
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "test_env.h" 00003 00004 void print_char(char c = '*') 00005 { 00006 printf("%c", c); 00007 fflush(stdout); 00008 } 00009 00010 Ticker flipper_1; 00011 DigitalOut led1(LED1); 00012 00013 void flip_1() { 00014 static int led1_state = 0; 00015 if (led1_state) { 00016 led1 = 0; led1_state = 0; 00017 } else { 00018 led1 = 1; led1_state = 1; 00019 } 00020 print_char(); 00021 } 00022 00023 Ticker flipper_2; 00024 DigitalOut led2(LED2); 00025 00026 void flip_2() { 00027 static int led2_state = 0; 00028 if (led2_state) { 00029 led2 = 0; led2_state = 0; 00030 } else { 00031 led2 = 1; led2_state = 1; 00032 } 00033 } 00034 00035 int main() { 00036 MBED_HOSTTEST_TIMEOUT(15); 00037 MBED_HOSTTEST_SELECT(wait_us_auto); 00038 MBED_HOSTTEST_DESCRIPTION(Ticker Int); 00039 MBED_HOSTTEST_START("MBED_11"); 00040 00041 led1 = 0; 00042 led2 = 0; 00043 flipper_1.attach(&flip_1, 1.0); // the address of the function to be attached (flip) and the interval (1 second) 00044 flipper_2.attach(&flip_2, 2.0); // the address of the function to be attached (flip) and the interval (2 seconds) 00045 00046 while (true) { 00047 wait(1.0); 00048 } 00049 }
Generated on Sun Jul 17 2022 08:25:26 by
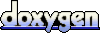