
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 /* mbed Microcontroller Library 00003 * Copyright (c) 2013-2015 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 #include "utest/utest.h" 00020 #include "unity/unity.h" 00021 00022 00023 using namespace utest::v1; 00024 00025 void never_call_case() 00026 { 00027 TEST_FAIL_MESSAGE("Case handler should have never been called!"); 00028 } 00029 Case cases[] = 00030 { 00031 Case("dummy test", never_call_case), 00032 Case("dummy test 2", never_call_case) 00033 }; 00034 00035 // this setup handler fails 00036 utest::v1::status_t failing_setup_handler(const size_t number_of_cases) 00037 { 00038 GREENTEA_SETUP(5, "default_auto"); 00039 00040 TEST_ASSERT_EQUAL(2, number_of_cases); 00041 greentea_test_setup_handler(number_of_cases); 00042 return STATUS_ABORT; // aborting test 00043 }; 00044 00045 // the teardown handler will then be called with the reason `REASON_TEST_SETUP` 00046 void failing_teardown_handler(const size_t passed, const size_t failed, const failure_t failure) 00047 { 00048 TEST_ASSERT_EQUAL(0, passed); 00049 TEST_ASSERT_EQUAL(0, failed); 00050 TEST_ASSERT_EQUAL(REASON_TEST_SETUP, failure.reason); 00051 TEST_ASSERT_EQUAL(LOCATION_TEST_SETUP, failure.location); 00052 00053 verbose_test_teardown_handler(passed, failed, failure); 00054 00055 // pretend to greentea that we actally executed two test case 00056 greentea_case_setup_handler(cases, 0); 00057 greentea_case_teardown_handler(cases, 1, 0, REASON_NONE); 00058 00059 greentea_case_setup_handler(cases + 1, 0); 00060 greentea_case_teardown_handler(cases + 1, 1, 0, REASON_NONE); 00061 00062 greentea_test_teardown_handler(2, 0, REASON_NONE); 00063 }; 00064 00065 Specification specification(failing_setup_handler, cases, failing_teardown_handler, selftest_handlers); 00066 00067 int main() 00068 { 00069 Harness::run(specification); 00070 }
Generated on Sun Jul 17 2022 08:25:26 by
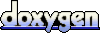