
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
config.h
00001 /** 00002 * \file config.h 00003 * 00004 * \brief Configuration options (set of defines) 00005 * 00006 * This set of compile-time options may be used to enable 00007 * or disable features selectively, and reduce the global 00008 * memory footprint. 00009 * 00010 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00011 * SPDX-License-Identifier: Apache-2.0 00012 * 00013 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00014 * not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at 00016 * 00017 * http://www.apache.org/licenses/LICENSE-2.0 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00021 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 * This file is part of mbed TLS (https://tls.mbed.org) 00026 */ 00027 00028 #ifndef MBEDTLS_CONFIG_H 00029 00030 #include "platform/inc/platform_mbed.h" 00031 00032 /* 00033 * Only use features that do not require an entropy source when 00034 * DEVICE_ENTROPY_SOURCE is not defined in mbed OS. 00035 */ 00036 #if !defined(MBEDTLS_ENTROPY_HARDWARE_ALT) && !defined(MBEDTLS_TEST_NULL_ENTROPY) 00037 #include "mbedtls/config-no-entropy.h" 00038 00039 #if defined(MBEDTLS_USER_CONFIG_FILE) 00040 #include MBEDTLS_USER_CONFIG_FILE 00041 #endif 00042 00043 #else 00044 #define MBEDTLS_CONFIG_H 00045 00046 #if defined(_MSC_VER) && !defined(_CRT_SECURE_NO_DEPRECATE) 00047 #define _CRT_SECURE_NO_DEPRECATE 1 00048 #endif 00049 00050 /** 00051 * \name SECTION: System support 00052 * 00053 * This section sets system specific settings. 00054 * \{ 00055 */ 00056 00057 /** 00058 * \def MBEDTLS_HAVE_ASM 00059 * 00060 * The compiler has support for asm(). 00061 * 00062 * Requires support for asm() in compiler. 00063 * 00064 * Used in: 00065 * library/timing.c 00066 * library/padlock.c 00067 * include/mbedtls/bn_mul.h 00068 * 00069 * Comment to disable the use of assembly code. 00070 */ 00071 #define MBEDTLS_HAVE_ASM 00072 00073 /** 00074 * \def MBEDTLS_NO_UDBL_DIVISION 00075 * 00076 * The platform lacks support for double-width integer division (64-bit 00077 * division on a 32-bit platform, 128-bit division on a 64-bit platform). 00078 * 00079 * Used in: 00080 * include/mbedtls/bignum.h 00081 * library/bignum.c 00082 * 00083 * The bignum code uses double-width division to speed up some operations. 00084 * Double-width division is often implemented in software that needs to 00085 * be linked with the program. The presence of a double-width integer 00086 * type is usually detected automatically through preprocessor macros, 00087 * but the automatic detection cannot know whether the code needs to 00088 * and can be linked with an implementation of division for that type. 00089 * By default division is assumed to be usable if the type is present. 00090 * Uncomment this option to prevent the use of double-width division. 00091 * 00092 * Note that division for the native integer type is always required. 00093 * Furthermore, a 64-bit type is always required even on a 32-bit 00094 * platform, but it need not support multiplication or division. In some 00095 * cases it is also desirable to disable some double-width operations. For 00096 * example, if double-width division is implemented in software, disabling 00097 * it can reduce code size in some embedded targets. 00098 */ 00099 //#define MBEDTLS_NO_UDBL_DIVISION 00100 00101 /** 00102 * \def MBEDTLS_HAVE_SSE2 00103 * 00104 * CPU supports SSE2 instruction set. 00105 * 00106 * Uncomment if the CPU supports SSE2 (IA-32 specific). 00107 */ 00108 //#define MBEDTLS_HAVE_SSE2 00109 00110 /** 00111 * \def MBEDTLS_HAVE_TIME 00112 * 00113 * System has time.h and time(). 00114 * The time does not need to be correct, only time differences are used, 00115 * by contrast with MBEDTLS_HAVE_TIME_DATE 00116 * 00117 * Defining MBEDTLS_HAVE_TIME allows you to specify MBEDTLS_PLATFORM_TIME_ALT, 00118 * MBEDTLS_PLATFORM_TIME_MACRO, MBEDTLS_PLATFORM_TIME_TYPE_MACRO and 00119 * MBEDTLS_PLATFORM_STD_TIME. 00120 * 00121 * Comment if your system does not support time functions 00122 */ 00123 #define MBEDTLS_HAVE_TIME 00124 00125 /** 00126 * \def MBEDTLS_HAVE_TIME_DATE 00127 * 00128 * System has time.h and time(), gmtime() and the clock is correct. 00129 * The time needs to be correct (not necesarily very accurate, but at least 00130 * the date should be correct). This is used to verify the validity period of 00131 * X.509 certificates. 00132 * 00133 * Comment if your system does not have a correct clock. 00134 */ 00135 //#define MBEDTLS_HAVE_TIME_DATE 00136 00137 /** 00138 * \def MBEDTLS_PLATFORM_MEMORY 00139 * 00140 * Enable the memory allocation layer. 00141 * 00142 * By default mbed TLS uses the system-provided calloc() and free(). 00143 * This allows different allocators (self-implemented or provided) to be 00144 * provided to the platform abstraction layer. 00145 * 00146 * Enabling MBEDTLS_PLATFORM_MEMORY without the 00147 * MBEDTLS_PLATFORM_{FREE,CALLOC}_MACROs will provide 00148 * "mbedtls_platform_set_calloc_free()" allowing you to set an alternative calloc() and 00149 * free() function pointer at runtime. 00150 * 00151 * Enabling MBEDTLS_PLATFORM_MEMORY and specifying 00152 * MBEDTLS_PLATFORM_{CALLOC,FREE}_MACROs will allow you to specify the 00153 * alternate function at compile time. 00154 * 00155 * Requires: MBEDTLS_PLATFORM_C 00156 * 00157 * Enable this layer to allow use of alternative memory allocators. 00158 */ 00159 //#define MBEDTLS_PLATFORM_MEMORY 00160 00161 /** 00162 * \def MBEDTLS_PLATFORM_NO_STD_FUNCTIONS 00163 * 00164 * Do not assign standard functions in the platform layer (e.g. calloc() to 00165 * MBEDTLS_PLATFORM_STD_CALLOC and printf() to MBEDTLS_PLATFORM_STD_PRINTF) 00166 * 00167 * This makes sure there are no linking errors on platforms that do not support 00168 * these functions. You will HAVE to provide alternatives, either at runtime 00169 * via the platform_set_xxx() functions or at compile time by setting 00170 * the MBEDTLS_PLATFORM_STD_XXX defines, or enabling a 00171 * MBEDTLS_PLATFORM_XXX_MACRO. 00172 * 00173 * Requires: MBEDTLS_PLATFORM_C 00174 * 00175 * Uncomment to prevent default assignment of standard functions in the 00176 * platform layer. 00177 */ 00178 //#define MBEDTLS_PLATFORM_NO_STD_FUNCTIONS 00179 00180 /** 00181 * \def MBEDTLS_PLATFORM_EXIT_ALT 00182 * 00183 * MBEDTLS_PLATFORM_XXX_ALT: Uncomment a macro to let mbed TLS support the 00184 * function in the platform abstraction layer. 00185 * 00186 * Example: In case you uncomment MBEDTLS_PLATFORM_PRINTF_ALT, mbed TLS will 00187 * provide a function "mbedtls_platform_set_printf()" that allows you to set an 00188 * alternative printf function pointer. 00189 * 00190 * All these define require MBEDTLS_PLATFORM_C to be defined! 00191 * 00192 * \note MBEDTLS_PLATFORM_SNPRINTF_ALT is required on Windows; 00193 * it will be enabled automatically by check_config.h 00194 * 00195 * \warning MBEDTLS_PLATFORM_XXX_ALT cannot be defined at the same time as 00196 * MBEDTLS_PLATFORM_XXX_MACRO! 00197 * 00198 * Requires: MBEDTLS_PLATFORM_TIME_ALT requires MBEDTLS_HAVE_TIME 00199 * 00200 * Uncomment a macro to enable alternate implementation of specific base 00201 * platform function 00202 */ 00203 //#define MBEDTLS_PLATFORM_EXIT_ALT 00204 //#define MBEDTLS_PLATFORM_TIME_ALT 00205 //#define MBEDTLS_PLATFORM_FPRINTF_ALT 00206 //#define MBEDTLS_PLATFORM_PRINTF_ALT 00207 //#define MBEDTLS_PLATFORM_SNPRINTF_ALT 00208 //#define MBEDTLS_PLATFORM_NV_SEED_ALT 00209 //#define MBEDTLS_PLATFORM_SETUP_TEARDOWN_ALT 00210 00211 /** 00212 * \def MBEDTLS_DEPRECATED_WARNING 00213 * 00214 * Mark deprecated functions so that they generate a warning if used. 00215 * Functions deprecated in one version will usually be removed in the next 00216 * version. You can enable this to help you prepare the transition to a new 00217 * major version by making sure your code is not using these functions. 00218 * 00219 * This only works with GCC and Clang. With other compilers, you may want to 00220 * use MBEDTLS_DEPRECATED_REMOVED 00221 * 00222 * Uncomment to get warnings on using deprecated functions. 00223 */ 00224 //#define MBEDTLS_DEPRECATED_WARNING 00225 00226 /** 00227 * \def MBEDTLS_DEPRECATED_REMOVED 00228 * 00229 * Remove deprecated functions so that they generate an error if used. 00230 * Functions deprecated in one version will usually be removed in the next 00231 * version. You can enable this to help you prepare the transition to a new 00232 * major version by making sure your code is not using these functions. 00233 * 00234 * Uncomment to get errors on using deprecated functions. 00235 */ 00236 //#define MBEDTLS_DEPRECATED_REMOVED 00237 00238 /* \} name SECTION: System support */ 00239 00240 /** 00241 * \name SECTION: mbed TLS feature support 00242 * 00243 * This section sets support for features that are or are not needed 00244 * within the modules that are enabled. 00245 * \{ 00246 */ 00247 00248 /** 00249 * \def MBEDTLS_TIMING_ALT 00250 * 00251 * Uncomment to provide your own alternate implementation for mbedtls_timing_hardclock(), 00252 * mbedtls_timing_get_timer(), mbedtls_set_alarm(), mbedtls_set/get_delay() 00253 * 00254 * Only works if you have MBEDTLS_TIMING_C enabled. 00255 * 00256 * You will need to provide a header "timing_alt.h" and an implementation at 00257 * compile time. 00258 */ 00259 //#define MBEDTLS_TIMING_ALT 00260 00261 /** 00262 * \def MBEDTLS_AES_ALT 00263 * 00264 * MBEDTLS__MODULE_NAME__ALT: Uncomment a macro to let mbed TLS use your 00265 * alternate core implementation of a symmetric crypto, an arithmetic or hash 00266 * module (e.g. platform specific assembly optimized implementations). Keep 00267 * in mind that the function prototypes should remain the same. 00268 * 00269 * This replaces the whole module. If you only want to replace one of the 00270 * functions, use one of the MBEDTLS__FUNCTION_NAME__ALT flags. 00271 * 00272 * Example: In case you uncomment MBEDTLS_AES_ALT, mbed TLS will no longer 00273 * provide the "struct mbedtls_aes_context" definition and omit the base 00274 * function declarations and implementations. "aes_alt.h" will be included from 00275 * "aes.h" to include the new function definitions. 00276 * 00277 * Uncomment a macro to enable alternate implementation of the corresponding 00278 * module. 00279 */ 00280 //#define MBEDTLS_AES_ALT 00281 //#define MBEDTLS_ARC4_ALT 00282 //#define MBEDTLS_BLOWFISH_ALT 00283 //#define MBEDTLS_CAMELLIA_ALT 00284 //#define MBEDTLS_DES_ALT 00285 //#define MBEDTLS_XTEA_ALT 00286 //#define MBEDTLS_MD2_ALT 00287 //#define MBEDTLS_MD4_ALT 00288 //#define MBEDTLS_MD5_ALT 00289 //#define MBEDTLS_RIPEMD160_ALT 00290 //#define MBEDTLS_SHA1_ALT 00291 //#define MBEDTLS_SHA256_ALT 00292 //#define MBEDTLS_SHA512_ALT 00293 /* 00294 * When replacing the elliptic curve module, pleace consider, that it is 00295 * implemented with two .c files: 00296 * - ecp.c 00297 * - ecp_curves.c 00298 * You can replace them very much like all the other MBEDTLS__MODULE_NAME__ALT 00299 * macros as described above. The only difference is that you have to make sure 00300 * that you provide functionality for both .c files. 00301 */ 00302 //#define MBEDTLS_ECP_ALT 00303 00304 /** 00305 * \def MBEDTLS_MD2_PROCESS_ALT 00306 * 00307 * MBEDTLS__FUNCTION_NAME__ALT: Uncomment a macro to let mbed TLS use you 00308 * alternate core implementation of symmetric crypto or hash function. Keep in 00309 * mind that function prototypes should remain the same. 00310 * 00311 * This replaces only one function. The header file from mbed TLS is still 00312 * used, in contrast to the MBEDTLS__MODULE_NAME__ALT flags. 00313 * 00314 * Example: In case you uncomment MBEDTLS_SHA256_PROCESS_ALT, mbed TLS will 00315 * no longer provide the mbedtls_sha1_process() function, but it will still provide 00316 * the other function (using your mbedtls_sha1_process() function) and the definition 00317 * of mbedtls_sha1_context, so your implementation of mbedtls_sha1_process must be compatible 00318 * with this definition. 00319 * 00320 * \note Because of a signature change, the core AES encryption and decryption routines are 00321 * currently named mbedtls_aes_internal_encrypt and mbedtls_aes_internal_decrypt, 00322 * respectively. When setting up alternative implementations, these functions should 00323 * be overriden, but the wrapper functions mbedtls_aes_decrypt and mbedtls_aes_encrypt 00324 * must stay untouched. 00325 * 00326 * \note If you use the AES_xxx_ALT macros, then is is recommended to also set 00327 * MBEDTLS_AES_ROM_TABLES in order to help the linker garbage-collect the AES 00328 * tables. 00329 * 00330 * Uncomment a macro to enable alternate implementation of the corresponding 00331 * function. 00332 */ 00333 //#define MBEDTLS_MD2_PROCESS_ALT 00334 //#define MBEDTLS_MD4_PROCESS_ALT 00335 //#define MBEDTLS_MD5_PROCESS_ALT 00336 //#define MBEDTLS_RIPEMD160_PROCESS_ALT 00337 //#define MBEDTLS_SHA1_PROCESS_ALT 00338 //#define MBEDTLS_SHA256_PROCESS_ALT 00339 //#define MBEDTLS_SHA512_PROCESS_ALT 00340 //#define MBEDTLS_DES_SETKEY_ALT 00341 //#define MBEDTLS_DES_CRYPT_ECB_ALT 00342 //#define MBEDTLS_DES3_CRYPT_ECB_ALT 00343 //#define MBEDTLS_AES_SETKEY_ENC_ALT 00344 //#define MBEDTLS_AES_SETKEY_DEC_ALT 00345 //#define MBEDTLS_AES_ENCRYPT_ALT 00346 //#define MBEDTLS_AES_DECRYPT_ALT 00347 00348 /** 00349 * \def MBEDTLS_ECP_INTERNAL_ALT 00350 * 00351 * Expose a part of the internal interface of the Elliptic Curve Point module. 00352 * 00353 * MBEDTLS_ECP__FUNCTION_NAME__ALT: Uncomment a macro to let mbed TLS use your 00354 * alternative core implementation of elliptic curve arithmetic. Keep in mind 00355 * that function prototypes should remain the same. 00356 * 00357 * This partially replaces one function. The header file from mbed TLS is still 00358 * used, in contrast to the MBEDTLS_ECP_ALT flag. The original implementation 00359 * is still present and it is used for group structures not supported by the 00360 * alternative. 00361 * 00362 * Any of these options become available by defining MBEDTLS_ECP_INTERNAL_ALT 00363 * and implementing the following functions: 00364 * unsigned char mbedtls_internal_ecp_grp_capable( 00365 * const mbedtls_ecp_group *grp ) 00366 * int mbedtls_internal_ecp_init( const mbedtls_ecp_group *grp ) 00367 * void mbedtls_internal_ecp_deinit( const mbedtls_ecp_group *grp ) 00368 * The mbedtls_internal_ecp_grp_capable function should return 1 if the 00369 * replacement functions implement arithmetic for the given group and 0 00370 * otherwise. 00371 * The functions mbedtls_internal_ecp_init and mbedtls_internal_ecp_deinit are 00372 * called before and after each point operation and provide an opportunity to 00373 * implement optimized set up and tear down instructions. 00374 * 00375 * Example: In case you uncomment MBEDTLS_ECP_INTERNAL_ALT and 00376 * MBEDTLS_ECP_DOUBLE_JAC_ALT, mbed TLS will still provide the ecp_double_jac 00377 * function, but will use your mbedtls_internal_ecp_double_jac if the group is 00378 * supported (your mbedtls_internal_ecp_grp_capable function returns 1 when 00379 * receives it as an argument). If the group is not supported then the original 00380 * implementation is used. The other functions and the definition of 00381 * mbedtls_ecp_group and mbedtls_ecp_point will not change, so your 00382 * implementation of mbedtls_internal_ecp_double_jac and 00383 * mbedtls_internal_ecp_grp_capable must be compatible with this definition. 00384 * 00385 * Uncomment a macro to enable alternate implementation of the corresponding 00386 * function. 00387 */ 00388 /* Required for all the functions in this section */ 00389 //#define MBEDTLS_ECP_INTERNAL_ALT 00390 /* Support for Weierstrass curves with Jacobi representation */ 00391 //#define MBEDTLS_ECP_RANDOMIZE_JAC_ALT 00392 //#define MBEDTLS_ECP_ADD_MIXED_ALT 00393 //#define MBEDTLS_ECP_DOUBLE_JAC_ALT 00394 //#define MBEDTLS_ECP_NORMALIZE_JAC_MANY_ALT 00395 //#define MBEDTLS_ECP_NORMALIZE_JAC_ALT 00396 /* Support for curves with Montgomery arithmetic */ 00397 //#define MBEDTLS_ECP_DOUBLE_ADD_MXZ_ALT 00398 //#define MBEDTLS_ECP_RANDOMIZE_MXZ_ALT 00399 //#define MBEDTLS_ECP_NORMALIZE_MXZ_ALT 00400 00401 /** 00402 * \def MBEDTLS_TEST_NULL_ENTROPY 00403 * 00404 * Enables testing and use of mbed TLS without any configured entropy sources. 00405 * This permits use of the library on platforms before an entropy source has 00406 * been integrated (see for example the MBEDTLS_ENTROPY_HARDWARE_ALT or the 00407 * MBEDTLS_ENTROPY_NV_SEED switches). 00408 * 00409 * WARNING! This switch MUST be disabled in production builds, and is suitable 00410 * only for development. 00411 * Enabling the switch negates any security provided by the library. 00412 * 00413 * Requires MBEDTLS_ENTROPY_C, MBEDTLS_NO_DEFAULT_ENTROPY_SOURCES 00414 * 00415 */ 00416 //#define MBEDTLS_TEST_NULL_ENTROPY 00417 00418 /** 00419 * \def MBEDTLS_ENTROPY_HARDWARE_ALT 00420 * 00421 * Uncomment this macro to let mbed TLS use your own implementation of a 00422 * hardware entropy collector. 00423 * 00424 * Your function must be called \c mbedtls_hardware_poll(), have the same 00425 * prototype as declared in entropy_poll.h, and accept NULL as first argument. 00426 * 00427 * Uncomment to use your own hardware entropy collector. 00428 */ 00429 //#define MBEDTLS_ENTROPY_HARDWARE_ALT 00430 00431 /** 00432 * \def MBEDTLS_AES_ROM_TABLES 00433 * 00434 * Store the AES tables in ROM. 00435 * 00436 * Uncomment this macro to store the AES tables in ROM. 00437 */ 00438 #define MBEDTLS_AES_ROM_TABLES 00439 00440 /** 00441 * \def MBEDTLS_CAMELLIA_SMALL_MEMORY 00442 * 00443 * Use less ROM for the Camellia implementation (saves about 768 bytes). 00444 * 00445 * Uncomment this macro to use less memory for Camellia. 00446 */ 00447 //#define MBEDTLS_CAMELLIA_SMALL_MEMORY 00448 00449 /** 00450 * \def MBEDTLS_CIPHER_MODE_CBC 00451 * 00452 * Enable Cipher Block Chaining mode (CBC) for symmetric ciphers. 00453 */ 00454 #define MBEDTLS_CIPHER_MODE_CBC 00455 00456 /** 00457 * \def MBEDTLS_CIPHER_MODE_CFB 00458 * 00459 * Enable Cipher Feedback mode (CFB) for symmetric ciphers. 00460 */ 00461 //#define MBEDTLS_CIPHER_MODE_CFB 00462 00463 /** 00464 * \def MBEDTLS_CIPHER_MODE_CTR 00465 * 00466 * Enable Counter Block Cipher mode (CTR) for symmetric ciphers. 00467 */ 00468 //#define MBEDTLS_CIPHER_MODE_CTR 00469 00470 /** 00471 * \def MBEDTLS_CIPHER_NULL_CIPHER 00472 * 00473 * Enable NULL cipher. 00474 * Warning: Only do so when you know what you are doing. This allows for 00475 * encryption or channels without any security! 00476 * 00477 * Requires MBEDTLS_ENABLE_WEAK_CIPHERSUITES as well to enable 00478 * the following ciphersuites: 00479 * MBEDTLS_TLS_ECDH_ECDSA_WITH_NULL_SHA 00480 * MBEDTLS_TLS_ECDH_RSA_WITH_NULL_SHA 00481 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_NULL_SHA 00482 * MBEDTLS_TLS_ECDHE_RSA_WITH_NULL_SHA 00483 * MBEDTLS_TLS_ECDHE_PSK_WITH_NULL_SHA384 00484 * MBEDTLS_TLS_ECDHE_PSK_WITH_NULL_SHA256 00485 * MBEDTLS_TLS_ECDHE_PSK_WITH_NULL_SHA 00486 * MBEDTLS_TLS_DHE_PSK_WITH_NULL_SHA384 00487 * MBEDTLS_TLS_DHE_PSK_WITH_NULL_SHA256 00488 * MBEDTLS_TLS_DHE_PSK_WITH_NULL_SHA 00489 * MBEDTLS_TLS_RSA_WITH_NULL_SHA256 00490 * MBEDTLS_TLS_RSA_WITH_NULL_SHA 00491 * MBEDTLS_TLS_RSA_WITH_NULL_MD5 00492 * MBEDTLS_TLS_RSA_PSK_WITH_NULL_SHA384 00493 * MBEDTLS_TLS_RSA_PSK_WITH_NULL_SHA256 00494 * MBEDTLS_TLS_RSA_PSK_WITH_NULL_SHA 00495 * MBEDTLS_TLS_PSK_WITH_NULL_SHA384 00496 * MBEDTLS_TLS_PSK_WITH_NULL_SHA256 00497 * MBEDTLS_TLS_PSK_WITH_NULL_SHA 00498 * 00499 * Uncomment this macro to enable the NULL cipher and ciphersuites 00500 */ 00501 //#define MBEDTLS_CIPHER_NULL_CIPHER 00502 00503 /** 00504 * \def MBEDTLS_CIPHER_PADDING_PKCS7 00505 * 00506 * MBEDTLS_CIPHER_PADDING_XXX: Uncomment or comment macros to add support for 00507 * specific padding modes in the cipher layer with cipher modes that support 00508 * padding (e.g. CBC) 00509 * 00510 * If you disable all padding modes, only full blocks can be used with CBC. 00511 * 00512 * Enable padding modes in the cipher layer. 00513 */ 00514 #define MBEDTLS_CIPHER_PADDING_PKCS7 00515 //#define MBEDTLS_CIPHER_PADDING_ONE_AND_ZEROS 00516 //#define MBEDTLS_CIPHER_PADDING_ZEROS_AND_LEN 00517 //#define MBEDTLS_CIPHER_PADDING_ZEROS 00518 00519 /** 00520 * \def MBEDTLS_ENABLE_WEAK_CIPHERSUITES 00521 * 00522 * Enable weak ciphersuites in SSL / TLS. 00523 * Warning: Only do so when you know what you are doing. This allows for 00524 * channels with virtually no security at all! 00525 * 00526 * This enables the following ciphersuites: 00527 * MBEDTLS_TLS_RSA_WITH_DES_CBC_SHA 00528 * MBEDTLS_TLS_DHE_RSA_WITH_DES_CBC_SHA 00529 * 00530 * Uncomment this macro to enable weak ciphersuites 00531 */ 00532 //#define MBEDTLS_ENABLE_WEAK_CIPHERSUITES 00533 00534 /** 00535 * \def MBEDTLS_REMOVE_ARC4_CIPHERSUITES 00536 * 00537 * Remove RC4 ciphersuites by default in SSL / TLS. 00538 * This flag removes the ciphersuites based on RC4 from the default list as 00539 * returned by mbedtls_ssl_list_ciphersuites(). However, it is still possible to 00540 * enable (some of) them with mbedtls_ssl_conf_ciphersuites() by including them 00541 * explicitly. 00542 * 00543 * Uncomment this macro to remove RC4 ciphersuites by default. 00544 */ 00545 #define MBEDTLS_REMOVE_ARC4_CIPHERSUITES 00546 00547 /** 00548 * \def MBEDTLS_ECP_DP_SECP192R1_ENABLED 00549 * 00550 * MBEDTLS_ECP_XXXX_ENABLED: Enables specific curves within the Elliptic Curve 00551 * module. By default all supported curves are enabled. 00552 * 00553 * Comment macros to disable the curve and functions for it 00554 */ 00555 //#define MBEDTLS_ECP_DP_SECP192R1_ENABLED 00556 //#define MBEDTLS_ECP_DP_SECP224R1_ENABLED 00557 #define MBEDTLS_ECP_DP_SECP256R1_ENABLED 00558 #define MBEDTLS_ECP_DP_SECP384R1_ENABLED 00559 //#define MBEDTLS_ECP_DP_SECP521R1_ENABLED 00560 //#define MBEDTLS_ECP_DP_SECP192K1_ENABLED 00561 //#define MBEDTLS_ECP_DP_SECP224K1_ENABLED 00562 //#define MBEDTLS_ECP_DP_SECP256K1_ENABLED 00563 //#define MBEDTLS_ECP_DP_BP256R1_ENABLED 00564 //#define MBEDTLS_ECP_DP_BP384R1_ENABLED 00565 //#define MBEDTLS_ECP_DP_BP512R1_ENABLED 00566 #define MBEDTLS_ECP_DP_CURVE25519_ENABLED 00567 00568 /** 00569 * \def MBEDTLS_ECP_NIST_OPTIM 00570 * 00571 * Enable specific 'modulo p' routines for each NIST prime. 00572 * Depending on the prime and architecture, makes operations 4 to 8 times 00573 * faster on the corresponding curve. 00574 * 00575 * Comment this macro to disable NIST curves optimisation. 00576 */ 00577 #define MBEDTLS_ECP_NIST_OPTIM 00578 00579 /** 00580 * \def MBEDTLS_ECDSA_DETERMINISTIC 00581 * 00582 * Enable deterministic ECDSA (RFC 6979). 00583 * Standard ECDSA is "fragile" in the sense that lack of entropy when signing 00584 * may result in a compromise of the long-term signing key. This is avoided by 00585 * the deterministic variant. 00586 * 00587 * Requires: MBEDTLS_HMAC_DRBG_C 00588 * 00589 * Comment this macro to disable deterministic ECDSA. 00590 */ 00591 #define MBEDTLS_ECDSA_DETERMINISTIC 00592 00593 /** 00594 * \def MBEDTLS_KEY_EXCHANGE_PSK_ENABLED 00595 * 00596 * Enable the PSK based ciphersuite modes in SSL / TLS. 00597 * 00598 * This enables the following ciphersuites (if other requisites are 00599 * enabled as well): 00600 * MBEDTLS_TLS_PSK_WITH_AES_256_GCM_SHA384 00601 * MBEDTLS_TLS_PSK_WITH_AES_256_CBC_SHA384 00602 * MBEDTLS_TLS_PSK_WITH_AES_256_CBC_SHA 00603 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_256_GCM_SHA384 00604 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_256_CBC_SHA384 00605 * MBEDTLS_TLS_PSK_WITH_AES_128_GCM_SHA256 00606 * MBEDTLS_TLS_PSK_WITH_AES_128_CBC_SHA256 00607 * MBEDTLS_TLS_PSK_WITH_AES_128_CBC_SHA 00608 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_128_GCM_SHA256 00609 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_128_CBC_SHA256 00610 * MBEDTLS_TLS_PSK_WITH_3DES_EDE_CBC_SHA 00611 * MBEDTLS_TLS_PSK_WITH_RC4_128_SHA 00612 */ 00613 #define MBEDTLS_KEY_EXCHANGE_PSK_ENABLED 00614 00615 /** 00616 * \def MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED 00617 * 00618 * Enable the DHE-PSK based ciphersuite modes in SSL / TLS. 00619 * 00620 * Requires: MBEDTLS_DHM_C 00621 * 00622 * This enables the following ciphersuites (if other requisites are 00623 * enabled as well): 00624 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_GCM_SHA384 00625 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_CBC_SHA384 00626 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_CBC_SHA 00627 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_256_GCM_SHA384 00628 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_256_CBC_SHA384 00629 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_GCM_SHA256 00630 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_CBC_SHA256 00631 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_CBC_SHA 00632 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_128_GCM_SHA256 00633 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_128_CBC_SHA256 00634 * MBEDTLS_TLS_DHE_PSK_WITH_3DES_EDE_CBC_SHA 00635 * MBEDTLS_TLS_DHE_PSK_WITH_RC4_128_SHA 00636 */ 00637 //#define MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED 00638 00639 /** 00640 * \def MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED 00641 * 00642 * Enable the ECDHE-PSK based ciphersuite modes in SSL / TLS. 00643 * 00644 * Requires: MBEDTLS_ECDH_C 00645 * 00646 * This enables the following ciphersuites (if other requisites are 00647 * enabled as well): 00648 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_256_CBC_SHA384 00649 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_256_CBC_SHA 00650 * MBEDTLS_TLS_ECDHE_PSK_WITH_CAMELLIA_256_CBC_SHA384 00651 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_128_CBC_SHA256 00652 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_128_CBC_SHA 00653 * MBEDTLS_TLS_ECDHE_PSK_WITH_CAMELLIA_128_CBC_SHA256 00654 * MBEDTLS_TLS_ECDHE_PSK_WITH_3DES_EDE_CBC_SHA 00655 * MBEDTLS_TLS_ECDHE_PSK_WITH_RC4_128_SHA 00656 */ 00657 #define MBEDTLS_KEY_EXCHANGE_ECDHE_PSK_ENABLED 00658 00659 /** 00660 * \def MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED 00661 * 00662 * Enable the RSA-PSK based ciphersuite modes in SSL / TLS. 00663 * 00664 * Requires: MBEDTLS_RSA_C, MBEDTLS_PKCS1_V15, 00665 * MBEDTLS_X509_CRT_PARSE_C 00666 * 00667 * This enables the following ciphersuites (if other requisites are 00668 * enabled as well): 00669 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_GCM_SHA384 00670 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_CBC_SHA384 00671 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_CBC_SHA 00672 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_256_GCM_SHA384 00673 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_256_CBC_SHA384 00674 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_GCM_SHA256 00675 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_CBC_SHA256 00676 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_CBC_SHA 00677 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_128_GCM_SHA256 00678 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_128_CBC_SHA256 00679 * MBEDTLS_TLS_RSA_PSK_WITH_3DES_EDE_CBC_SHA 00680 * MBEDTLS_TLS_RSA_PSK_WITH_RC4_128_SHA 00681 */ 00682 //#define MBEDTLS_KEY_EXCHANGE_RSA_PSK_ENABLED 00683 00684 /** 00685 * \def MBEDTLS_KEY_EXCHANGE_RSA_ENABLED 00686 * 00687 * Enable the RSA-only based ciphersuite modes in SSL / TLS. 00688 * 00689 * Requires: MBEDTLS_RSA_C, MBEDTLS_PKCS1_V15, 00690 * MBEDTLS_X509_CRT_PARSE_C 00691 * 00692 * This enables the following ciphersuites (if other requisites are 00693 * enabled as well): 00694 * MBEDTLS_TLS_RSA_WITH_AES_256_GCM_SHA384 00695 * MBEDTLS_TLS_RSA_WITH_AES_256_CBC_SHA256 00696 * MBEDTLS_TLS_RSA_WITH_AES_256_CBC_SHA 00697 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_GCM_SHA384 00698 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_CBC_SHA256 00699 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_CBC_SHA 00700 * MBEDTLS_TLS_RSA_WITH_AES_128_GCM_SHA256 00701 * MBEDTLS_TLS_RSA_WITH_AES_128_CBC_SHA256 00702 * MBEDTLS_TLS_RSA_WITH_AES_128_CBC_SHA 00703 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_GCM_SHA256 00704 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_CBC_SHA256 00705 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_CBC_SHA 00706 * MBEDTLS_TLS_RSA_WITH_3DES_EDE_CBC_SHA 00707 * MBEDTLS_TLS_RSA_WITH_RC4_128_SHA 00708 * MBEDTLS_TLS_RSA_WITH_RC4_128_MD5 00709 */ 00710 //#define MBEDTLS_KEY_EXCHANGE_RSA_ENABLED 00711 00712 /** 00713 * \def MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED 00714 * 00715 * Enable the DHE-RSA based ciphersuite modes in SSL / TLS. 00716 * 00717 * Requires: MBEDTLS_DHM_C, MBEDTLS_RSA_C, MBEDTLS_PKCS1_V15, 00718 * MBEDTLS_X509_CRT_PARSE_C 00719 * 00720 * This enables the following ciphersuites (if other requisites are 00721 * enabled as well): 00722 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_GCM_SHA384 00723 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_CBC_SHA256 00724 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_CBC_SHA 00725 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_GCM_SHA384 00726 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_CBC_SHA256 00727 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_CBC_SHA 00728 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_GCM_SHA256 00729 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_CBC_SHA256 00730 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_CBC_SHA 00731 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_GCM_SHA256 00732 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_CBC_SHA256 00733 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_CBC_SHA 00734 * MBEDTLS_TLS_DHE_RSA_WITH_3DES_EDE_CBC_SHA 00735 */ 00736 //#define MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED 00737 00738 /** 00739 * \def MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED 00740 * 00741 * Enable the ECDHE-RSA based ciphersuite modes in SSL / TLS. 00742 * 00743 * Requires: MBEDTLS_ECDH_C, MBEDTLS_RSA_C, MBEDTLS_PKCS1_V15, 00744 * MBEDTLS_X509_CRT_PARSE_C 00745 * 00746 * This enables the following ciphersuites (if other requisites are 00747 * enabled as well): 00748 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384 00749 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA384 00750 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA 00751 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_256_GCM_SHA384 00752 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_256_CBC_SHA384 00753 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256 00754 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256 00755 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA 00756 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_128_GCM_SHA256 00757 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_128_CBC_SHA256 00758 * MBEDTLS_TLS_ECDHE_RSA_WITH_3DES_EDE_CBC_SHA 00759 * MBEDTLS_TLS_ECDHE_RSA_WITH_RC4_128_SHA 00760 */ 00761 #define MBEDTLS_KEY_EXCHANGE_ECDHE_RSA_ENABLED 00762 00763 /** 00764 * \def MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED 00765 * 00766 * Enable the ECDHE-ECDSA based ciphersuite modes in SSL / TLS. 00767 * 00768 * Requires: MBEDTLS_ECDH_C, MBEDTLS_ECDSA_C, MBEDTLS_X509_CRT_PARSE_C, 00769 * 00770 * This enables the following ciphersuites (if other requisites are 00771 * enabled as well): 00772 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384 00773 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA384 00774 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA 00775 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_256_GCM_SHA384 00776 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_256_CBC_SHA384 00777 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256 00778 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256 00779 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA 00780 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_128_GCM_SHA256 00781 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_128_CBC_SHA256 00782 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_3DES_EDE_CBC_SHA 00783 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_RC4_128_SHA 00784 */ 00785 #define MBEDTLS_KEY_EXCHANGE_ECDHE_ECDSA_ENABLED 00786 00787 /** 00788 * \def MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED 00789 * 00790 * Enable the ECDH-ECDSA based ciphersuite modes in SSL / TLS. 00791 * 00792 * Requires: MBEDTLS_ECDH_C, MBEDTLS_X509_CRT_PARSE_C 00793 * 00794 * This enables the following ciphersuites (if other requisites are 00795 * enabled as well): 00796 * MBEDTLS_TLS_ECDH_ECDSA_WITH_RC4_128_SHA 00797 * MBEDTLS_TLS_ECDH_ECDSA_WITH_3DES_EDE_CBC_SHA 00798 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_CBC_SHA 00799 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_CBC_SHA 00800 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_CBC_SHA256 00801 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_CBC_SHA384 00802 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_GCM_SHA256 00803 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_GCM_SHA384 00804 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_128_CBC_SHA256 00805 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_256_CBC_SHA384 00806 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_128_GCM_SHA256 00807 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_256_GCM_SHA384 00808 */ 00809 //#define MBEDTLS_KEY_EXCHANGE_ECDH_ECDSA_ENABLED 00810 00811 /** 00812 * \def MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED 00813 * 00814 * Enable the ECDH-RSA based ciphersuite modes in SSL / TLS. 00815 * 00816 * Requires: MBEDTLS_ECDH_C, MBEDTLS_X509_CRT_PARSE_C 00817 * 00818 * This enables the following ciphersuites (if other requisites are 00819 * enabled as well): 00820 * MBEDTLS_TLS_ECDH_RSA_WITH_RC4_128_SHA 00821 * MBEDTLS_TLS_ECDH_RSA_WITH_3DES_EDE_CBC_SHA 00822 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_CBC_SHA 00823 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_CBC_SHA 00824 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_CBC_SHA256 00825 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_CBC_SHA384 00826 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_GCM_SHA256 00827 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_GCM_SHA384 00828 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_128_CBC_SHA256 00829 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_256_CBC_SHA384 00830 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_128_GCM_SHA256 00831 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_256_GCM_SHA384 00832 */ 00833 //#define MBEDTLS_KEY_EXCHANGE_ECDH_RSA_ENABLED 00834 00835 /** 00836 * \def MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED 00837 * 00838 * Enable the ECJPAKE based ciphersuite modes in SSL / TLS. 00839 * 00840 * \warning This is currently experimental. EC J-PAKE support is based on the 00841 * Thread v1.0.0 specification; incompatible changes to the specification 00842 * might still happen. For this reason, this is disabled by default. 00843 * 00844 * Requires: MBEDTLS_ECJPAKE_C 00845 * MBEDTLS_SHA256_C 00846 * MBEDTLS_ECP_DP_SECP256R1_ENABLED 00847 * 00848 * This enables the following ciphersuites (if other requisites are 00849 * enabled as well): 00850 * MBEDTLS_TLS_ECJPAKE_WITH_AES_128_CCM_8 00851 */ 00852 //#define MBEDTLS_KEY_EXCHANGE_ECJPAKE_ENABLED 00853 00854 /** 00855 * \def MBEDTLS_PK_PARSE_EC_EXTENDED 00856 * 00857 * Enhance support for reading EC keys using variants of SEC1 not allowed by 00858 * RFC 5915 and RFC 5480. 00859 * 00860 * Currently this means parsing the SpecifiedECDomain choice of EC 00861 * parameters (only known groups are supported, not arbitrary domains, to 00862 * avoid validation issues). 00863 * 00864 * Disable if you only need to support RFC 5915 + 5480 key formats. 00865 */ 00866 //#define MBEDTLS_PK_PARSE_EC_EXTENDED 00867 00868 /** 00869 * \def MBEDTLS_ERROR_STRERROR_DUMMY 00870 * 00871 * Enable a dummy error function to make use of mbedtls_strerror() in 00872 * third party libraries easier when MBEDTLS_ERROR_C is disabled 00873 * (no effect when MBEDTLS_ERROR_C is enabled). 00874 * 00875 * You can safely disable this if MBEDTLS_ERROR_C is enabled, or if you're 00876 * not using mbedtls_strerror() or error_strerror() in your application. 00877 * 00878 * Disable if you run into name conflicts and want to really remove the 00879 * mbedtls_strerror() 00880 */ 00881 #define MBEDTLS_ERROR_STRERROR_DUMMY 00882 00883 /** 00884 * \def MBEDTLS_GENPRIME 00885 * 00886 * Enable the prime-number generation code. 00887 * 00888 * Requires: MBEDTLS_BIGNUM_C 00889 */ 00890 //#define MBEDTLS_GENPRIME 00891 00892 /** 00893 * \def MBEDTLS_FS_IO 00894 * 00895 * Enable functions that use the filesystem. 00896 */ 00897 //#define MBEDTLS_FS_IO 00898 00899 /** 00900 * \def MBEDTLS_NO_DEFAULT_ENTROPY_SOURCES 00901 * 00902 * Do not add default entropy sources. These are the platform specific, 00903 * mbedtls_timing_hardclock and HAVEGE based poll functions. 00904 * 00905 * This is useful to have more control over the added entropy sources in an 00906 * application. 00907 * 00908 * Uncomment this macro to prevent loading of default entropy functions. 00909 */ 00910 //#define MBEDTLS_NO_DEFAULT_ENTROPY_SOURCES 00911 00912 /** 00913 * \def MBEDTLS_NO_PLATFORM_ENTROPY 00914 * 00915 * Do not use built-in platform entropy functions. 00916 * This is useful if your platform does not support 00917 * standards like the /dev/urandom or Windows CryptoAPI. 00918 * 00919 * Uncomment this macro to disable the built-in platform entropy functions. 00920 */ 00921 #define MBEDTLS_NO_PLATFORM_ENTROPY 00922 00923 /** 00924 * \def MBEDTLS_ENTROPY_FORCE_SHA256 00925 * 00926 * Force the entropy accumulator to use a SHA-256 accumulator instead of the 00927 * default SHA-512 based one (if both are available). 00928 * 00929 * Requires: MBEDTLS_SHA256_C 00930 * 00931 * On 32-bit systems SHA-256 can be much faster than SHA-512. Use this option 00932 * if you have performance concerns. 00933 * 00934 * This option is only useful if both MBEDTLS_SHA256_C and 00935 * MBEDTLS_SHA512_C are defined. Otherwise the available hash module is used. 00936 */ 00937 //#define MBEDTLS_ENTROPY_FORCE_SHA256 00938 00939 /** 00940 * \def MBEDTLS_ENTROPY_NV_SEED 00941 * 00942 * Enable the non-volatile (NV) seed file-based entropy source. 00943 * (Also enables the NV seed read/write functions in the platform layer) 00944 * 00945 * This is crucial (if not required) on systems that do not have a 00946 * cryptographic entropy source (in hardware or kernel) available. 00947 * 00948 * Requires: MBEDTLS_ENTROPY_C, MBEDTLS_PLATFORM_C 00949 * 00950 * \note The read/write functions that are used by the entropy source are 00951 * determined in the platform layer, and can be modified at runtime and/or 00952 * compile-time depending on the flags (MBEDTLS_PLATFORM_NV_SEED_*) used. 00953 * 00954 * \note If you use the default implementation functions that read a seedfile 00955 * with regular fopen(), please make sure you make a seedfile with the 00956 * proper name (defined in MBEDTLS_PLATFORM_STD_NV_SEED_FILE) and at 00957 * least MBEDTLS_ENTROPY_BLOCK_SIZE bytes in size that can be read from 00958 * and written to or you will get an entropy source error! The default 00959 * implementation will only use the first MBEDTLS_ENTROPY_BLOCK_SIZE 00960 * bytes from the file. 00961 * 00962 * \note The entropy collector will write to the seed file before entropy is 00963 * given to an external source, to update it. 00964 */ 00965 //#define MBEDTLS_ENTROPY_NV_SEED 00966 00967 /** 00968 * \def MBEDTLS_MEMORY_DEBUG 00969 * 00970 * Enable debugging of buffer allocator memory issues. Automatically prints 00971 * (to stderr) all (fatal) messages on memory allocation issues. Enables 00972 * function for 'debug output' of allocated memory. 00973 * 00974 * Requires: MBEDTLS_MEMORY_BUFFER_ALLOC_C 00975 * 00976 * Uncomment this macro to let the buffer allocator print out error messages. 00977 */ 00978 //#define MBEDTLS_MEMORY_DEBUG 00979 00980 /** 00981 * \def MBEDTLS_MEMORY_BACKTRACE 00982 * 00983 * Include backtrace information with each allocated block. 00984 * 00985 * Requires: MBEDTLS_MEMORY_BUFFER_ALLOC_C 00986 * GLIBC-compatible backtrace() an backtrace_symbols() support 00987 * 00988 * Uncomment this macro to include backtrace information 00989 */ 00990 //#define MBEDTLS_MEMORY_BACKTRACE 00991 00992 /** 00993 * \def MBEDTLS_PK_RSA_ALT_SUPPORT 00994 * 00995 * Support external private RSA keys (eg from a HSM) in the PK layer. 00996 * 00997 * Comment this macro to disable support for external private RSA keys. 00998 */ 00999 #define MBEDTLS_PK_RSA_ALT_SUPPORT 01000 01001 /** 01002 * \def MBEDTLS_PKCS1_V15 01003 * 01004 * Enable support for PKCS#1 v1.5 encoding. 01005 * 01006 * Requires: MBEDTLS_RSA_C 01007 * 01008 * This enables support for PKCS#1 v1.5 operations. 01009 */ 01010 #define MBEDTLS_PKCS1_V15 01011 01012 /** 01013 * \def MBEDTLS_PKCS1_V21 01014 * 01015 * Enable support for PKCS#1 v2.1 encoding. 01016 * 01017 * Requires: MBEDTLS_MD_C, MBEDTLS_RSA_C 01018 * 01019 * This enables support for RSAES-OAEP and RSASSA-PSS operations. 01020 */ 01021 #define MBEDTLS_PKCS1_V21 01022 01023 /** 01024 * \def MBEDTLS_RSA_NO_CRT 01025 * 01026 * Do not use the Chinese Remainder Theorem for the RSA private operation. 01027 * 01028 * Uncomment this macro to disable the use of CRT in RSA. 01029 * 01030 */ 01031 //#define MBEDTLS_RSA_NO_CRT 01032 01033 /** 01034 * \def MBEDTLS_SELF_TEST 01035 * 01036 * Enable the checkup functions (*_self_test). 01037 */ 01038 #define MBEDTLS_SELF_TEST 01039 01040 /** 01041 * \def MBEDTLS_SHA256_SMALLER 01042 * 01043 * Enable an implementation of SHA-256 that has lower ROM footprint but also 01044 * lower performance. 01045 * 01046 * The default implementation is meant to be a reasonnable compromise between 01047 * performance and size. This version optimizes more aggressively for size at 01048 * the expense of performance. Eg on Cortex-M4 it reduces the size of 01049 * mbedtls_sha256_process() from ~2KB to ~0.5KB for a performance hit of about 01050 * 30%. 01051 * 01052 * Uncomment to enable the smaller implementation of SHA256. 01053 */ 01054 //#define MBEDTLS_SHA256_SMALLER 01055 01056 /** 01057 * \def MBEDTLS_SSL_ALL_ALERT_MESSAGES 01058 * 01059 * Enable sending of alert messages in case of encountered errors as per RFC. 01060 * If you choose not to send the alert messages, mbed TLS can still communicate 01061 * with other servers, only debugging of failures is harder. 01062 * 01063 * The advantage of not sending alert messages, is that no information is given 01064 * about reasons for failures thus preventing adversaries of gaining intel. 01065 * 01066 * Enable sending of all alert messages 01067 */ 01068 #define MBEDTLS_SSL_ALL_ALERT_MESSAGES 01069 01070 /** 01071 * \def MBEDTLS_SSL_DEBUG_ALL 01072 * 01073 * Enable the debug messages in SSL module for all issues. 01074 * Debug messages have been disabled in some places to prevent timing 01075 * attacks due to (unbalanced) debugging function calls. 01076 * 01077 * If you need all error reporting you should enable this during debugging, 01078 * but remove this for production servers that should log as well. 01079 * 01080 * Uncomment this macro to report all debug messages on errors introducing 01081 * a timing side-channel. 01082 * 01083 */ 01084 //#define MBEDTLS_SSL_DEBUG_ALL 01085 01086 /** \def MBEDTLS_SSL_ENCRYPT_THEN_MAC 01087 * 01088 * Enable support for Encrypt-then-MAC, RFC 7366. 01089 * 01090 * This allows peers that both support it to use a more robust protection for 01091 * ciphersuites using CBC, providing deep resistance against timing attacks 01092 * on the padding or underlying cipher. 01093 * 01094 * This only affects CBC ciphersuites, and is useless if none is defined. 01095 * 01096 * Requires: MBEDTLS_SSL_PROTO_TLS1 or 01097 * MBEDTLS_SSL_PROTO_TLS1_1 or 01098 * MBEDTLS_SSL_PROTO_TLS1_2 01099 * 01100 * Comment this macro to disable support for Encrypt-then-MAC 01101 */ 01102 #define MBEDTLS_SSL_ENCRYPT_THEN_MAC 01103 01104 /** \def MBEDTLS_SSL_EXTENDED_MASTER_SECRET 01105 * 01106 * Enable support for Extended Master Secret, aka Session Hash 01107 * (draft-ietf-tls-session-hash-02). 01108 * 01109 * This was introduced as "the proper fix" to the Triple Handshake familiy of 01110 * attacks, but it is recommended to always use it (even if you disable 01111 * renegotiation), since it actually fixes a more fundamental issue in the 01112 * original SSL/TLS design, and has implications beyond Triple Handshake. 01113 * 01114 * Requires: MBEDTLS_SSL_PROTO_TLS1 or 01115 * MBEDTLS_SSL_PROTO_TLS1_1 or 01116 * MBEDTLS_SSL_PROTO_TLS1_2 01117 * 01118 * Comment this macro to disable support for Extended Master Secret. 01119 */ 01120 #define MBEDTLS_SSL_EXTENDED_MASTER_SECRET 01121 01122 /** 01123 * \def MBEDTLS_SSL_FALLBACK_SCSV 01124 * 01125 * Enable support for FALLBACK_SCSV (draft-ietf-tls-downgrade-scsv-00). 01126 * 01127 * For servers, it is recommended to always enable this, unless you support 01128 * only one version of TLS, or know for sure that none of your clients 01129 * implements a fallback strategy. 01130 * 01131 * For clients, you only need this if you're using a fallback strategy, which 01132 * is not recommended in the first place, unless you absolutely need it to 01133 * interoperate with buggy (version-intolerant) servers. 01134 * 01135 * Comment this macro to disable support for FALLBACK_SCSV 01136 */ 01137 //#define MBEDTLS_SSL_FALLBACK_SCSV 01138 01139 /** 01140 * \def MBEDTLS_SSL_HW_RECORD_ACCEL 01141 * 01142 * Enable hooking functions in SSL module for hardware acceleration of 01143 * individual records. 01144 * 01145 * Uncomment this macro to enable hooking functions. 01146 */ 01147 //#define MBEDTLS_SSL_HW_RECORD_ACCEL 01148 01149 /** 01150 * \def MBEDTLS_SSL_CBC_RECORD_SPLITTING 01151 * 01152 * Enable 1/n-1 record splitting for CBC mode in SSLv3 and TLS 1.0. 01153 * 01154 * This is a countermeasure to the BEAST attack, which also minimizes the risk 01155 * of interoperability issues compared to sending 0-length records. 01156 * 01157 * Comment this macro to disable 1/n-1 record splitting. 01158 */ 01159 //#define MBEDTLS_SSL_CBC_RECORD_SPLITTING 01160 01161 /** 01162 * \def MBEDTLS_SSL_RENEGOTIATION 01163 * 01164 * Disable support for TLS renegotiation. 01165 * 01166 * The two main uses of renegotiation are (1) refresh keys on long-lived 01167 * connections and (2) client authentication after the initial handshake. 01168 * If you don't need renegotiation, it's probably better to disable it, since 01169 * it has been associated with security issues in the past and is easy to 01170 * misuse/misunderstand. 01171 * 01172 * Comment this to disable support for renegotiation. 01173 */ 01174 #define MBEDTLS_SSL_RENEGOTIATION 01175 01176 /** 01177 * \def MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO 01178 * 01179 * Enable support for receiving and parsing SSLv2 Client Hello messages for the 01180 * SSL Server module (MBEDTLS_SSL_SRV_C). 01181 * 01182 * Uncomment this macro to enable support for SSLv2 Client Hello messages. 01183 */ 01184 //#define MBEDTLS_SSL_SRV_SUPPORT_SSLV2_CLIENT_HELLO 01185 01186 /** 01187 * \def MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE 01188 * 01189 * Pick the ciphersuite according to the client's preferences rather than ours 01190 * in the SSL Server module (MBEDTLS_SSL_SRV_C). 01191 * 01192 * Uncomment this macro to respect client's ciphersuite order 01193 */ 01194 //#define MBEDTLS_SSL_SRV_RESPECT_CLIENT_PREFERENCE 01195 01196 /** 01197 * \def MBEDTLS_SSL_MAX_FRAGMENT_LENGTH 01198 * 01199 * Enable support for RFC 6066 max_fragment_length extension in SSL. 01200 * 01201 * Comment this macro to disable support for the max_fragment_length extension 01202 */ 01203 #define MBEDTLS_SSL_MAX_FRAGMENT_LENGTH 01204 01205 /** 01206 * \def MBEDTLS_SSL_PROTO_SSL3 01207 * 01208 * Enable support for SSL 3.0. 01209 * 01210 * Requires: MBEDTLS_MD5_C 01211 * MBEDTLS_SHA1_C 01212 * 01213 * Comment this macro to disable support for SSL 3.0 01214 */ 01215 //#define MBEDTLS_SSL_PROTO_SSL3 01216 01217 /** 01218 * \def MBEDTLS_SSL_PROTO_TLS1 01219 * 01220 * Enable support for TLS 1.0. 01221 * 01222 * Requires: MBEDTLS_MD5_C 01223 * MBEDTLS_SHA1_C 01224 * 01225 * Comment this macro to disable support for TLS 1.0 01226 */ 01227 //#define MBEDTLS_SSL_PROTO_TLS1 01228 01229 /** 01230 * \def MBEDTLS_SSL_PROTO_TLS1_1 01231 * 01232 * Enable support for TLS 1.1 (and DTLS 1.0 if DTLS is enabled). 01233 * 01234 * Requires: MBEDTLS_MD5_C 01235 * MBEDTLS_SHA1_C 01236 * 01237 * Comment this macro to disable support for TLS 1.1 / DTLS 1.0 01238 */ 01239 //#define MBEDTLS_SSL_PROTO_TLS1_1 01240 01241 /** 01242 * \def MBEDTLS_SSL_PROTO_TLS1_2 01243 * 01244 * Enable support for TLS 1.2 (and DTLS 1.2 if DTLS is enabled). 01245 * 01246 * Requires: MBEDTLS_SHA1_C or MBEDTLS_SHA256_C or MBEDTLS_SHA512_C 01247 * (Depends on ciphersuites) 01248 * 01249 * Comment this macro to disable support for TLS 1.2 / DTLS 1.2 01250 */ 01251 #define MBEDTLS_SSL_PROTO_TLS1_2 01252 01253 /** 01254 * \def MBEDTLS_SSL_PROTO_DTLS 01255 * 01256 * Enable support for DTLS (all available versions). 01257 * 01258 * Enable this and MBEDTLS_SSL_PROTO_TLS1_1 to enable DTLS 1.0, 01259 * and/or this and MBEDTLS_SSL_PROTO_TLS1_2 to enable DTLS 1.2. 01260 * 01261 * Requires: MBEDTLS_SSL_PROTO_TLS1_1 01262 * or MBEDTLS_SSL_PROTO_TLS1_2 01263 * 01264 * Comment this macro to disable support for DTLS 01265 */ 01266 #define MBEDTLS_SSL_PROTO_DTLS 01267 01268 /** 01269 * \def MBEDTLS_SSL_ALPN 01270 * 01271 * Enable support for RFC 7301 Application Layer Protocol Negotiation. 01272 * 01273 * Comment this macro to disable support for ALPN. 01274 */ 01275 #define MBEDTLS_SSL_ALPN 01276 01277 /** 01278 * \def MBEDTLS_SSL_DTLS_ANTI_REPLAY 01279 * 01280 * Enable support for the anti-replay mechanism in DTLS. 01281 * 01282 * Requires: MBEDTLS_SSL_TLS_C 01283 * MBEDTLS_SSL_PROTO_DTLS 01284 * 01285 * \warning Disabling this is often a security risk! 01286 * See mbedtls_ssl_conf_dtls_anti_replay() for details. 01287 * 01288 * Comment this to disable anti-replay in DTLS. 01289 */ 01290 #define MBEDTLS_SSL_DTLS_ANTI_REPLAY 01291 01292 /** 01293 * \def MBEDTLS_SSL_DTLS_HELLO_VERIFY 01294 * 01295 * Enable support for HelloVerifyRequest on DTLS servers. 01296 * 01297 * This feature is highly recommended to prevent DTLS servers being used as 01298 * amplifiers in DoS attacks against other hosts. It should always be enabled 01299 * unless you know for sure amplification cannot be a problem in the 01300 * environment in which your server operates. 01301 * 01302 * \warning Disabling this can ba a security risk! (see above) 01303 * 01304 * Requires: MBEDTLS_SSL_PROTO_DTLS 01305 * 01306 * Comment this to disable support for HelloVerifyRequest. 01307 */ 01308 #define MBEDTLS_SSL_DTLS_HELLO_VERIFY 01309 01310 /** 01311 * \def MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE 01312 * 01313 * Enable server-side support for clients that reconnect from the same port. 01314 * 01315 * Some clients unexpectedly close the connection and try to reconnect using the 01316 * same source port. This needs special support from the server to handle the 01317 * new connection securely, as described in section 4.2.8 of RFC 6347. This 01318 * flag enables that support. 01319 * 01320 * Requires: MBEDTLS_SSL_DTLS_HELLO_VERIFY 01321 * 01322 * Comment this to disable support for clients reusing the source port. 01323 */ 01324 #define MBEDTLS_SSL_DTLS_CLIENT_PORT_REUSE 01325 01326 /** 01327 * \def MBEDTLS_SSL_DTLS_BADMAC_LIMIT 01328 * 01329 * Enable support for a limit of records with bad MAC. 01330 * 01331 * See mbedtls_ssl_conf_dtls_badmac_limit(). 01332 * 01333 * Requires: MBEDTLS_SSL_PROTO_DTLS 01334 */ 01335 #define MBEDTLS_SSL_DTLS_BADMAC_LIMIT 01336 01337 /** 01338 * \def MBEDTLS_SSL_SESSION_TICKETS 01339 * 01340 * Enable support for RFC 5077 session tickets in SSL. 01341 * Client-side, provides full support for session tickets (maintainance of a 01342 * session store remains the responsibility of the application, though). 01343 * Server-side, you also need to provide callbacks for writing and parsing 01344 * tickets, including authenticated encryption and key management. Example 01345 * callbacks are provided by MBEDTLS_SSL_TICKET_C. 01346 * 01347 * Comment this macro to disable support for SSL session tickets 01348 */ 01349 #define MBEDTLS_SSL_SESSION_TICKETS 01350 01351 /** 01352 * \def MBEDTLS_SSL_EXPORT_KEYS 01353 * 01354 * Enable support for exporting key block and master secret. 01355 * This is required for certain users of TLS, e.g. EAP-TLS. 01356 * 01357 * Comment this macro to disable support for key export 01358 */ 01359 #define MBEDTLS_SSL_EXPORT_KEYS 01360 01361 /** 01362 * \def MBEDTLS_SSL_SERVER_NAME_INDICATION 01363 * 01364 * Enable support for RFC 6066 server name indication (SNI) in SSL. 01365 * 01366 * Requires: MBEDTLS_X509_CRT_PARSE_C 01367 * 01368 * Comment this macro to disable support for server name indication in SSL 01369 */ 01370 #define MBEDTLS_SSL_SERVER_NAME_INDICATION 01371 01372 /** 01373 * \def MBEDTLS_SSL_TRUNCATED_HMAC 01374 * 01375 * Enable support for RFC 6066 truncated HMAC in SSL. 01376 * 01377 * Comment this macro to disable support for truncated HMAC in SSL 01378 */ 01379 //#define MBEDTLS_SSL_TRUNCATED_HMAC 01380 01381 /** 01382 * \def MBEDTLS_THREADING_ALT 01383 * 01384 * Provide your own alternate threading implementation. 01385 * 01386 * Requires: MBEDTLS_THREADING_C 01387 * 01388 * Uncomment this to allow your own alternate threading implementation. 01389 */ 01390 //#define MBEDTLS_THREADING_ALT 01391 01392 /** 01393 * \def MBEDTLS_THREADING_PTHREAD 01394 * 01395 * Enable the pthread wrapper layer for the threading layer. 01396 * 01397 * Requires: MBEDTLS_THREADING_C 01398 * 01399 * Uncomment this to enable pthread mutexes. 01400 */ 01401 //#define MBEDTLS_THREADING_PTHREAD 01402 01403 /** 01404 * \def MBEDTLS_VERSION_FEATURES 01405 * 01406 * Allow run-time checking of compile-time enabled features. Thus allowing users 01407 * to check at run-time if the library is for instance compiled with threading 01408 * support via mbedtls_version_check_feature(). 01409 * 01410 * Requires: MBEDTLS_VERSION_C 01411 * 01412 * Comment this to disable run-time checking and save ROM space 01413 */ 01414 #define MBEDTLS_VERSION_FEATURES 01415 01416 /** 01417 * \def MBEDTLS_X509_ALLOW_EXTENSIONS_NON_V3 01418 * 01419 * If set, the X509 parser will not break-off when parsing an X509 certificate 01420 * and encountering an extension in a v1 or v2 certificate. 01421 * 01422 * Uncomment to prevent an error. 01423 */ 01424 //#define MBEDTLS_X509_ALLOW_EXTENSIONS_NON_V3 01425 01426 /** 01427 * \def MBEDTLS_X509_ALLOW_UNSUPPORTED_CRITICAL_EXTENSION 01428 * 01429 * If set, the X509 parser will not break-off when parsing an X509 certificate 01430 * and encountering an unknown critical extension. 01431 * 01432 * \warning Depending on your PKI use, enabling this can be a security risk! 01433 * 01434 * Uncomment to prevent an error. 01435 */ 01436 //#define MBEDTLS_X509_ALLOW_UNSUPPORTED_CRITICAL_EXTENSION 01437 01438 /** 01439 * \def MBEDTLS_X509_CHECK_KEY_USAGE 01440 * 01441 * Enable verification of the keyUsage extension (CA and leaf certificates). 01442 * 01443 * Disabling this avoids problems with mis-issued and/or misused 01444 * (intermediate) CA and leaf certificates. 01445 * 01446 * \warning Depending on your PKI use, disabling this can be a security risk! 01447 * 01448 * Comment to skip keyUsage checking for both CA and leaf certificates. 01449 */ 01450 #define MBEDTLS_X509_CHECK_KEY_USAGE 01451 01452 /** 01453 * \def MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE 01454 * 01455 * Enable verification of the extendedKeyUsage extension (leaf certificates). 01456 * 01457 * Disabling this avoids problems with mis-issued and/or misused certificates. 01458 * 01459 * \warning Depending on your PKI use, disabling this can be a security risk! 01460 * 01461 * Comment to skip extendedKeyUsage checking for certificates. 01462 */ 01463 #define MBEDTLS_X509_CHECK_EXTENDED_KEY_USAGE 01464 01465 /** 01466 * \def MBEDTLS_X509_RSASSA_PSS_SUPPORT 01467 * 01468 * Enable parsing and verification of X.509 certificates, CRLs and CSRS 01469 * signed with RSASSA-PSS (aka PKCS#1 v2.1). 01470 * 01471 * Comment this macro to disallow using RSASSA-PSS in certificates. 01472 */ 01473 //#define MBEDTLS_X509_RSASSA_PSS_SUPPORT 01474 01475 /** 01476 * \def MBEDTLS_ZLIB_SUPPORT 01477 * 01478 * If set, the SSL/TLS module uses ZLIB to support compression and 01479 * decompression of packet data. 01480 * 01481 * \warning TLS-level compression MAY REDUCE SECURITY! See for example the 01482 * CRIME attack. Before enabling this option, you should examine with care if 01483 * CRIME or similar exploits may be a applicable to your use case. 01484 * 01485 * \note Currently compression can't be used with DTLS. 01486 * 01487 * Used in: library/ssl_tls.c 01488 * library/ssl_cli.c 01489 * library/ssl_srv.c 01490 * 01491 * This feature requires zlib library and headers to be present. 01492 * 01493 * Uncomment to enable use of ZLIB 01494 */ 01495 //#define MBEDTLS_ZLIB_SUPPORT 01496 /* \} name SECTION: mbed TLS feature support */ 01497 01498 /** 01499 * \name SECTION: mbed TLS modules 01500 * 01501 * This section enables or disables entire modules in mbed TLS 01502 * \{ 01503 */ 01504 01505 /** 01506 * \def MBEDTLS_AESNI_C 01507 * 01508 * Enable AES-NI support on x86-64. 01509 * 01510 * Module: library/aesni.c 01511 * Caller: library/aes.c 01512 * 01513 * Requires: MBEDTLS_HAVE_ASM 01514 * 01515 * This modules adds support for the AES-NI instructions on x86-64 01516 */ 01517 //#define MBEDTLS_AESNI_C 01518 01519 /** 01520 * \def MBEDTLS_AES_C 01521 * 01522 * Enable the AES block cipher. 01523 * 01524 * Module: library/aes.c 01525 * Caller: library/ssl_tls.c 01526 * library/pem.c 01527 * library/ctr_drbg.c 01528 * 01529 * This module enables the following ciphersuites (if other requisites are 01530 * enabled as well): 01531 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_CBC_SHA 01532 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_CBC_SHA 01533 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_CBC_SHA 01534 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_CBC_SHA 01535 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_CBC_SHA256 01536 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_CBC_SHA384 01537 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_CBC_SHA256 01538 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_CBC_SHA384 01539 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_128_GCM_SHA256 01540 * MBEDTLS_TLS_ECDH_ECDSA_WITH_AES_256_GCM_SHA384 01541 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_128_GCM_SHA256 01542 * MBEDTLS_TLS_ECDH_RSA_WITH_AES_256_GCM_SHA384 01543 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384 01544 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384 01545 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_GCM_SHA384 01546 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA384 01547 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA384 01548 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_CBC_SHA256 01549 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA 01550 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA 01551 * MBEDTLS_TLS_DHE_RSA_WITH_AES_256_CBC_SHA 01552 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256 01553 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256 01554 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_GCM_SHA256 01555 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA256 01556 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256 01557 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_CBC_SHA256 01558 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA 01559 * MBEDTLS_TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA 01560 * MBEDTLS_TLS_DHE_RSA_WITH_AES_128_CBC_SHA 01561 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_GCM_SHA384 01562 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_256_CBC_SHA384 01563 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_CBC_SHA384 01564 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_256_CBC_SHA 01565 * MBEDTLS_TLS_DHE_PSK_WITH_AES_256_CBC_SHA 01566 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_GCM_SHA256 01567 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_128_CBC_SHA256 01568 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_CBC_SHA256 01569 * MBEDTLS_TLS_ECDHE_PSK_WITH_AES_128_CBC_SHA 01570 * MBEDTLS_TLS_DHE_PSK_WITH_AES_128_CBC_SHA 01571 * MBEDTLS_TLS_RSA_WITH_AES_256_GCM_SHA384 01572 * MBEDTLS_TLS_RSA_WITH_AES_256_CBC_SHA256 01573 * MBEDTLS_TLS_RSA_WITH_AES_256_CBC_SHA 01574 * MBEDTLS_TLS_RSA_WITH_AES_128_GCM_SHA256 01575 * MBEDTLS_TLS_RSA_WITH_AES_128_CBC_SHA256 01576 * MBEDTLS_TLS_RSA_WITH_AES_128_CBC_SHA 01577 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_GCM_SHA384 01578 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_CBC_SHA384 01579 * MBEDTLS_TLS_RSA_PSK_WITH_AES_256_CBC_SHA 01580 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_GCM_SHA256 01581 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_CBC_SHA256 01582 * MBEDTLS_TLS_RSA_PSK_WITH_AES_128_CBC_SHA 01583 * MBEDTLS_TLS_PSK_WITH_AES_256_GCM_SHA384 01584 * MBEDTLS_TLS_PSK_WITH_AES_256_CBC_SHA384 01585 * MBEDTLS_TLS_PSK_WITH_AES_256_CBC_SHA 01586 * MBEDTLS_TLS_PSK_WITH_AES_128_GCM_SHA256 01587 * MBEDTLS_TLS_PSK_WITH_AES_128_CBC_SHA256 01588 * MBEDTLS_TLS_PSK_WITH_AES_128_CBC_SHA 01589 * 01590 * PEM_PARSE uses AES for decrypting encrypted keys. 01591 */ 01592 #define MBEDTLS_AES_C 01593 01594 /** 01595 * \def MBEDTLS_ARC4_C 01596 * 01597 * Enable the ARCFOUR stream cipher. 01598 * 01599 * Module: library/arc4.c 01600 * Caller: library/ssl_tls.c 01601 * 01602 * This module enables the following ciphersuites (if other requisites are 01603 * enabled as well): 01604 * MBEDTLS_TLS_ECDH_ECDSA_WITH_RC4_128_SHA 01605 * MBEDTLS_TLS_ECDH_RSA_WITH_RC4_128_SHA 01606 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_RC4_128_SHA 01607 * MBEDTLS_TLS_ECDHE_RSA_WITH_RC4_128_SHA 01608 * MBEDTLS_TLS_ECDHE_PSK_WITH_RC4_128_SHA 01609 * MBEDTLS_TLS_DHE_PSK_WITH_RC4_128_SHA 01610 * MBEDTLS_TLS_RSA_WITH_RC4_128_SHA 01611 * MBEDTLS_TLS_RSA_WITH_RC4_128_MD5 01612 * MBEDTLS_TLS_RSA_PSK_WITH_RC4_128_SHA 01613 * MBEDTLS_TLS_PSK_WITH_RC4_128_SHA 01614 */ 01615 //#define MBEDTLS_ARC4_C 01616 01617 /** 01618 * \def MBEDTLS_ASN1_PARSE_C 01619 * 01620 * Enable the generic ASN1 parser. 01621 * 01622 * Module: library/asn1.c 01623 * Caller: library/x509.c 01624 * library/dhm.c 01625 * library/pkcs12.c 01626 * library/pkcs5.c 01627 * library/pkparse.c 01628 */ 01629 #define MBEDTLS_ASN1_PARSE_C 01630 01631 /** 01632 * \def MBEDTLS_ASN1_WRITE_C 01633 * 01634 * Enable the generic ASN1 writer. 01635 * 01636 * Module: library/asn1write.c 01637 * Caller: library/ecdsa.c 01638 * library/pkwrite.c 01639 * library/x509_create.c 01640 * library/x509write_crt.c 01641 * library/x509write_csr.c 01642 */ 01643 #define MBEDTLS_ASN1_WRITE_C 01644 01645 /** 01646 * \def MBEDTLS_BASE64_C 01647 * 01648 * Enable the Base64 module. 01649 * 01650 * Module: library/base64.c 01651 * Caller: library/pem.c 01652 * 01653 * This module is required for PEM support (required by X.509). 01654 */ 01655 #define MBEDTLS_BASE64_C 01656 01657 /** 01658 * \def MBEDTLS_BIGNUM_C 01659 * 01660 * Enable the multi-precision integer library. 01661 * 01662 * Module: library/bignum.c 01663 * Caller: library/dhm.c 01664 * library/ecp.c 01665 * library/ecdsa.c 01666 * library/rsa.c 01667 * library/ssl_tls.c 01668 * 01669 * This module is required for RSA, DHM and ECC (ECDH, ECDSA) support. 01670 */ 01671 #define MBEDTLS_BIGNUM_C 01672 01673 /** 01674 * \def MBEDTLS_BLOWFISH_C 01675 * 01676 * Enable the Blowfish block cipher. 01677 * 01678 * Module: library/blowfish.c 01679 */ 01680 //#define MBEDTLS_BLOWFISH_C 01681 01682 /** 01683 * \def MBEDTLS_CAMELLIA_C 01684 * 01685 * Enable the Camellia block cipher. 01686 * 01687 * Module: library/camellia.c 01688 * Caller: library/ssl_tls.c 01689 * 01690 * This module enables the following ciphersuites (if other requisites are 01691 * enabled as well): 01692 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_128_CBC_SHA256 01693 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_256_CBC_SHA384 01694 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_128_CBC_SHA256 01695 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_256_CBC_SHA384 01696 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_128_GCM_SHA256 01697 * MBEDTLS_TLS_ECDH_ECDSA_WITH_CAMELLIA_256_GCM_SHA384 01698 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_128_GCM_SHA256 01699 * MBEDTLS_TLS_ECDH_RSA_WITH_CAMELLIA_256_GCM_SHA384 01700 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_256_GCM_SHA384 01701 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_256_GCM_SHA384 01702 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_GCM_SHA384 01703 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_256_CBC_SHA384 01704 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_256_CBC_SHA384 01705 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_CBC_SHA256 01706 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_256_CBC_SHA 01707 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_128_GCM_SHA256 01708 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_128_GCM_SHA256 01709 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_GCM_SHA256 01710 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_CAMELLIA_128_CBC_SHA256 01711 * MBEDTLS_TLS_ECDHE_RSA_WITH_CAMELLIA_128_CBC_SHA256 01712 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_CBC_SHA256 01713 * MBEDTLS_TLS_DHE_RSA_WITH_CAMELLIA_128_CBC_SHA 01714 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_256_GCM_SHA384 01715 * MBEDTLS_TLS_ECDHE_PSK_WITH_CAMELLIA_256_CBC_SHA384 01716 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_256_CBC_SHA384 01717 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_128_GCM_SHA256 01718 * MBEDTLS_TLS_DHE_PSK_WITH_CAMELLIA_128_CBC_SHA256 01719 * MBEDTLS_TLS_ECDHE_PSK_WITH_CAMELLIA_128_CBC_SHA256 01720 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_GCM_SHA384 01721 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_CBC_SHA256 01722 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_256_CBC_SHA 01723 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_GCM_SHA256 01724 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_CBC_SHA256 01725 * MBEDTLS_TLS_RSA_WITH_CAMELLIA_128_CBC_SHA 01726 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_256_GCM_SHA384 01727 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_256_CBC_SHA384 01728 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_128_GCM_SHA256 01729 * MBEDTLS_TLS_RSA_PSK_WITH_CAMELLIA_128_CBC_SHA256 01730 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_256_GCM_SHA384 01731 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_256_CBC_SHA384 01732 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_128_GCM_SHA256 01733 * MBEDTLS_TLS_PSK_WITH_CAMELLIA_128_CBC_SHA256 01734 */ 01735 //#define MBEDTLS_CAMELLIA_C 01736 01737 /** 01738 * \def MBEDTLS_CCM_C 01739 * 01740 * Enable the Counter with CBC-MAC (CCM) mode for 128-bit block cipher. 01741 * 01742 * Module: library/ccm.c 01743 * 01744 * Requires: MBEDTLS_AES_C or MBEDTLS_CAMELLIA_C 01745 * 01746 * This module enables the AES-CCM ciphersuites, if other requisites are 01747 * enabled as well. 01748 */ 01749 #define MBEDTLS_CCM_C 01750 01751 /** 01752 * \def MBEDTLS_CERTS_C 01753 * 01754 * Enable the test certificates. 01755 * 01756 * Module: library/certs.c 01757 * Caller: 01758 * 01759 * This module is used for testing (ssl_client/server). 01760 */ 01761 #define MBEDTLS_CERTS_C 01762 01763 /** 01764 * \def MBEDTLS_CIPHER_C 01765 * 01766 * Enable the generic cipher layer. 01767 * 01768 * Module: library/cipher.c 01769 * Caller: library/ssl_tls.c 01770 * 01771 * Uncomment to enable generic cipher wrappers. 01772 */ 01773 #define MBEDTLS_CIPHER_C 01774 01775 /** 01776 * \def MBEDTLS_CMAC_C 01777 * 01778 * Enable the CMAC (Cipher-based Message Authentication Code) mode for block 01779 * ciphers. 01780 * 01781 * Module: library/cmac.c 01782 * 01783 * Requires: MBEDTLS_AES_C or MBEDTLS_DES_C 01784 * 01785 */ 01786 //#define MBEDTLS_CMAC_C 01787 01788 /** 01789 * \def MBEDTLS_CTR_DRBG_C 01790 * 01791 * Enable the CTR_DRBG AES-256-based random generator. 01792 * 01793 * Module: library/ctr_drbg.c 01794 * Caller: 01795 * 01796 * Requires: MBEDTLS_AES_C 01797 * 01798 * This module provides the CTR_DRBG AES-256 random number generator. 01799 */ 01800 #define MBEDTLS_CTR_DRBG_C 01801 01802 /** 01803 * \def MBEDTLS_DEBUG_C 01804 * 01805 * Enable the debug functions. 01806 * 01807 * Module: library/debug.c 01808 * Caller: library/ssl_cli.c 01809 * library/ssl_srv.c 01810 * library/ssl_tls.c 01811 * 01812 * This module provides debugging functions. 01813 */ 01814 #define MBEDTLS_DEBUG_C 01815 01816 /** 01817 * \def MBEDTLS_DES_C 01818 * 01819 * Enable the DES block cipher. 01820 * 01821 * Module: library/des.c 01822 * Caller: library/pem.c 01823 * library/ssl_tls.c 01824 * 01825 * This module enables the following ciphersuites (if other requisites are 01826 * enabled as well): 01827 * MBEDTLS_TLS_ECDH_ECDSA_WITH_3DES_EDE_CBC_SHA 01828 * MBEDTLS_TLS_ECDH_RSA_WITH_3DES_EDE_CBC_SHA 01829 * MBEDTLS_TLS_ECDHE_ECDSA_WITH_3DES_EDE_CBC_SHA 01830 * MBEDTLS_TLS_ECDHE_RSA_WITH_3DES_EDE_CBC_SHA 01831 * MBEDTLS_TLS_DHE_RSA_WITH_3DES_EDE_CBC_SHA 01832 * MBEDTLS_TLS_ECDHE_PSK_WITH_3DES_EDE_CBC_SHA 01833 * MBEDTLS_TLS_DHE_PSK_WITH_3DES_EDE_CBC_SHA 01834 * MBEDTLS_TLS_RSA_WITH_3DES_EDE_CBC_SHA 01835 * MBEDTLS_TLS_RSA_PSK_WITH_3DES_EDE_CBC_SHA 01836 * MBEDTLS_TLS_PSK_WITH_3DES_EDE_CBC_SHA 01837 * 01838 * PEM_PARSE uses DES/3DES for decrypting encrypted keys. 01839 */ 01840 //#define MBEDTLS_DES_C 01841 01842 /** 01843 * \def MBEDTLS_DHM_C 01844 * 01845 * Enable the Diffie-Hellman-Merkle module. 01846 * 01847 * Module: library/dhm.c 01848 * Caller: library/ssl_cli.c 01849 * library/ssl_srv.c 01850 * 01851 * This module is used by the following key exchanges: 01852 * DHE-RSA, DHE-PSK 01853 */ 01854 //#define MBEDTLS_DHM_C 01855 01856 /** 01857 * \def MBEDTLS_ECDH_C 01858 * 01859 * Enable the elliptic curve Diffie-Hellman library. 01860 * 01861 * Module: library/ecdh.c 01862 * Caller: library/ssl_cli.c 01863 * library/ssl_srv.c 01864 * 01865 * This module is used by the following key exchanges: 01866 * ECDHE-ECDSA, ECDHE-RSA, DHE-PSK 01867 * 01868 * Requires: MBEDTLS_ECP_C 01869 */ 01870 #define MBEDTLS_ECDH_C 01871 01872 /** 01873 * \def MBEDTLS_ECDSA_C 01874 * 01875 * Enable the elliptic curve DSA library. 01876 * 01877 * Module: library/ecdsa.c 01878 * Caller: 01879 * 01880 * This module is used by the following key exchanges: 01881 * ECDHE-ECDSA 01882 * 01883 * Requires: MBEDTLS_ECP_C, MBEDTLS_ASN1_WRITE_C, MBEDTLS_ASN1_PARSE_C 01884 */ 01885 #define MBEDTLS_ECDSA_C 01886 01887 /** 01888 * \def MBEDTLS_ECJPAKE_C 01889 * 01890 * Enable the elliptic curve J-PAKE library. 01891 * 01892 * \warning This is currently experimental. EC J-PAKE support is based on the 01893 * Thread v1.0.0 specification; incompatible changes to the specification 01894 * might still happen. For this reason, this is disabled by default. 01895 * 01896 * Module: library/ecjpake.c 01897 * Caller: 01898 * 01899 * This module is used by the following key exchanges: 01900 * ECJPAKE 01901 * 01902 * Requires: MBEDTLS_ECP_C, MBEDTLS_MD_C 01903 */ 01904 //#define MBEDTLS_ECJPAKE_C 01905 01906 /** 01907 * \def MBEDTLS_ECP_C 01908 * 01909 * Enable the elliptic curve over GF(p) library. 01910 * 01911 * Module: library/ecp.c 01912 * Caller: library/ecdh.c 01913 * library/ecdsa.c 01914 * library/ecjpake.c 01915 * 01916 * Requires: MBEDTLS_BIGNUM_C and at least one MBEDTLS_ECP_DP_XXX_ENABLED 01917 */ 01918 #define MBEDTLS_ECP_C 01919 01920 /** 01921 * \def MBEDTLS_ENTROPY_C 01922 * 01923 * Enable the platform-specific entropy code. 01924 * 01925 * Module: library/entropy.c 01926 * Caller: 01927 * 01928 * Requires: MBEDTLS_SHA512_C or MBEDTLS_SHA256_C 01929 * 01930 * This module provides a generic entropy pool 01931 */ 01932 #define MBEDTLS_ENTROPY_C 01933 01934 /** 01935 * \def MBEDTLS_ERROR_C 01936 * 01937 * Enable error code to error string conversion. 01938 * 01939 * Module: library/error.c 01940 * Caller: 01941 * 01942 * This module enables mbedtls_strerror(). 01943 */ 01944 #define MBEDTLS_ERROR_C 01945 01946 /** 01947 * \def MBEDTLS_GCM_C 01948 * 01949 * Enable the Galois/Counter Mode (GCM) for AES. 01950 * 01951 * Module: library/gcm.c 01952 * 01953 * Requires: MBEDTLS_AES_C or MBEDTLS_CAMELLIA_C 01954 * 01955 * This module enables the AES-GCM and CAMELLIA-GCM ciphersuites, if other 01956 * requisites are enabled as well. 01957 */ 01958 #define MBEDTLS_GCM_C 01959 01960 /** 01961 * \def MBEDTLS_HAVEGE_C 01962 * 01963 * Enable the HAVEGE random generator. 01964 * 01965 * Warning: the HAVEGE random generator is not suitable for virtualized 01966 * environments 01967 * 01968 * Warning: the HAVEGE random generator is dependent on timing and specific 01969 * processor traits. It is therefore not advised to use HAVEGE as 01970 * your applications primary random generator or primary entropy pool 01971 * input. As a secondary input to your entropy pool, it IS able add 01972 * the (limited) extra entropy it provides. 01973 * 01974 * Module: library/havege.c 01975 * Caller: 01976 * 01977 * Requires: MBEDTLS_TIMING_C 01978 * 01979 * Uncomment to enable the HAVEGE random generator. 01980 */ 01981 //#define MBEDTLS_HAVEGE_C 01982 01983 /** 01984 * \def MBEDTLS_HMAC_DRBG_C 01985 * 01986 * Enable the HMAC_DRBG random generator. 01987 * 01988 * Module: library/hmac_drbg.c 01989 * Caller: 01990 * 01991 * Requires: MBEDTLS_MD_C 01992 * 01993 * Uncomment to enable the HMAC_DRBG random number geerator. 01994 */ 01995 #define MBEDTLS_HMAC_DRBG_C 01996 01997 /** 01998 * \def MBEDTLS_MD_C 01999 * 02000 * Enable the generic message digest layer. 02001 * 02002 * Module: library/md.c 02003 * Caller: 02004 * 02005 * Uncomment to enable generic message digest wrappers. 02006 */ 02007 #define MBEDTLS_MD_C 02008 02009 /** 02010 * \def MBEDTLS_MD2_C 02011 * 02012 * Enable the MD2 hash algorithm. 02013 * 02014 * Module: library/md2.c 02015 * Caller: 02016 * 02017 * Uncomment to enable support for (rare) MD2-signed X.509 certs. 02018 */ 02019 //#define MBEDTLS_MD2_C 02020 02021 /** 02022 * \def MBEDTLS_MD4_C 02023 * 02024 * Enable the MD4 hash algorithm. 02025 * 02026 * Module: library/md4.c 02027 * Caller: 02028 * 02029 * Uncomment to enable support for (rare) MD4-signed X.509 certs. 02030 */ 02031 //#define MBEDTLS_MD4_C 02032 02033 /** 02034 * \def MBEDTLS_MD5_C 02035 * 02036 * Enable the MD5 hash algorithm. 02037 * 02038 * Module: library/md5.c 02039 * Caller: library/md.c 02040 * library/pem.c 02041 * library/ssl_tls.c 02042 * 02043 * This module is required for SSL/TLS and X.509. 02044 * PEM_PARSE uses MD5 for decrypting encrypted keys. 02045 */ 02046 //#define MBEDTLS_MD5_C 02047 02048 /** 02049 * \def MBEDTLS_MEMORY_BUFFER_ALLOC_C 02050 * 02051 * Enable the buffer allocator implementation that makes use of a (stack) 02052 * based buffer to 'allocate' dynamic memory. (replaces calloc() and free() 02053 * calls) 02054 * 02055 * Module: library/memory_buffer_alloc.c 02056 * 02057 * Requires: MBEDTLS_PLATFORM_C 02058 * MBEDTLS_PLATFORM_MEMORY (to use it within mbed TLS) 02059 * 02060 * Enable this module to enable the buffer memory allocator. 02061 */ 02062 //#define MBEDTLS_MEMORY_BUFFER_ALLOC_C 02063 02064 /** 02065 * \def MBEDTLS_NET_C 02066 * 02067 * Enable the TCP and UDP over IPv6/IPv4 networking routines. 02068 * 02069 * \note This module only works on POSIX/Unix (including Linux, BSD and OS X) 02070 * and Windows. For other platforms, you'll want to disable it, and write your 02071 * own networking callbacks to be passed to \c mbedtls_ssl_set_bio(). 02072 * 02073 * \note See also our Knowledge Base article about porting to a new 02074 * environment: 02075 * https://tls.mbed.org/kb/how-to/how-do-i-port-mbed-tls-to-a-new-environment-OS 02076 * 02077 * Module: library/net_sockets.c 02078 * 02079 * This module provides networking routines. 02080 */ 02081 //#define MBEDTLS_NET_C 02082 02083 /** 02084 * \def MBEDTLS_OID_C 02085 * 02086 * Enable the OID database. 02087 * 02088 * Module: library/oid.c 02089 * Caller: library/asn1write.c 02090 * library/pkcs5.c 02091 * library/pkparse.c 02092 * library/pkwrite.c 02093 * library/rsa.c 02094 * library/x509.c 02095 * library/x509_create.c 02096 * library/x509_crl.c 02097 * library/x509_crt.c 02098 * library/x509_csr.c 02099 * library/x509write_crt.c 02100 * library/x509write_csr.c 02101 * 02102 * This modules translates between OIDs and internal values. 02103 */ 02104 #define MBEDTLS_OID_C 02105 02106 /** 02107 * \def MBEDTLS_PADLOCK_C 02108 * 02109 * Enable VIA Padlock support on x86. 02110 * 02111 * Module: library/padlock.c 02112 * Caller: library/aes.c 02113 * 02114 * Requires: MBEDTLS_HAVE_ASM 02115 * 02116 * This modules adds support for the VIA PadLock on x86. 02117 */ 02118 //#define MBEDTLS_PADLOCK_C 02119 02120 /** 02121 * \def MBEDTLS_PEM_PARSE_C 02122 * 02123 * Enable PEM decoding / parsing. 02124 * 02125 * Module: library/pem.c 02126 * Caller: library/dhm.c 02127 * library/pkparse.c 02128 * library/x509_crl.c 02129 * library/x509_crt.c 02130 * library/x509_csr.c 02131 * 02132 * Requires: MBEDTLS_BASE64_C 02133 * 02134 * This modules adds support for decoding / parsing PEM files. 02135 */ 02136 #define MBEDTLS_PEM_PARSE_C 02137 02138 /** 02139 * \def MBEDTLS_PEM_WRITE_C 02140 * 02141 * Enable PEM encoding / writing. 02142 * 02143 * Module: library/pem.c 02144 * Caller: library/pkwrite.c 02145 * library/x509write_crt.c 02146 * library/x509write_csr.c 02147 * 02148 * Requires: MBEDTLS_BASE64_C 02149 * 02150 * This modules adds support for encoding / writing PEM files. 02151 */ 02152 //#define MBEDTLS_PEM_WRITE_C 02153 02154 /** 02155 * \def MBEDTLS_PK_C 02156 * 02157 * Enable the generic public (asymetric) key layer. 02158 * 02159 * Module: library/pk.c 02160 * Caller: library/ssl_tls.c 02161 * library/ssl_cli.c 02162 * library/ssl_srv.c 02163 * 02164 * Requires: MBEDTLS_RSA_C or MBEDTLS_ECP_C 02165 * 02166 * Uncomment to enable generic public key wrappers. 02167 */ 02168 #define MBEDTLS_PK_C 02169 02170 /** 02171 * \def MBEDTLS_PK_PARSE_C 02172 * 02173 * Enable the generic public (asymetric) key parser. 02174 * 02175 * Module: library/pkparse.c 02176 * Caller: library/x509_crt.c 02177 * library/x509_csr.c 02178 * 02179 * Requires: MBEDTLS_PK_C 02180 * 02181 * Uncomment to enable generic public key parse functions. 02182 */ 02183 #define MBEDTLS_PK_PARSE_C 02184 02185 /** 02186 * \def MBEDTLS_PK_WRITE_C 02187 * 02188 * Enable the generic public (asymetric) key writer. 02189 * 02190 * Module: library/pkwrite.c 02191 * Caller: library/x509write.c 02192 * 02193 * Requires: MBEDTLS_PK_C 02194 * 02195 * Uncomment to enable generic public key write functions. 02196 */ 02197 #define MBEDTLS_PK_WRITE_C 02198 02199 /** 02200 * \def MBEDTLS_PKCS5_C 02201 * 02202 * Enable PKCS#5 functions. 02203 * 02204 * Module: library/pkcs5.c 02205 * 02206 * Requires: MBEDTLS_MD_C 02207 * 02208 * This module adds support for the PKCS#5 functions. 02209 */ 02210 //#define MBEDTLS_PKCS5_C 02211 02212 /** 02213 * \def MBEDTLS_PKCS11_C 02214 * 02215 * Enable wrapper for PKCS#11 smartcard support. 02216 * 02217 * Module: library/pkcs11.c 02218 * Caller: library/pk.c 02219 * 02220 * Requires: MBEDTLS_PK_C 02221 * 02222 * This module enables SSL/TLS PKCS #11 smartcard support. 02223 * Requires the presence of the PKCS#11 helper library (libpkcs11-helper) 02224 */ 02225 //#define MBEDTLS_PKCS11_C 02226 02227 /** 02228 * \def MBEDTLS_PKCS12_C 02229 * 02230 * Enable PKCS#12 PBE functions. 02231 * Adds algorithms for parsing PKCS#8 encrypted private keys 02232 * 02233 * Module: library/pkcs12.c 02234 * Caller: library/pkparse.c 02235 * 02236 * Requires: MBEDTLS_ASN1_PARSE_C, MBEDTLS_CIPHER_C, MBEDTLS_MD_C 02237 * Can use: MBEDTLS_ARC4_C 02238 * 02239 * This module enables PKCS#12 functions. 02240 */ 02241 //#define MBEDTLS_PKCS12_C 02242 02243 /** 02244 * \def MBEDTLS_PLATFORM_C 02245 * 02246 * Enable the platform abstraction layer that allows you to re-assign 02247 * functions like calloc(), free(), snprintf(), printf(), fprintf(), exit(). 02248 * 02249 * Enabling MBEDTLS_PLATFORM_C enables to use of MBEDTLS_PLATFORM_XXX_ALT 02250 * or MBEDTLS_PLATFORM_XXX_MACRO directives, allowing the functions mentioned 02251 * above to be specified at runtime or compile time respectively. 02252 * 02253 * \note This abstraction layer must be enabled on Windows (including MSYS2) 02254 * as other module rely on it for a fixed snprintf implementation. 02255 * 02256 * Module: library/platform.c 02257 * Caller: Most other .c files 02258 * 02259 * This module enables abstraction of common (libc) functions. 02260 */ 02261 #define MBEDTLS_PLATFORM_C 02262 02263 /** 02264 * \def MBEDTLS_RIPEMD160_C 02265 * 02266 * Enable the RIPEMD-160 hash algorithm. 02267 * 02268 * Module: library/ripemd160.c 02269 * Caller: library/md.c 02270 * 02271 */ 02272 //#define MBEDTLS_RIPEMD160_C 02273 02274 /** 02275 * \def MBEDTLS_RSA_C 02276 * 02277 * Enable the RSA public-key cryptosystem. 02278 * 02279 * Module: library/rsa.c 02280 * Caller: library/ssl_cli.c 02281 * library/ssl_srv.c 02282 * library/ssl_tls.c 02283 * library/x509.c 02284 * 02285 * This module is used by the following key exchanges: 02286 * RSA, DHE-RSA, ECDHE-RSA, RSA-PSK 02287 * 02288 * Requires: MBEDTLS_BIGNUM_C, MBEDTLS_OID_C 02289 */ 02290 #define MBEDTLS_RSA_C 02291 02292 /** 02293 * \def MBEDTLS_SHA1_C 02294 * 02295 * Enable the SHA1 cryptographic hash algorithm. 02296 * 02297 * Module: library/sha1.c 02298 * Caller: library/md.c 02299 * library/ssl_cli.c 02300 * library/ssl_srv.c 02301 * library/ssl_tls.c 02302 * library/x509write_crt.c 02303 * 02304 * This module is required for SSL/TLS up to version 1.1, for TLS 1.2 02305 * depending on the handshake parameters, and for SHA1-signed certificates. 02306 */ 02307 //#define MBEDTLS_SHA1_C 02308 02309 /** 02310 * \def MBEDTLS_SHA256_C 02311 * 02312 * Enable the SHA-224 and SHA-256 cryptographic hash algorithms. 02313 * 02314 * Module: library/sha256.c 02315 * Caller: library/entropy.c 02316 * library/md.c 02317 * library/ssl_cli.c 02318 * library/ssl_srv.c 02319 * library/ssl_tls.c 02320 * 02321 * This module adds support for SHA-224 and SHA-256. 02322 * This module is required for the SSL/TLS 1.2 PRF function. 02323 */ 02324 #define MBEDTLS_SHA256_C 02325 02326 /** 02327 * \def MBEDTLS_SHA512_C 02328 * 02329 * Enable the SHA-384 and SHA-512 cryptographic hash algorithms. 02330 * 02331 * Module: library/sha512.c 02332 * Caller: library/entropy.c 02333 * library/md.c 02334 * library/ssl_cli.c 02335 * library/ssl_srv.c 02336 * 02337 * This module adds support for SHA-384 and SHA-512. 02338 */ 02339 #define MBEDTLS_SHA512_C 02340 02341 /** 02342 * \def MBEDTLS_SSL_CACHE_C 02343 * 02344 * Enable simple SSL cache implementation. 02345 * 02346 * Module: library/ssl_cache.c 02347 * Caller: 02348 * 02349 * Requires: MBEDTLS_SSL_CACHE_C 02350 */ 02351 #define MBEDTLS_SSL_CACHE_C 02352 02353 /** 02354 * \def MBEDTLS_SSL_COOKIE_C 02355 * 02356 * Enable basic implementation of DTLS cookies for hello verification. 02357 * 02358 * Module: library/ssl_cookie.c 02359 * Caller: 02360 */ 02361 #define MBEDTLS_SSL_COOKIE_C 02362 02363 /** 02364 * \def MBEDTLS_SSL_TICKET_C 02365 * 02366 * Enable an implementation of TLS server-side callbacks for session tickets. 02367 * 02368 * Module: library/ssl_ticket.c 02369 * Caller: 02370 * 02371 * Requires: MBEDTLS_CIPHER_C 02372 */ 02373 #define MBEDTLS_SSL_TICKET_C 02374 02375 /** 02376 * \def MBEDTLS_SSL_CLI_C 02377 * 02378 * Enable the SSL/TLS client code. 02379 * 02380 * Module: library/ssl_cli.c 02381 * Caller: 02382 * 02383 * Requires: MBEDTLS_SSL_TLS_C 02384 * 02385 * This module is required for SSL/TLS client support. 02386 */ 02387 #define MBEDTLS_SSL_CLI_C 02388 02389 /** 02390 * \def MBEDTLS_SSL_SRV_C 02391 * 02392 * Enable the SSL/TLS server code. 02393 * 02394 * Module: library/ssl_srv.c 02395 * Caller: 02396 * 02397 * Requires: MBEDTLS_SSL_TLS_C 02398 * 02399 * This module is required for SSL/TLS server support. 02400 */ 02401 #define MBEDTLS_SSL_SRV_C 02402 02403 /** 02404 * \def MBEDTLS_SSL_TLS_C 02405 * 02406 * Enable the generic SSL/TLS code. 02407 * 02408 * Module: library/ssl_tls.c 02409 * Caller: library/ssl_cli.c 02410 * library/ssl_srv.c 02411 * 02412 * Requires: MBEDTLS_CIPHER_C, MBEDTLS_MD_C 02413 * and at least one of the MBEDTLS_SSL_PROTO_XXX defines 02414 * 02415 * This module is required for SSL/TLS. 02416 */ 02417 #define MBEDTLS_SSL_TLS_C 02418 02419 /** 02420 * \def MBEDTLS_THREADING_C 02421 * 02422 * Enable the threading abstraction layer. 02423 * By default mbed TLS assumes it is used in a non-threaded environment or that 02424 * contexts are not shared between threads. If you do intend to use contexts 02425 * between threads, you will need to enable this layer to prevent race 02426 * conditions. See also our Knowledge Base article about threading: 02427 * https://tls.mbed.org/kb/development/thread-safety-and-multi-threading 02428 * 02429 * Module: library/threading.c 02430 * 02431 * This allows different threading implementations (self-implemented or 02432 * provided). 02433 * 02434 * You will have to enable either MBEDTLS_THREADING_ALT or 02435 * MBEDTLS_THREADING_PTHREAD. 02436 * 02437 * Enable this layer to allow use of mutexes within mbed TLS 02438 */ 02439 //#define MBEDTLS_THREADING_C 02440 02441 /** 02442 * \def MBEDTLS_TIMING_C 02443 * 02444 * Enable the semi-portable timing interface. 02445 * 02446 * \note The provided implementation only works on POSIX/Unix (including Linux, 02447 * BSD and OS X) and Windows. On other platforms, you can either disable that 02448 * module and provide your own implementations of the callbacks needed by 02449 * \c mbedtls_ssl_set_timer_cb() for DTLS, or leave it enabled and provide 02450 * your own implementation of the whole module by setting 02451 * \c MBEDTLS_TIMING_ALT in the current file. 02452 * 02453 * \note See also our Knowledge Base article about porting to a new 02454 * environment: 02455 * https://tls.mbed.org/kb/how-to/how-do-i-port-mbed-tls-to-a-new-environment-OS 02456 * 02457 * Module: library/timing.c 02458 * Caller: library/havege.c 02459 * 02460 * This module is used by the HAVEGE random number generator. 02461 */ 02462 //#define MBEDTLS_TIMING_C 02463 02464 /** 02465 * \def MBEDTLS_VERSION_C 02466 * 02467 * Enable run-time version information. 02468 * 02469 * Module: library/version.c 02470 * 02471 * This module provides run-time version information. 02472 */ 02473 #define MBEDTLS_VERSION_C 02474 02475 /** 02476 * \def MBEDTLS_X509_USE_C 02477 * 02478 * Enable X.509 core for using certificates. 02479 * 02480 * Module: library/x509.c 02481 * Caller: library/x509_crl.c 02482 * library/x509_crt.c 02483 * library/x509_csr.c 02484 * 02485 * Requires: MBEDTLS_ASN1_PARSE_C, MBEDTLS_BIGNUM_C, MBEDTLS_OID_C, 02486 * MBEDTLS_PK_PARSE_C 02487 * 02488 * This module is required for the X.509 parsing modules. 02489 */ 02490 #define MBEDTLS_X509_USE_C 02491 02492 /** 02493 * \def MBEDTLS_X509_CRT_PARSE_C 02494 * 02495 * Enable X.509 certificate parsing. 02496 * 02497 * Module: library/x509_crt.c 02498 * Caller: library/ssl_cli.c 02499 * library/ssl_srv.c 02500 * library/ssl_tls.c 02501 * 02502 * Requires: MBEDTLS_X509_USE_C 02503 * 02504 * This module is required for X.509 certificate parsing. 02505 */ 02506 #define MBEDTLS_X509_CRT_PARSE_C 02507 02508 /** 02509 * \def MBEDTLS_X509_CRL_PARSE_C 02510 * 02511 * Enable X.509 CRL parsing. 02512 * 02513 * Module: library/x509_crl.c 02514 * Caller: library/x509_crt.c 02515 * 02516 * Requires: MBEDTLS_X509_USE_C 02517 * 02518 * This module is required for X.509 CRL parsing. 02519 */ 02520 #define MBEDTLS_X509_CRL_PARSE_C 02521 02522 /** 02523 * \def MBEDTLS_X509_CSR_PARSE_C 02524 * 02525 * Enable X.509 Certificate Signing Request (CSR) parsing. 02526 * 02527 * Module: library/x509_csr.c 02528 * Caller: library/x509_crt_write.c 02529 * 02530 * Requires: MBEDTLS_X509_USE_C 02531 * 02532 * This module is used for reading X.509 certificate request. 02533 */ 02534 //#define MBEDTLS_X509_CSR_PARSE_C 02535 02536 /** 02537 * \def MBEDTLS_X509_CREATE_C 02538 * 02539 * Enable X.509 core for creating certificates. 02540 * 02541 * Module: library/x509_create.c 02542 * 02543 * Requires: MBEDTLS_BIGNUM_C, MBEDTLS_OID_C, MBEDTLS_PK_WRITE_C 02544 * 02545 * This module is the basis for creating X.509 certificates and CSRs. 02546 */ 02547 //#define MBEDTLS_X509_CREATE_C 02548 02549 /** 02550 * \def MBEDTLS_X509_CRT_WRITE_C 02551 * 02552 * Enable creating X.509 certificates. 02553 * 02554 * Module: library/x509_crt_write.c 02555 * 02556 * Requires: MBEDTLS_X509_CREATE_C 02557 * 02558 * This module is required for X.509 certificate creation. 02559 */ 02560 //#define MBEDTLS_X509_CRT_WRITE_C 02561 02562 /** 02563 * \def MBEDTLS_X509_CSR_WRITE_C 02564 * 02565 * Enable creating X.509 Certificate Signing Requests (CSR). 02566 * 02567 * Module: library/x509_csr_write.c 02568 * 02569 * Requires: MBEDTLS_X509_CREATE_C 02570 * 02571 * This module is required for X.509 certificate request writing. 02572 */ 02573 //#define MBEDTLS_X509_CSR_WRITE_C 02574 02575 /** 02576 * \def MBEDTLS_XTEA_C 02577 * 02578 * Enable the XTEA block cipher. 02579 * 02580 * Module: library/xtea.c 02581 * Caller: 02582 */ 02583 //#define MBEDTLS_XTEA_C 02584 02585 /* \} name SECTION: mbed TLS modules */ 02586 02587 /** 02588 * \name SECTION: Module configuration options 02589 * 02590 * This section allows for the setting of module specific sizes and 02591 * configuration options. The default values are already present in the 02592 * relevant header files and should suffice for the regular use cases. 02593 * 02594 * Our advice is to enable options and change their values here 02595 * only if you have a good reason and know the consequences. 02596 * 02597 * Please check the respective header file for documentation on these 02598 * parameters (to prevent duplicate documentation). 02599 * \{ 02600 */ 02601 02602 /* MPI / BIGNUM options */ 02603 //#define MBEDTLS_MPI_WINDOW_SIZE 6 /**< Maximum windows size used. */ 02604 //#define MBEDTLS_MPI_MAX_SIZE 1024 /**< Maximum number of bytes for usable MPIs. */ 02605 02606 /* CTR_DRBG options */ 02607 //#define MBEDTLS_CTR_DRBG_ENTROPY_LEN 48 /**< Amount of entropy used per seed by default (48 with SHA-512, 32 with SHA-256) */ 02608 //#define MBEDTLS_CTR_DRBG_RESEED_INTERVAL 10000 /**< Interval before reseed is performed by default */ 02609 //#define MBEDTLS_CTR_DRBG_MAX_INPUT 256 /**< Maximum number of additional input bytes */ 02610 //#define MBEDTLS_CTR_DRBG_MAX_REQUEST 1024 /**< Maximum number of requested bytes per call */ 02611 //#define MBEDTLS_CTR_DRBG_MAX_SEED_INPUT 384 /**< Maximum size of (re)seed buffer */ 02612 02613 /* HMAC_DRBG options */ 02614 //#define MBEDTLS_HMAC_DRBG_RESEED_INTERVAL 10000 /**< Interval before reseed is performed by default */ 02615 //#define MBEDTLS_HMAC_DRBG_MAX_INPUT 256 /**< Maximum number of additional input bytes */ 02616 //#define MBEDTLS_HMAC_DRBG_MAX_REQUEST 1024 /**< Maximum number of requested bytes per call */ 02617 //#define MBEDTLS_HMAC_DRBG_MAX_SEED_INPUT 384 /**< Maximum size of (re)seed buffer */ 02618 02619 /* ECP options */ 02620 //#define MBEDTLS_ECP_MAX_BITS 521 /**< Maximum bit size of groups */ 02621 //#define MBEDTLS_ECP_WINDOW_SIZE 6 /**< Maximum window size used */ 02622 //#define MBEDTLS_ECP_FIXED_POINT_OPTIM 1 /**< Enable fixed-point speed-up */ 02623 02624 /* Entropy options */ 02625 //#define MBEDTLS_ENTROPY_MAX_SOURCES 20 /**< Maximum number of sources supported */ 02626 //#define MBEDTLS_ENTROPY_MAX_GATHER 128 /**< Maximum amount requested from entropy sources */ 02627 //#define MBEDTLS_ENTROPY_MIN_HARDWARE 32 /**< Default minimum number of bytes required for the hardware entropy source mbedtls_hardware_poll() before entropy is released */ 02628 02629 /* Memory buffer allocator options */ 02630 //#define MBEDTLS_MEMORY_ALIGN_MULTIPLE 4 /**< Align on multiples of this value */ 02631 02632 /* Platform options */ 02633 //#define MBEDTLS_PLATFORM_STD_MEM_HDR <stdlib.h> /**< Header to include if MBEDTLS_PLATFORM_NO_STD_FUNCTIONS is defined. Don't define if no header is needed. */ 02634 //#define MBEDTLS_PLATFORM_STD_CALLOC calloc /**< Default allocator to use, can be undefined */ 02635 //#define MBEDTLS_PLATFORM_STD_FREE free /**< Default free to use, can be undefined */ 02636 //#define MBEDTLS_PLATFORM_STD_EXIT exit /**< Default exit to use, can be undefined */ 02637 //#define MBEDTLS_PLATFORM_STD_TIME time /**< Default time to use, can be undefined. MBEDTLS_HAVE_TIME must be enabled */ 02638 //#define MBEDTLS_PLATFORM_STD_FPRINTF fprintf /**< Default fprintf to use, can be undefined */ 02639 //#define MBEDTLS_PLATFORM_STD_PRINTF printf /**< Default printf to use, can be undefined */ 02640 /* Note: your snprintf must correclty zero-terminate the buffer! */ 02641 //#define MBEDTLS_PLATFORM_STD_SNPRINTF snprintf /**< Default snprintf to use, can be undefined */ 02642 //#define MBEDTLS_PLATFORM_STD_EXIT_SUCCESS 0 /**< Default exit value to use, can be undefined */ 02643 //#define MBEDTLS_PLATFORM_STD_EXIT_FAILURE 1 /**< Default exit value to use, can be undefined */ 02644 //#define MBEDTLS_PLATFORM_STD_NV_SEED_READ mbedtls_platform_std_nv_seed_read /**< Default nv_seed_read function to use, can be undefined */ 02645 //#define MBEDTLS_PLATFORM_STD_NV_SEED_WRITE mbedtls_platform_std_nv_seed_write /**< Default nv_seed_write function to use, can be undefined */ 02646 //#define MBEDTLS_PLATFORM_STD_NV_SEED_FILE "seedfile" /**< Seed file to read/write with default implementation */ 02647 02648 /* To Use Function Macros MBEDTLS_PLATFORM_C must be enabled */ 02649 /* MBEDTLS_PLATFORM_XXX_MACRO and MBEDTLS_PLATFORM_XXX_ALT cannot both be defined */ 02650 //#define MBEDTLS_PLATFORM_CALLOC_MACRO calloc /**< Default allocator macro to use, can be undefined */ 02651 //#define MBEDTLS_PLATFORM_FREE_MACRO free /**< Default free macro to use, can be undefined */ 02652 //#define MBEDTLS_PLATFORM_EXIT_MACRO exit /**< Default exit macro to use, can be undefined */ 02653 //#define MBEDTLS_PLATFORM_TIME_MACRO time /**< Default time macro to use, can be undefined. MBEDTLS_HAVE_TIME must be enabled */ 02654 //#define MBEDTLS_PLATFORM_TIME_TYPE_MACRO time_t /**< Default time macro to use, can be undefined. MBEDTLS_HAVE_TIME must be enabled */ 02655 //#define MBEDTLS_PLATFORM_FPRINTF_MACRO fprintf /**< Default fprintf macro to use, can be undefined */ 02656 //#define MBEDTLS_PLATFORM_PRINTF_MACRO printf /**< Default printf macro to use, can be undefined */ 02657 /* Note: your snprintf must correclty zero-terminate the buffer! */ 02658 //#define MBEDTLS_PLATFORM_SNPRINTF_MACRO snprintf /**< Default snprintf macro to use, can be undefined */ 02659 //#define MBEDTLS_PLATFORM_NV_SEED_READ_MACRO mbedtls_platform_std_nv_seed_read /**< Default nv_seed_read function to use, can be undefined */ 02660 //#define MBEDTLS_PLATFORM_NV_SEED_WRITE_MACRO mbedtls_platform_std_nv_seed_write /**< Default nv_seed_write function to use, can be undefined */ 02661 02662 /* SSL Cache options */ 02663 //#define MBEDTLS_SSL_CACHE_DEFAULT_TIMEOUT 86400 /**< 1 day */ 02664 //#define MBEDTLS_SSL_CACHE_DEFAULT_MAX_ENTRIES 50 /**< Maximum entries in cache */ 02665 02666 /* SSL options */ 02667 //#define MBEDTLS_SSL_MAX_CONTENT_LEN 16384 /**< Maxium fragment length in bytes, determines the size of each of the two internal I/O buffers */ 02668 //#define MBEDTLS_SSL_DEFAULT_TICKET_LIFETIME 86400 /**< Lifetime of session tickets (if enabled) */ 02669 //#define MBEDTLS_PSK_MAX_LEN 32 /**< Max size of TLS pre-shared keys, in bytes (default 256 bits) */ 02670 //#define MBEDTLS_SSL_COOKIE_TIMEOUT 60 /**< Default expiration delay of DTLS cookies, in seconds if HAVE_TIME, or in number of cookies issued */ 02671 02672 /** 02673 * Complete list of ciphersuites to use, in order of preference. 02674 * 02675 * \warning No dependency checking is done on that field! This option can only 02676 * be used to restrict the set of available ciphersuites. It is your 02677 * responsibility to make sure the needed modules are active. 02678 * 02679 * Use this to save a few hundred bytes of ROM (default ordering of all 02680 * available ciphersuites) and a few to a few hundred bytes of RAM. 02681 * 02682 * The value below is only an example, not the default. 02683 */ 02684 //#define MBEDTLS_SSL_CIPHERSUITES MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_256_GCM_SHA384,MBEDTLS_TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256 02685 02686 /* X509 options */ 02687 //#define MBEDTLS_X509_MAX_INTERMEDIATE_CA 8 /**< Maximum number of intermediate CAs in a verification chain. */ 02688 //#define MBEDTLS_X509_MAX_FILE_PATH_LEN 512 /**< Maximum length of a path/filename string in bytes including the null terminator character ('\0'). */ 02689 02690 /** 02691 * Allow SHA-1 in the default TLS configuration for certificate signing. 02692 * Without this build-time option, SHA-1 support must be activated explicitly 02693 * through mbedtls_ssl_conf_cert_profile. Turning on this option is not 02694 * recommended because of it is possible to generte SHA-1 collisions, however 02695 * this may be safe for legacy infrastructure where additional controls apply. 02696 */ 02697 // #define MBEDTLS_TLS_DEFAULT_ALLOW_SHA1_IN_CERTIFICATES 02698 02699 /** 02700 * Allow SHA-1 in the default TLS configuration for TLS 1.2 handshake 02701 * signature and ciphersuite selection. Without this build-time option, SHA-1 02702 * support must be activated explicitly through mbedtls_ssl_conf_sig_hashes. 02703 * The use of SHA-1 in TLS <= 1.1 and in HMAC-SHA-1 is always allowed by 02704 * default. At the time of writing, there is no practical attack on the use 02705 * of SHA-1 in handshake signatures, hence this option is turned on by default 02706 * for compatibility with existing peers. 02707 */ 02708 #define MBEDTLS_TLS_DEFAULT_ALLOW_SHA1_IN_KEY_EXCHANGE 02709 02710 /* \} name SECTION: Customisation configuration options */ 02711 02712 /* Target and application specific configurations */ 02713 //#define YOTTA_CFG_MBEDTLS_TARGET_CONFIG_FILE "mbedtls/target_config.h" 02714 02715 #if defined(TARGET_LIKE_MBED) && defined(YOTTA_CFG_MBEDTLS_TARGET_CONFIG_FILE) 02716 #include YOTTA_CFG_MBEDTLS_TARGET_CONFIG_FILE 02717 #endif 02718 02719 /* 02720 * Allow user to override any previous default. 02721 * 02722 * Use two macro names for that, as: 02723 * - with yotta the prefix YOTTA_CFG_ is forced 02724 * - without yotta is looks weird to have a YOTTA prefix. 02725 */ 02726 #if defined(YOTTA_CFG_MBEDTLS_USER_CONFIG_FILE) 02727 #include YOTTA_CFG_MBEDTLS_USER_CONFIG_FILE 02728 #elif defined(MBEDTLS_USER_CONFIG_FILE) 02729 #include MBEDTLS_USER_CONFIG_FILE 02730 #endif 02731 02732 #include "check_config.h" 02733 02734 #endif /* !MBEDTLS_ENTROPY_HARDWARE_ALT && !MBEDTLS_TEST_NULL_ENTROPY */ 02735 02736 #if defined(MBEDTLS_TEST_NULL_ENTROPY) 02737 #warning "MBEDTLS_TEST_NULL_ENTROPY has been enabled. This " \ 02738 "configuration is not secure and is not suitable for production use" 02739 #endif 02740 02741 #if defined(MBEDTLS_SSL_TLS_C) && !defined(MBEDTLS_TEST_NULL_ENTROPY) && \ 02742 !defined(MBEDTLS_ENTROPY_HARDWARE_ALT) 02743 #error "No entropy source was found at build time, so TLS " \ 02744 "functionality is not available" 02745 #endif 02746 02747 #endif /* MBEDTLS_CONFIG_H */
Generated on Sun Jul 17 2022 08:25:21 by
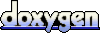