
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2013-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include <stdio.h> 00018 #include <string.h> 00019 #include "mbed.h" 00020 #include "greentea-client/test_env.h" 00021 #include "unity/unity.h" 00022 #include "utest/utest.h" 00023 00024 #include "mbedtls/sha256.h" 00025 00026 00027 using namespace utest::v1; 00028 00029 #if defined(MBEDTLS_SHA256_C) 00030 /* Tests several call to mbedtls_sha256_update function that are not modulo 64 bytes */ 00031 void test_case_sha256_split() { 00032 const unsigned char test_buf[] = {"abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq"}; 00033 // sha256_output_values for 3*abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00034 const unsigned char test_sum[] = 00035 { 0x50, 0xEA, 0x82, 0x5D, 0x96, 0x84, 0xF4, 0x22, 00036 0x9C, 0xA2, 0x9F, 0x1F, 0xEC, 0x51, 0x15, 0x93, 00037 0xE2, 0x81, 0xE4, 0x6A, 0x14, 0x0D, 0x81, 0xE0, 00038 0x00, 0x5F, 0x8F, 0x68, 0x86, 0x69, 0xA0, 0x6C}; 00039 unsigned char outsum[32]; 00040 int i; 00041 00042 mbedtls_sha256_context ctx; 00043 printf("test sha256\n"); 00044 mbedtls_sha256_init( &ctx ); 00045 mbedtls_sha256_starts( &ctx, 0); 00046 #if 0 00047 printf("test not splitted\n"); 00048 mbedtls_sha256_update( &ctx, test_buf, 168 ); 00049 #else 00050 printf("test splitted into 3 pieces\n"); 00051 mbedtls_sha256_update( &ctx, test_buf, 2 ); 00052 mbedtls_sha256_update( &ctx, test_buf+2, 66 ); 00053 mbedtls_sha256_update( &ctx, test_buf+68, 100 ); 00054 #endif 00055 00056 mbedtls_sha256_finish( &ctx, outsum ); 00057 mbedtls_sha256_free( &ctx ); 00058 00059 printf("\nreceived result : "); 00060 for (i=0;i<32;i++) { printf("%02X",outsum[i]);} 00061 printf("\nawaited result : 50EA825D9684F4229CA29F1FEC511593E281E46A140D81E0005F8F688669A06C\n"); // for abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00062 00063 printf("\nend of test sha256\n"); 00064 TEST_ASSERT_EQUAL_UINT8_ARRAY(outsum, test_sum,32); 00065 } 00066 00067 /* Tests that treating 2 sha256 objects in // does not impact the result */ 00068 void test_case_sha256_multi() { 00069 const unsigned char test_buf[] = {"abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq"}; 00070 const unsigned char test_buf2[] = {"abcdefghijklmnopqrstuvwxyz012345678901234567890123456789"}; 00071 00072 // sha256_output_values for abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00073 const unsigned char test_sum1[] = 00074 { 0x24, 0x8D, 0x6A, 0x61, 0xD2, 0x06, 0x38, 0xB8, 00075 0xE5, 0xC0, 0x26, 0x93, 0x0C, 0x3E, 0x60, 0x39, 00076 0xA3, 0x3C, 0xE4, 0x59, 0x64, 0xFF, 0x21, 0x67, 00077 0xF6, 0xEC, 0xED, 0xD4, 0x19, 0xDB, 0x06, 0xC1 }; 00078 // sha256_output_values for 3*abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00079 const unsigned char test_sum2[] = 00080 { 0x50, 0xEA, 0x82, 0x5D, 0x96, 0x84, 0xF4, 0x22, 00081 0x9C, 0xA2, 0x9F, 0x1F, 0xEC, 0x51, 0x15, 0x93, 00082 0xE2, 0x81, 0xE4, 0x6A, 0x14, 0x0D, 0x81, 0xE0, 00083 0x00, 0x5F, 0x8F, 0x68, 0x86, 0x69, 0xA0, 0x6C}; 00084 // sha256_output_values for abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopqabcdefghijklmnopqrstuvwxyz012345678901234567890123456789 00085 const unsigned char test_sum3[] = 00086 { 0x6D, 0x5D, 0xDB, 0x5F, 0x4A, 0x94, 0xAB, 0x7E, 00087 0x5C, 0xF7, 0x9A, 0xD8, 0x3F, 0x58, 0xD3, 0x97, 00088 0xFE, 0x79, 0xFB, 0x0D, 0x79, 0xB2, 0x0D, 0x22, 00089 0xFF, 0x95, 0x9F, 0x04, 0xA2, 0xE4, 0x6C, 0x68}; 00090 unsigned char outsum1[32], outsum2[32], outsum3[32]; 00091 int i; 00092 00093 mbedtls_sha256_context ctx1; 00094 mbedtls_sha256_context ctx2; 00095 mbedtls_sha256_context ctx3; 00096 printf("test sha256_multi\n"); 00097 //Init both contexts 00098 mbedtls_sha256_init( &ctx1); 00099 mbedtls_sha256_init( &ctx2); 00100 mbedtls_sha256_init( &ctx3); 00101 //Start both contexts 00102 mbedtls_sha256_starts( &ctx1, 0); 00103 mbedtls_sha256_starts( &ctx2, 0); 00104 00105 printf("upd ctx1\n"); 00106 mbedtls_sha256_update( &ctx1, test_buf, 56 ); 00107 printf("upd ctx2\n"); 00108 mbedtls_sha256_update( &ctx2, test_buf, 66 ); 00109 printf("finish ctx1\n"); 00110 mbedtls_sha256_finish( &ctx1, outsum1 ); 00111 printf("upd ctx2\n"); 00112 mbedtls_sha256_update( &ctx2, test_buf+66, 46 ); 00113 printf("clone ctx2 in ctx3\n"); 00114 mbedtls_sha256_clone(&ctx3, (const mbedtls_sha256_context *)&ctx2); 00115 printf("free ctx1\n"); 00116 mbedtls_sha256_free( &ctx1 ); 00117 printf("upd ctx2\n"); 00118 mbedtls_sha256_update( &ctx2, test_buf+112, 56 ); 00119 printf("upd ctx3 with different values than ctx2\n"); 00120 mbedtls_sha256_update( &ctx3, test_buf2, 56 ); 00121 printf("finish ctx2\n"); 00122 mbedtls_sha256_finish( &ctx2, outsum2 ); 00123 printf("finish ctx3\n"); 00124 mbedtls_sha256_finish( &ctx3, outsum3 ); 00125 printf("free ctx2\n"); 00126 mbedtls_sha256_free( &ctx2 ); 00127 printf("free ctx3\n"); 00128 mbedtls_sha256_free( &ctx3 ); 00129 00130 printf("\nreceived result ctx1 : "); 00131 for (i=0;i<32;i++) { printf("%02X",outsum1[i]);} 00132 printf("\nawaited result : 248D6A61D20638B8E5C026930C3E6039A33CE45964FF216F6ECEDD19DB06C1\n"); // for abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00133 printf("\nreceived result ctx2 : "); 00134 for (i=0;i<32;i++) { printf("%02X",outsum2[i]);} 00135 printf("\nawaited result : 50EA825D9684F4229CA29F1FEC511593E281E46A140D81E0005F8F688669A06C\n"); // for 3*abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq 00136 printf("\nreceived result ctx3 : "); 00137 for (i=0;i<32;i++) { printf("%02X",outsum3[i]);} 00138 printf("\nawaited result : 6D5DDB5F4A94AB7E5CF79AD83F58D397FE79FB0D79B20D22FF959F04A2E46C68\n"); // for 2*abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq+3*0123456789 00139 printf("\nend of test sha256\n"); 00140 TEST_ASSERT_EQUAL_UINT8_ARRAY(outsum1, test_sum1,32); 00141 TEST_ASSERT_EQUAL_UINT8_ARRAY(outsum2, test_sum2,32); 00142 TEST_ASSERT_EQUAL_UINT8_ARRAY(outsum3, test_sum3,32); 00143 } 00144 #endif //MBEDTLS_SHA256_C 00145 00146 utest::v1::status_t greentea_failure_handler(const Case *const source, const failure_t reason) { 00147 greentea_case_failure_abort_handler(source, reason); 00148 return STATUS_CONTINUE; 00149 } 00150 00151 Case cases[] = { 00152 #if defined(MBEDTLS_SHA256_C) 00153 Case("Crypto: sha256_split", test_case_sha256_split, greentea_failure_handler), 00154 Case("Crypto: sha256_multi", test_case_sha256_multi, greentea_failure_handler), 00155 #endif 00156 }; 00157 00158 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) { 00159 GREENTEA_SETUP(10, "default_auto"); 00160 return greentea_test_setup_handler(number_of_cases); 00161 } 00162 00163 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00164 00165 int main() { 00166 Harness::run(specification); 00167 }
Generated on Sun Jul 17 2022 08:25:27 by
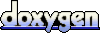