
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "utest/utest.h" 00017 #include "unity/unity.h" 00018 #include "greentea-client/test_env.h" 00019 00020 #if !DEVICE_SLEEP 00021 #error [NOT_SUPPORTED] test not supported 00022 #endif 00023 00024 using namespace utest::v1; 00025 00026 void sleep_manager_deepsleep_counter_test() 00027 { 00028 bool deep_sleep_allowed = sleep_manager_can_deep_sleep(); 00029 TEST_ASSERT_TRUE(deep_sleep_allowed); 00030 00031 sleep_manager_lock_deep_sleep(); 00032 deep_sleep_allowed = sleep_manager_can_deep_sleep(); 00033 TEST_ASSERT_FALSE(deep_sleep_allowed); 00034 00035 sleep_manager_unlock_deep_sleep(); 00036 deep_sleep_allowed = sleep_manager_can_deep_sleep(); 00037 TEST_ASSERT_TRUE(deep_sleep_allowed); 00038 } 00039 00040 utest::v1::status_t greentea_failure_handler(const Case *const source, const failure_t reason) 00041 { 00042 greentea_case_failure_abort_handler(source, reason); 00043 return STATUS_CONTINUE; 00044 } 00045 00046 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) 00047 { 00048 GREENTEA_SETUP(20, "default_auto"); 00049 return greentea_test_setup_handler(number_of_cases); 00050 } 00051 00052 Case cases[] = { 00053 Case("sleep manager - deep sleep counter", sleep_manager_deepsleep_counter_test, greentea_failure_handler), 00054 }; 00055 00056 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00057 00058 int main() { 00059 Harness::run(specification); 00060 }
Generated on Sun Jul 17 2022 08:25:27 by
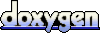