
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
memp_priv.h
Go to the documentation of this file.
00001 /** 00002 * @file 00003 * memory pools lwIP internal implementations (do not use in application code) 00004 */ 00005 00006 /* 00007 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00008 * All rights reserved. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. The name of the author may not be used to endorse or promote products 00019 * derived from this software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00022 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00023 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00024 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00026 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00027 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00028 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00029 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00030 * OF SUCH DAMAGE. 00031 * 00032 * This file is part of the lwIP TCP/IP stack. 00033 * 00034 * Author: Adam Dunkels <adam@sics.se> 00035 * 00036 */ 00037 00038 #ifndef LWIP_HDR_MEMP_PRIV_H 00039 #define LWIP_HDR_MEMP_PRIV_H 00040 00041 #include "lwip/opt.h" 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 #include "lwip/mem.h" 00048 00049 #if MEMP_OVERFLOW_CHECK 00050 /* if MEMP_OVERFLOW_CHECK is turned on, we reserve some bytes at the beginning 00051 * and at the end of each element, initialize them as 0xcd and check 00052 * them later. */ 00053 /* If MEMP_OVERFLOW_CHECK is >= 2, on every call to memp_malloc or memp_free, 00054 * every single element in each pool is checked! 00055 * This is VERY SLOW but also very helpful. */ 00056 /* MEMP_SANITY_REGION_BEFORE and MEMP_SANITY_REGION_AFTER can be overridden in 00057 * lwipopts.h to change the amount reserved for checking. */ 00058 #ifndef MEMP_SANITY_REGION_BEFORE 00059 #define MEMP_SANITY_REGION_BEFORE 16 00060 #endif /* MEMP_SANITY_REGION_BEFORE*/ 00061 #if MEMP_SANITY_REGION_BEFORE > 0 00062 #define MEMP_SANITY_REGION_BEFORE_ALIGNED LWIP_MEM_ALIGN_SIZE(MEMP_SANITY_REGION_BEFORE) 00063 #else 00064 #define MEMP_SANITY_REGION_BEFORE_ALIGNED 0 00065 #endif /* MEMP_SANITY_REGION_BEFORE*/ 00066 #ifndef MEMP_SANITY_REGION_AFTER 00067 #define MEMP_SANITY_REGION_AFTER 16 00068 #endif /* MEMP_SANITY_REGION_AFTER*/ 00069 #if MEMP_SANITY_REGION_AFTER > 0 00070 #define MEMP_SANITY_REGION_AFTER_ALIGNED LWIP_MEM_ALIGN_SIZE(MEMP_SANITY_REGION_AFTER) 00071 #else 00072 #define MEMP_SANITY_REGION_AFTER_ALIGNED 0 00073 #endif /* MEMP_SANITY_REGION_AFTER*/ 00074 00075 /* MEMP_SIZE: save space for struct memp and for sanity check */ 00076 #define MEMP_SIZE (LWIP_MEM_ALIGN_SIZE(sizeof(struct memp)) + MEMP_SANITY_REGION_BEFORE_ALIGNED) 00077 #define MEMP_ALIGN_SIZE(x) (LWIP_MEM_ALIGN_SIZE(x) + MEMP_SANITY_REGION_AFTER_ALIGNED) 00078 00079 #else /* MEMP_OVERFLOW_CHECK */ 00080 00081 /* No sanity checks 00082 * We don't need to preserve the struct memp while not allocated, so we 00083 * can save a little space and set MEMP_SIZE to 0. 00084 */ 00085 #define MEMP_SIZE 0 00086 #define MEMP_ALIGN_SIZE(x) (LWIP_MEM_ALIGN_SIZE(x)) 00087 00088 #endif /* MEMP_OVERFLOW_CHECK */ 00089 00090 #if !MEMP_MEM_MALLOC || MEMP_OVERFLOW_CHECK 00091 struct memp { 00092 struct memp *next; 00093 #if MEMP_OVERFLOW_CHECK 00094 const char *file; 00095 int line; 00096 #endif /* MEMP_OVERFLOW_CHECK */ 00097 }; 00098 #endif /* !MEMP_MEM_MALLOC || MEMP_OVERFLOW_CHECK */ 00099 00100 #if MEM_USE_POOLS && MEMP_USE_CUSTOM_POOLS 00101 /* Use a helper type to get the start and end of the user "memory pools" for mem_malloc */ 00102 typedef enum { 00103 /* Get the first (via: 00104 MEMP_POOL_HELPER_START = ((u8_t) 1*MEMP_POOL_A + 0*MEMP_POOL_B + 0*MEMP_POOL_C + 0)*/ 00105 MEMP_POOL_HELPER_FIRST = ((u8_t) 00106 #define LWIP_MEMPOOL(name,num,size,desc) 00107 #define LWIP_MALLOC_MEMPOOL_START 1 00108 #define LWIP_MALLOC_MEMPOOL(num, size) * MEMP_POOL_##size + 0 00109 #define LWIP_MALLOC_MEMPOOL_END 00110 #include "lwip/priv/memp_std.h" 00111 ) , 00112 /* Get the last (via: 00113 MEMP_POOL_HELPER_END = ((u8_t) 0 + MEMP_POOL_A*0 + MEMP_POOL_B*0 + MEMP_POOL_C*1) */ 00114 MEMP_POOL_HELPER_LAST = ((u8_t) 00115 #define LWIP_MEMPOOL(name,num,size,desc) 00116 #define LWIP_MALLOC_MEMPOOL_START 00117 #define LWIP_MALLOC_MEMPOOL(num, size) 0 + MEMP_POOL_##size * 00118 #define LWIP_MALLOC_MEMPOOL_END 1 00119 #include "lwip/priv/memp_std.h" 00120 ) 00121 } memp_pool_helper_t; 00122 00123 /* The actual start and stop values are here (cast them over) 00124 We use this helper type and these defines so we can avoid using const memp_t values */ 00125 #define MEMP_POOL_FIRST ((memp_t) MEMP_POOL_HELPER_FIRST) 00126 #define MEMP_POOL_LAST ((memp_t) MEMP_POOL_HELPER_LAST) 00127 #endif /* MEM_USE_POOLS && MEMP_USE_CUSTOM_POOLS */ 00128 00129 /** Memory pool descriptor */ 00130 struct memp_desc { 00131 #if defined(LWIP_DEBUG) || MEMP_OVERFLOW_CHECK || LWIP_STATS_DISPLAY 00132 /** Textual description */ 00133 const char *desc; 00134 #endif /* LWIP_DEBUG || MEMP_OVERFLOW_CHECK || LWIP_STATS_DISPLAY */ 00135 #if MEMP_STATS 00136 /** Statistics */ 00137 struct stats_mem *stats; 00138 #endif 00139 00140 /** Element size */ 00141 u16_t size; 00142 00143 #if !MEMP_MEM_MALLOC 00144 /** Number of elements */ 00145 u16_t num; 00146 00147 /** Base address */ 00148 u8_t *base; 00149 00150 /** First free element of each pool. Elements form a linked list. */ 00151 struct memp **tab; 00152 #endif /* MEMP_MEM_MALLOC */ 00153 }; 00154 00155 #if defined(LWIP_DEBUG) || MEMP_OVERFLOW_CHECK || LWIP_STATS_DISPLAY 00156 #define DECLARE_LWIP_MEMPOOL_DESC(desc) (desc), 00157 #else 00158 #define DECLARE_LWIP_MEMPOOL_DESC(desc) 00159 #endif 00160 00161 #if MEMP_STATS 00162 #define LWIP_MEMPOOL_DECLARE_STATS_INSTANCE(name) static struct stats_mem name; 00163 #define LWIP_MEMPOOL_DECLARE_STATS_REFERENCE(name) &name, 00164 #else 00165 #define LWIP_MEMPOOL_DECLARE_STATS_INSTANCE(name) 00166 #define LWIP_MEMPOOL_DECLARE_STATS_REFERENCE(name) 00167 #endif 00168 00169 void memp_init_pool(const struct memp_desc *desc); 00170 00171 #if MEMP_OVERFLOW_CHECK 00172 void *memp_malloc_pool_fn(const struct memp_desc* desc, const char* file, const int line); 00173 #define memp_malloc_pool(d) memp_malloc_pool_fn((d), __FILE__, __LINE__) 00174 #else 00175 void *memp_malloc_pool(const struct memp_desc *desc); 00176 #endif 00177 void memp_free_pool(const struct memp_desc* desc, void *mem); 00178 00179 #ifdef __cplusplus 00180 } 00181 #endif 00182 00183 #endif /* LWIP_HDR_MEMP_PRIV_H */
Generated on Sun Jul 17 2022 08:25:28 by
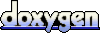