
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #if defined(TARGET_LPC1768) 00004 #define UART_TX p9 00005 #define UART_RX p10 00006 #define FLOW_CONTROL_RTS p30 00007 #define FLOW_CONTROL_CTS p29 00008 #define RTS_CHECK_PIN p8 00009 00010 #elif defined(TARGET_SAMR21G18A) 00011 #define UART_TX PA04 00012 #define UART_RX PA05 00013 #define FLOW_CONTROL_RTS PA06 00014 #define FLOW_CONTROL_CTS PA07 00015 #define RTS_CHECK_PIN PB03 00016 00017 #elif defined(TARGET_SAMD21J18A) /*USB debug port is not having RTS and CTS pins in expansion connectors in D21 Xplained Pro Board*/ 00018 #define UART_TX PA22 /* Short this pin to PA04 */ 00019 #define UART_RX PA23 /* Short this pin to PA05 */ 00020 #define FLOW_CONTROL_RTS PA24 00021 #define FLOW_CONTROL_CTS PA25 00022 #define RTS_CHECK_PIN PB03 00023 00024 #elif defined(TARGET_SAMD21G18A) /*USB debug port is not having RTS and CTS pins in expansion connectors in W25 Xplained Pro Board*/ 00025 #define UART_TX PA16 /* Short this pin to PB10 */ 00026 #define UART_RX PA17 /* Short this pin to PB11 */ 00027 #define FLOW_CONTROL_RTS PA18 00028 #define FLOW_CONTROL_CTS PA19 00029 #define RTS_CHECK_PIN PB03 00030 00031 #elif defined(TARGET_SAMG55J19) 00032 #define UART_TX PA28 00033 #define UART_RX PA27 00034 #define FLOW_CONTROL_RTS PA31 00035 #define FLOW_CONTROL_CTS PA30 00036 #define RTS_CHECK_PIN PA18 00037 00038 #else 00039 #error This test is not supported on this target 00040 #endif 00041 00042 Serial pc(UART_TX, UART_RX); 00043 00044 #ifdef RTS_CHECK_PIN 00045 InterruptIn in(RTS_CHECK_PIN); 00046 DigitalOut led(LED1); 00047 static void checker(void) { 00048 led = !led; 00049 } 00050 #endif 00051 00052 int main() { 00053 char buf[256]; 00054 00055 pc.set_flow_control(Serial::RTSCTS, FLOW_CONTROL_RTS, FLOW_CONTROL_CTS); 00056 #ifdef RTS_CHECK_PIN 00057 in.fall(checker); 00058 #endif 00059 while (1) { 00060 pc.gets(buf, 256); 00061 pc.printf("%s", buf); 00062 } 00063 }
Generated on Sun Jul 17 2022 08:25:26 by
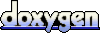