
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "greentea-client/test_env.h" 00019 #include "unity.h" 00020 #include "utest.h" 00021 #include "rtos.h" 00022 00023 using namespace utest::v1; 00024 00025 #if !defined(MBEDTLS_CONFIG_FILE) 00026 #include "mbedtls/config.h" 00027 #else 00028 #include MBEDTLS_CONFIG_FILE 00029 #endif 00030 00031 #include "mbedtls/sha256.h" 00032 #include "mbedtls/sha512.h" 00033 #include "mbedtls/entropy.h" 00034 #include "mbedtls/entropy_poll.h" 00035 00036 #include <string.h> 00037 00038 #if defined(MBEDTLS_PLATFORM_C) 00039 #include "mbedtls/platform.h" 00040 #else 00041 #include <stdio.h> 00042 #include <stdlib.h> 00043 #define mbedtls_printf printf 00044 #define mbedtls_snprintf snprintf 00045 #define mbedtls_exit exit 00046 #define MBEDTLS_EXIT_SUCCESS EXIT_SUCCESS 00047 #define MBEDTLS_EXIT_FAILURE EXIT_FAILURE 00048 #endif 00049 00050 #define MBEDTLS_SELF_TEST_TEST_CASE(self_test_function) \ 00051 void self_test_function ## _test_case() { \ 00052 int ret = self_test_function(0); \ 00053 TEST_ASSERT_EQUAL(ret, 0); \ 00054 } 00055 00056 #if defined(MBEDTLS_SELF_TEST) 00057 00058 #if defined(MBEDTLS_SHA256_C) 00059 MBEDTLS_SELF_TEST_TEST_CASE(mbedtls_sha256_self_test) 00060 #endif 00061 00062 #if defined(MBEDTLS_SHA512_C) 00063 MBEDTLS_SELF_TEST_TEST_CASE(mbedtls_sha512_self_test) 00064 #endif 00065 00066 #if defined(MBEDTLS_ENTROPY_C) 00067 MBEDTLS_SELF_TEST_TEST_CASE(mbedtls_entropy_self_test) 00068 #endif 00069 00070 #else 00071 #warning "MBEDTLS_SELF_TEST not enabled" 00072 #endif /* MBEDTLS_SELF_TEST */ 00073 00074 Case cases[] = { 00075 #if defined(MBEDTLS_SELF_TEST) 00076 00077 #if defined(MBEDTLS_SHA256_C) 00078 Case("mbedtls_sha256_self_test", mbedtls_sha256_self_test_test_case), 00079 #endif 00080 00081 #if defined(MBEDTLS_SHA512_C) 00082 Case("mbedtls_sha512_self_test", mbedtls_sha512_self_test_test_case), 00083 #endif 00084 00085 #if defined(MBEDTLS_ENTROPY_C) 00086 Case("mbedtls_entropy_self_test", mbedtls_entropy_self_test_test_case), 00087 #endif 00088 00089 #endif /* MBEDTLS_SELF_TEST */ 00090 }; 00091 00092 utest::v1::status_t test_setup(const size_t num_cases) { 00093 GREENTEA_SETUP(120, "default_auto"); 00094 return verbose_test_setup_handler(num_cases); 00095 } 00096 00097 Specification specification(test_setup, cases); 00098 00099 int main() { 00100 return !Harness::run(specification); 00101 } 00102
Generated on Sun Jul 17 2022 08:25:27 by
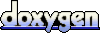