
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "test_env.h" 00003 #include "rtos.h" 00004 00005 #if defined(MBED_RTOS_SINGLE_THREAD) 00006 #error [NOT_SUPPORTED] test not supported 00007 #endif 00008 00009 #define THREAD_DELAY 50 00010 #define SIGNALS_TO_EMIT 100 00011 00012 /* 00013 * The stack size is defined in cmsis_os.h mainly dependent on the underlying toolchain and 00014 * the C standard library. For GCC, ARM_STD and IAR it is defined with a size of 2048 bytes 00015 * and for ARM_MICRO 512. Because of reduce RAM size some targets need a reduced stacksize. 00016 */ 00017 #if defined(TARGET_STM32F334R8) && defined(TOOLCHAIN_IAR) 00018 #define STACK_SIZE DEFAULT_STACK_SIZE/4 00019 #elif (defined(TARGET_STM32F070RB) || defined(TARGET_STM32F072RB)) 00020 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00021 #elif defined(TARGET_STM32F302R8) && defined(TOOLCHAIN_IAR) 00022 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00023 #elif defined(TARGET_STM32F303K8) && defined(TOOLCHAIN_IAR) 00024 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00025 #elif defined(TARGET_STM32F334C8) 00026 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00027 #elif defined(TARGET_STM32L073RZ) 00028 #define STACK_SIZE DEFAULT_STACK_SIZE/2 00029 #elif (defined(TARGET_EFM32HG_STK3400)) && !defined(TOOLCHAIN_ARM_MICRO) 00030 #define STACK_SIZE 512 00031 #elif (defined(TARGET_EFM32LG_STK3600) || defined(TARGET_EFM32WG_STK3800) || defined(TARGET_EFM32PG_STK3401)) && !defined(TOOLCHAIN_ARM_MICRO) 00032 #define STACK_SIZE 768 00033 #elif (defined(TARGET_EFM32GG_STK3700)) && !defined(TOOLCHAIN_ARM_MICRO) 00034 #define STACK_SIZE 1536 00035 #elif defined(TARGET_MCU_NRF51822) 00036 #define STACK_SIZE 768 00037 #else 00038 #define STACK_SIZE DEFAULT_STACK_SIZE 00039 #endif 00040 00041 void print_char(char c = '*') { 00042 printf("%c", c); 00043 fflush(stdout); 00044 } 00045 00046 Mutex stdio_mutex; 00047 DigitalOut led(LED1); 00048 00049 volatile int change_counter = 0; 00050 volatile bool changing_counter = false; 00051 volatile bool mutex_defect = false; 00052 00053 bool manipulate_protected_zone(const int thread_delay) { 00054 bool result = true; 00055 00056 stdio_mutex.lock(); // LOCK 00057 if (changing_counter == true) { 00058 // 'e' stands for error. If changing_counter is true access is not exclusively 00059 print_char('e'); 00060 result = false; 00061 mutex_defect = true; 00062 } 00063 changing_counter = true; 00064 00065 // Some action on protected 00066 led = !led; 00067 change_counter++; 00068 print_char('.'); 00069 Thread::wait(thread_delay); 00070 00071 changing_counter = false; 00072 stdio_mutex.unlock(); // UNLOCK 00073 return result; 00074 } 00075 00076 void test_thread(void const *args) { 00077 const int thread_delay = int(args); 00078 while (true) { 00079 manipulate_protected_zone(thread_delay); 00080 } 00081 } 00082 00083 int main() { 00084 MBED_HOSTTEST_TIMEOUT(20); 00085 MBED_HOSTTEST_SELECT(default); 00086 MBED_HOSTTEST_DESCRIPTION(Mutex resource lock); 00087 MBED_HOSTTEST_START("RTOS_2"); 00088 00089 const int t1_delay = THREAD_DELAY * 1; 00090 const int t2_delay = THREAD_DELAY * 2; 00091 const int t3_delay = THREAD_DELAY * 3; 00092 Thread t2(test_thread, (void *)t2_delay, osPriorityNormal, STACK_SIZE); 00093 Thread t3(test_thread, (void *)t3_delay, osPriorityNormal, STACK_SIZE); 00094 00095 while (true) { 00096 // Thread 1 action 00097 Thread::wait(t1_delay); 00098 manipulate_protected_zone(t1_delay); 00099 if (change_counter >= SIGNALS_TO_EMIT or mutex_defect == true) { 00100 t2.terminate(); 00101 t3.terminate(); 00102 break; 00103 } 00104 } 00105 00106 fflush(stdout); 00107 MBED_HOSTTEST_RESULT(!mutex_defect); 00108 return 0; 00109 }
Generated on Sun Jul 17 2022 08:25:26 by
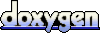