
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "test_env.h" 00002 #include "mbed_semihost_api.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 00006 #define FILENAME "/local/out.txt" 00007 #define TEST_STRING "Hello World!" 00008 00009 FILE *test_open(const char *mode) { 00010 FILE *f = fopen(FILENAME, mode); 00011 if (f == NULL) { 00012 printf("Error opening file"NL); 00013 notify_completion(false); 00014 } 00015 return f; 00016 } 00017 00018 void test_write(FILE *f, char *str, int str_len) { 00019 int n = fprintf(f, str); 00020 00021 if (n != str_len) { 00022 printf("Error writing file"NL); 00023 notify_completion(false); 00024 } 00025 } 00026 00027 void test_read(FILE *f, char *str, int str_len) { 00028 int n = fread(str, sizeof(unsigned char), str_len, f); 00029 00030 if (n != str_len) { 00031 printf("Error reading file"NL); 00032 notify_completion(false); 00033 } 00034 } 00035 00036 void test_close(FILE *f) { 00037 int rc = fclose(f); 00038 00039 if (rc != 0) { 00040 printf("Error closing file"NL); 00041 notify_completion(false); 00042 } 00043 } 00044 00045 int main() { 00046 MBED_HOSTTEST_TIMEOUT(20); 00047 MBED_HOSTTEST_SELECT(default_auto); 00048 MBED_HOSTTEST_DESCRIPTION(Semihost file system); 00049 MBED_HOSTTEST_START("MBED_A2"); 00050 00051 pc.printf("Test the Stream class\n"); 00052 00053 printf("connected: %s\n", (semihost_connected()) ? ("Yes") : ("No")); 00054 00055 char mac[16]; 00056 mbed_mac_address(mac); 00057 printf("mac address: %02x,%02x,%02x,%02x,%02x,%02x\n", mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]); 00058 00059 LocalFileSystem local("local"); 00060 00061 FILE *f; 00062 char *str = TEST_STRING; 00063 char *buffer = (char *)malloc(sizeof(unsigned char) * strlen(TEST_STRING)); 00064 int str_len = strlen(TEST_STRING); 00065 00066 // Write 00067 f = test_open("w"); 00068 test_write(f, str, str_len); 00069 test_close(f); 00070 00071 // Read 00072 f = test_open("r"); 00073 test_read(f, buffer, str_len); 00074 test_close(f); 00075 00076 // Check the two strings are equal 00077 MBED_HOSTTEST_RESULT((strncmp(buffer, str, str_len) == 0)); 00078 }
Generated on Sun Jul 17 2022 08:25:26 by
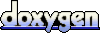