
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut status_led(LED_BLUE); 00004 DigitalOut error_led(LED_RED); 00005 00006 extern "C" void RTC_IRQHandler(void) { 00007 error_led = 0; 00008 } 00009 00010 extern "C" void RTC_Seconds_IRQHandler(void) { 00011 error_led = 0; 00012 } 00013 00014 extern "C" void HardFault_Handler(void) { 00015 error_led = 0; 00016 } 00017 00018 extern "C" void NMI_Handler_Handler(void) { 00019 error_led = 0; 00020 } 00021 00022 void rtc_init(void) { 00023 // enable the clock to SRTC module register space 00024 SIM->SCGC6 |= SIM_SCGC6_RTC_MASK; 00025 SIM->SOPT1 = (SIM->SOPT1 & ~SIM_SOPT1_OSC32KSEL_MASK) | SIM_SOPT1_OSC32KSEL(0); 00026 00027 // disable interrupts 00028 NVIC_DisableIRQ(RTC_Seconds_IRQn); 00029 NVIC_DisableIRQ(RTC_IRQn); 00030 00031 // Reset 00032 RTC->CR = RTC_CR_SWR_MASK; 00033 RTC->CR &= ~RTC_CR_SWR_MASK; 00034 00035 // Allow write 00036 RTC->CR = RTC_CR_UM_MASK | RTC_CR_SUP_MASK; 00037 00038 NVIC_EnableIRQ(RTC_Seconds_IRQn); 00039 NVIC_EnableIRQ(RTC_Seconds_IRQn); 00040 00041 printf("LR: 0x%x\n", RTC->LR); 00042 printf("CR: 0x%x\n", RTC->CR); 00043 wait(1); 00044 if (RTC->SR & RTC_SR_TIF_MASK){ 00045 RTC->TSR = 0; 00046 } 00047 RTC->TCR = 0; 00048 00049 // After setting this bit, wait the oscillator startup time before enabling 00050 // the time counter to allow the clock time to stabilize 00051 RTC->CR |= RTC_CR_OSCE_MASK; 00052 for (volatile int i=0; i<0x600000; i++); 00053 00054 //enable seconds interrupts 00055 RTC->IER |= RTC_IER_TSIE_MASK; 00056 00057 // enable time counter 00058 RTC->SR |= RTC_SR_TCE_MASK; 00059 00060 00061 } 00062 00063 int main() { 00064 error_led = 1; 00065 rtc_init(); 00066 00067 while (true) { 00068 wait(1); 00069 status_led = !status_led; 00070 printf("%u\n", RTC->TSR); 00071 } 00072 }
Generated on Sun Jul 17 2022 08:25:26 by
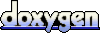