69) for more details 00422 */ 00423 typedef struct mlme_comm_status_s { 00424 uint16_t PANId; /**< Messages Pan-id */ 00425 unsigned SrcAddrMode:2; /**< source address mode: MAC_ADDR_MODE_NONE,MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00426 uint8_t SrcAddr[8]; /**< source address when mode is: MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00427 unsigned DstAddrMode:2; /**< destination address mode: MAC_ADDR_MODE_NONE,MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00428 uint8_t DstAddr[8]; /**< Destination address when mode is: MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00429 uint8_t status; /**< Communication status */ 00430 mlme_security_t Key; /**< Messages Security parameters */ 00431 } mlme_comm_status_t; 00432 00433 /** 00434 * @brief struct mlme_start_t Start request structure 00435 * 00436 * See IEEE standard 802.15.4-2006 (table 72) for more details 00437 */ 00438 typedef struct mlme_start_s { 00439 uint16_t PANId; /**< Pan-id */ 00440 uint8_t LogicalChannel; /**< Operated Logical channel */ 00441 uint8_t ChannelPage; /**< Operated Logical channel page */ 00442 uint32_t StartTime; /**< Start time, set 0 */ 00443 unsigned BeaconOrder:4; /**< Beacon order, set 15 */ 00444 unsigned SuperframeOrder:4; /**< Super frame order, set 15 */ 00445 bool PANCoordinator:1; /**< true= Enable beacon response for beacon request, false = disable beacon request responses */ 00446 bool BatteryLifeExtension:1; /**< Set false */ 00447 bool CoordRealignment:1; /**< Set false */ 00448 mlme_security_t CoordRealignKey; /**< Coordinator Realignment security parameter's (Valid only CoordRealignment = true)*/ 00449 mlme_security_t BeaconRealignKey; /**< Beacon realign security parameter's (Valid only CoordRealignment = true)*/ 00450 } mlme_start_t; 00451 00452 /** 00453 * @brief struct mlme_start_conf_t Start confirm structure (Currently not triggered yet) 00454 * 00455 * See IEEE standard 802.15.4-2006 (table 73) for more details 00456 */ 00457 typedef struct mlme_start_conf_s { 00458 uint8_t status; /**< Status for start confirmation */ 00459 } mlme_start_conf_t; 00460 00461 00462 /** 00463 * @brief struct mlme_sync_loss_s Synch loss indication 00464 * 00465 * Stack will trig this with FHSS enabled mac when synch to parent is lost 00466 * See IEEE standard 802.15.4-2006 (table 73) for more details 00467 */ 00468 typedef struct mlme_sync_loss_s { 00469 mlme_loss_reason_t LossReason; /**< Loss reason, BEACON_LOST with FHSS */ 00470 uint16_t PANId; /**< Pan-id */ 00471 uint8_t LogicalChannel; /**< Logical channel */ 00472 uint8_t ChannelPage; /**< Logical channel page */ 00473 mlme_security_t Key; /**< Security parameters */ 00474 } mlme_sync_loss_t; 00475 00476 /** 00477 * @brief struct mlme_poll_t Poll request structure 00478 * 00479 * See IEEE standard 802.15.4-2006 (table 76) for more details 00480 */ 00481 typedef struct mlme_poll_s { 00482 unsigned CoordAddrMode:2; /**< coordinator address mode:MAC_ADDR_MODE_16_BIT or MAC_ADDR_MODE_64_BIT */ 00483 uint16_t CoordPANId; /**< coordinator Pan-id to coordinator*/ 00484 uint8_t CoordAddress[8]; /**< coordinator address */ 00485 mlme_security_t Key; /**< Security parameters for Poll request */ 00486 } mlme_poll_t; 00487 00488 /** 00489 * @brief struct mlme_poll_conf_t Poll confirm structure 00490 * 00491 * See IEEE standard 802.15.4-2006 (table 77) for more details 00492 */ 00493 typedef struct mlme_poll_conf_s { 00494 uint8_t status; /**< Status of Poll operation */ 00495 } mlme_poll_conf_t; 00496 00497 #endif /* MLME_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
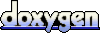