
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
mesh_interface_types.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __MESH_INTERFACE_TYPES_H__ 00018 #define __MESH_INTERFACE_TYPES_H__ 00019 00020 #ifdef __cplusplus 00021 extern "C" { 00022 #endif 00023 00024 /** 00025 * Mesh network types 00026 */ 00027 typedef enum { 00028 MESH_TYPE_6LOWPAN_ND = 0, 00029 MESH_TYPE_THREAD 00030 } mesh_network_type_t; 00031 00032 /* 00033 * Error status values returned by Mesh network API. 00034 */ 00035 typedef enum { 00036 MESH_ERROR_NONE = 0, /*<! No error */ 00037 MESH_ERROR_UNKNOWN, /*<! Unspecified error */ 00038 MESH_ERROR_MEMORY, /*<! Memory error */ 00039 MESH_ERROR_STATE, /*<! Illegal state */ 00040 MESH_ERROR_PARAM, /*<! Illegal parameter */ 00041 } mesh_error_t; 00042 00043 /* 00044 * Mesh network connection status codes returned in callback. 00045 */ 00046 typedef enum { 00047 MESH_CONNECTED = 0, /*<! connected to network */ 00048 MESH_DISCONNECTED, /*<! disconnected from network */ 00049 MESH_BOOTSTRAP_START_FAILED, /*<! error during bootstrap start */ 00050 MESH_BOOTSTRAP_FAILED /*<! error in bootstrap */ 00051 } mesh_connection_status_t; 00052 00053 /* 00054 * Mesh device types 00055 */ 00056 typedef enum { 00057 MESH_DEVICE_TYPE_THREAD_ROUTER = 0, /*<! Thread router */ 00058 MESH_DEVICE_TYPE_THREAD_SLEEPY_END_DEVICE, /*<! Thread Sleepy end device */ 00059 MESH_DEVICE_TYPE_THREAD_MINIMAL_END_DEVICE /*<! Thread minimal end device */ 00060 } mesh_device_type_t; 00061 00062 #ifdef __cplusplus 00063 } 00064 #endif 00065 00066 #endif /* __MESH_INTERFACE_TYPES_H__ */
Generated on Sun Jul 17 2022 08:25:28 by
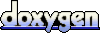