
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "greentea-client/test_env.h" 00018 #include "unity.h" 00019 #include "utest.h" 00020 00021 using namespace utest::v1; 00022 00023 00024 // static functions 00025 template <typename T> 00026 T static_func0() { return 0; } 00027 template <typename T> 00028 T static_func1(T a0) { return 0 | a0; } 00029 template <typename T> 00030 T static_func2(T a0, T a1) { return 0 | a0 | a1; } 00031 template <typename T> 00032 T static_func3(T a0, T a1, T a2) { return 0 | a0 | a1 | a2; } 00033 template <typename T> 00034 T static_func4(T a0, T a1, T a2, T a3) { return 0 | a0 | a1 | a2 | a3; } 00035 template <typename T> 00036 T static_func5(T a0, T a1, T a2, T a3, T a4) { return 0 | a0 | a1 | a2 | a3 | a4; } 00037 00038 // class functions 00039 template <typename T> 00040 struct Thing { 00041 T t; 00042 Thing() : t(0x80) {} 00043 00044 T member_func0() { return t; } 00045 T member_func1(T a0) { return t | a0; } 00046 T member_func2(T a0, T a1) { return t | a0 | a1; } 00047 T member_func3(T a0, T a1, T a2) { return t | a0 | a1 | a2; } 00048 T member_func4(T a0, T a1, T a2, T a3) { return t | a0 | a1 | a2 | a3; } 00049 T member_func5(T a0, T a1, T a2, T a3, T a4) { return t | a0 | a1 | a2 | a3 | a4; } 00050 00051 T const_member_func0() const { return t; } 00052 T const_member_func1(T a0) const { return t | a0; } 00053 T const_member_func2(T a0, T a1) const { return t | a0 | a1; } 00054 T const_member_func3(T a0, T a1, T a2) const { return t | a0 | a1 | a2; } 00055 T const_member_func4(T a0, T a1, T a2, T a3) const { return t | a0 | a1 | a2 | a3; } 00056 T const_member_func5(T a0, T a1, T a2, T a3, T a4) const { return t | a0 | a1 | a2 | a3 | a4; } 00057 00058 T volatile_member_func0() volatile { return t; } 00059 T volatile_member_func1(T a0) volatile { return t | a0; } 00060 T volatile_member_func2(T a0, T a1) volatile { return t | a0 | a1; } 00061 T volatile_member_func3(T a0, T a1, T a2) volatile { return t | a0 | a1 | a2; } 00062 T volatile_member_func4(T a0, T a1, T a2, T a3) volatile { return t | a0 | a1 | a2 | a3; } 00063 T volatile_member_func5(T a0, T a1, T a2, T a3, T a4) volatile { return t | a0 | a1 | a2 | a3 | a4; } 00064 00065 T const_volatile_member_func0() const volatile { return t; } 00066 T const_volatile_member_func1(T a0) const volatile { return t | a0; } 00067 T const_volatile_member_func2(T a0, T a1) const volatile { return t | a0 | a1; } 00068 T const_volatile_member_func3(T a0, T a1, T a2) const volatile { return t | a0 | a1 | a2; } 00069 T const_volatile_member_func4(T a0, T a1, T a2, T a3) const volatile { return t | a0 | a1 | a2 | a3; } 00070 T const_volatile_member_func5(T a0, T a1, T a2, T a3, T a4) const volatile { return t | a0 | a1 | a2 | a3 | a4; } 00071 }; 00072 00073 // bound functions 00074 template <typename T> 00075 T bound_func0(Thing<T> *t) { return t->t; } 00076 template <typename T> 00077 T bound_func1(Thing<T> *t, T a0) { return t->t | a0; } 00078 template <typename T> 00079 T bound_func2(Thing<T> *t, T a0, T a1) { return t->t | a0 | a1; } 00080 template <typename T> 00081 T bound_func3(Thing<T> *t, T a0, T a1, T a2) { return t->t | a0 | a1 | a2; } 00082 template <typename T> 00083 T bound_func4(Thing<T> *t, T a0, T a1, T a2, T a3) { return t->t | a0 | a1 | a2 | a3; } 00084 template <typename T> 00085 T bound_func5(Thing<T> *t, T a0, T a1, T a2, T a3, T a4) { return t->t | a0 | a1 | a2 | a3 | a4; } 00086 00087 // const bound functions 00088 template <typename T> 00089 T const_func0(const Thing<T> *t) { return t->t; } 00090 template <typename T> 00091 T const_func1(const Thing<T> *t, T a0) { return t->t | a0; } 00092 template <typename T> 00093 T const_func2(const Thing<T> *t, T a0, T a1) { return t->t | a0 | a1; } 00094 template <typename T> 00095 T const_func3(const Thing<T> *t, T a0, T a1, T a2) { return t->t | a0 | a1 | a2; } 00096 template <typename T> 00097 T const_func4(const Thing<T> *t, T a0, T a1, T a2, T a3) { return t->t | a0 | a1 | a2 | a3; } 00098 template <typename T> 00099 T const_func5(const Thing<T> *t, T a0, T a1, T a2, T a3, T a4) { return t->t | a0 | a1 | a2 | a3 | a4; } 00100 00101 // volatile bound functions 00102 template <typename T> 00103 T volatile_func0(volatile Thing<T> *t) { return t->t; } 00104 template <typename T> 00105 T volatile_func1(volatile Thing<T> *t, T a0) { return t->t | a0; } 00106 template <typename T> 00107 T volatile_func2(volatile Thing<T> *t, T a0, T a1) { return t->t | a0 | a1; } 00108 template <typename T> 00109 T volatile_func3(volatile Thing<T> *t, T a0, T a1, T a2) { return t->t | a0 | a1 | a2; } 00110 template <typename T> 00111 T volatile_func4(volatile Thing<T> *t, T a0, T a1, T a2, T a3) { return t->t | a0 | a1 | a2 | a3; } 00112 template <typename T> 00113 T volatile_func5(volatile Thing<T> *t, T a0, T a1, T a2, T a3, T a4) { return t->t | a0 | a1 | a2 | a3 | a4; } 00114 00115 // const volatile bound functions 00116 template <typename T> 00117 T const_volatile_func0(const volatile Thing<T> *t) { return t->t; } 00118 template <typename T> 00119 T const_volatile_func1(const volatile Thing<T> *t, T a0) { return t->t | a0; } 00120 template <typename T> 00121 T const_volatile_func2(const volatile Thing<T> *t, T a0, T a1) { return t->t | a0 | a1; } 00122 template <typename T> 00123 T const_volatile_func3(const volatile Thing<T> *t, T a0, T a1, T a2) { return t->t | a0 | a1 | a2; } 00124 template <typename T> 00125 T const_volatile_func4(const volatile Thing<T> *t, T a0, T a1, T a2, T a3) { return t->t | a0 | a1 | a2 | a3; } 00126 template <typename T> 00127 T const_volatile_func5(const volatile Thing<T> *t, T a0, T a1, T a2, T a3, T a4) { return t->t | a0 | a1 | a2 | a3 | a4; } 00128 00129 00130 // function call and result verification 00131 template <typename T> 00132 struct Verifier { 00133 static void verify0(Callback<T()> func) { 00134 T result = func(); 00135 TEST_ASSERT_EQUAL(result, 0x00); 00136 } 00137 00138 template <typename O, typename M> 00139 static void verify0(O *obj, M method) { 00140 Callback<T()> func(obj, method); 00141 T result = func(); 00142 TEST_ASSERT_EQUAL(result, 0x80); 00143 } 00144 00145 static void verify1(Callback<T(T)> func) { 00146 T result = func((1 << 0)); 00147 TEST_ASSERT_EQUAL(result, 0x00 | (1 << 0)); 00148 } 00149 00150 template <typename O, typename M> 00151 static void verify1(O *obj, M method) { 00152 Callback<T(T)> func(obj, method); 00153 T result = func((1 << 0)); 00154 TEST_ASSERT_EQUAL(result, 0x80 | (1 << 0)); 00155 } 00156 00157 static void verify2(Callback<T(T, T)> func) { 00158 T result = func((1 << 0), (1 << 1)); 00159 TEST_ASSERT_EQUAL(result, 0x00 | (1 << 0) | (1 << 1)); 00160 } 00161 00162 template <typename O, typename M> 00163 static void verify2(O *obj, M method) { 00164 Callback<T(T, T)> func(obj, method); 00165 T result = func((1 << 0), (1 << 1)); 00166 TEST_ASSERT_EQUAL(result, 0x80 | (1 << 0) | (1 << 1)); 00167 } 00168 00169 static void verify3(Callback<T(T, T, T)> func) { 00170 T result = func((1 << 0), (1 << 1), (1 << 2)); 00171 TEST_ASSERT_EQUAL(result, 0x00 | (1 << 0) | (1 << 1) | (1 << 2)); 00172 } 00173 00174 template <typename O, typename M> 00175 static void verify3(O *obj, M method) { 00176 Callback<T(T, T, T)> func(obj, method); 00177 T result = func((1 << 0), (1 << 1), (1 << 2)); 00178 TEST_ASSERT_EQUAL(result, 0x80 | (1 << 0) | (1 << 1) | (1 << 2)); 00179 } 00180 00181 static void verify4(Callback<T(T, T, T, T)> func) { 00182 T result = func((1 << 0), (1 << 1), (1 << 2), (1 << 3)); 00183 TEST_ASSERT_EQUAL(result, 0x00 | (1 << 0) | (1 << 1) | (1 << 2) | (1 << 3)); 00184 } 00185 00186 template <typename O, typename M> 00187 static void verify4(O *obj, M method) { 00188 Callback<T(T, T, T, T)> func(obj, method); 00189 T result = func((1 << 0), (1 << 1), (1 << 2), (1 << 3)); 00190 TEST_ASSERT_EQUAL(result, 0x80 | (1 << 0) | (1 << 1) | (1 << 2) | (1 << 3)); 00191 } 00192 00193 static void verify5(Callback<T(T, T, T, T, T)> func) { 00194 T result = func((1 << 0), (1 << 1), (1 << 2), (1 << 3), (1 << 4)); 00195 TEST_ASSERT_EQUAL(result, 0x00 | (1 << 0) | (1 << 1) | (1 << 2) | (1 << 3) | (1 << 4)); 00196 } 00197 00198 template <typename O, typename M> 00199 static void verify5(O *obj, M method) { 00200 Callback<T(T, T, T, T, T)> func(obj, method); 00201 T result = func((1 << 0), (1 << 1), (1 << 2), (1 << 3), (1 << 4)); 00202 TEST_ASSERT_EQUAL(result, 0x80 | (1 << 0) | (1 << 1) | (1 << 2) | (1 << 3) | (1 << 4)); 00203 } 00204 }; 00205 00206 00207 // test dispatch 00208 template <typename T> 00209 void test_dispatch0() { 00210 Thing<T> thing; 00211 Verifier<T>::verify0(static_func0<T>); 00212 Verifier<T>::verify0(&thing, &Thing<T>::member_func0); 00213 Verifier<T>::verify0((const Thing<T>*)&thing, &Thing<T>::const_member_func0); 00214 Verifier<T>::verify0((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func0); 00215 Verifier<T>::verify0((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func0); 00216 Verifier<T>::verify0(&bound_func0<T>, &thing); 00217 Verifier<T>::verify0(&const_func0<T>, (const Thing<T>*)&thing); 00218 Verifier<T>::verify0(&volatile_func0<T>, (volatile Thing<T>*)&thing); 00219 Verifier<T>::verify0(&const_volatile_func0<T>, (const volatile Thing<T>*)&thing); 00220 Verifier<T>::verify0(callback(static_func0<T>)); 00221 00222 Callback<T()> cb(static_func0); 00223 Verifier<T>::verify0(cb); 00224 cb = static_func0; 00225 Verifier<T>::verify0(cb); 00226 cb.attach(&bound_func0<T>, &thing); 00227 Verifier<T>::verify0(&cb, &Callback<T()>::call); 00228 Verifier<T>::verify0(&Callback<T()>::thunk, (void*)&cb); 00229 } 00230 00231 template <typename T> 00232 void test_dispatch1() { 00233 Thing<T> thing; 00234 Verifier<T>::verify1(static_func1<T>); 00235 Verifier<T>::verify1(&thing, &Thing<T>::member_func1); 00236 Verifier<T>::verify1((const Thing<T>*)&thing, &Thing<T>::const_member_func1); 00237 Verifier<T>::verify1((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func1); 00238 Verifier<T>::verify1((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func1); 00239 Verifier<T>::verify1(&bound_func1<T>, &thing); 00240 Verifier<T>::verify1(&const_func1<T>, (const Thing<T>*)&thing); 00241 Verifier<T>::verify1(&volatile_func1<T>, (volatile Thing<T>*)&thing); 00242 Verifier<T>::verify1(&const_volatile_func1<T>, (const volatile Thing<T>*)&thing); 00243 Verifier<T>::verify1(callback(static_func1<T>)); 00244 00245 Callback<T(T)> cb(static_func1); 00246 Verifier<T>::verify1(cb); 00247 cb = static_func1; 00248 Verifier<T>::verify1(cb); 00249 cb.attach(&bound_func1<T>, &thing); 00250 Verifier<T>::verify1(&cb, &Callback<T(T)>::call); 00251 Verifier<T>::verify1(&Callback<T(T)>::thunk, (void*)&cb); 00252 } 00253 00254 template <typename T> 00255 void test_dispatch2() { 00256 Thing<T> thing; 00257 Verifier<T>::verify2(static_func2<T>); 00258 Verifier<T>::verify2(&thing, &Thing<T>::member_func2); 00259 Verifier<T>::verify2((const Thing<T>*)&thing, &Thing<T>::const_member_func2); 00260 Verifier<T>::verify2((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func2); 00261 Verifier<T>::verify2((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func2); 00262 Verifier<T>::verify2(&bound_func2<T>, &thing); 00263 Verifier<T>::verify2(&const_func2<T>, (const Thing<T>*)&thing); 00264 Verifier<T>::verify2(&volatile_func2<T>, (volatile Thing<T>*)&thing); 00265 Verifier<T>::verify2(&const_volatile_func2<T>, (const volatile Thing<T>*)&thing); 00266 Verifier<T>::verify2(callback(static_func2<T>)); 00267 00268 Callback<T(T, T)> cb(static_func2); 00269 Verifier<T>::verify2(cb); 00270 cb = static_func2; 00271 Verifier<T>::verify2(cb); 00272 cb.attach(&bound_func2<T>, &thing); 00273 Verifier<T>::verify2(&cb, &Callback<T(T, T)>::call); 00274 Verifier<T>::verify2(&Callback<T(T, T)>::thunk, (void*)&cb); 00275 } 00276 00277 template <typename T> 00278 void test_dispatch3() { 00279 Thing<T> thing; 00280 Verifier<T>::verify3(static_func3<T>); 00281 Verifier<T>::verify3(&thing, &Thing<T>::member_func3); 00282 Verifier<T>::verify3((const Thing<T>*)&thing, &Thing<T>::const_member_func3); 00283 Verifier<T>::verify3((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func3); 00284 Verifier<T>::verify3((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func3); 00285 Verifier<T>::verify3(&bound_func3<T>, &thing); 00286 Verifier<T>::verify3(&const_func3<T>, (const Thing<T>*)&thing); 00287 Verifier<T>::verify3(&volatile_func3<T>, (volatile Thing<T>*)&thing); 00288 Verifier<T>::verify3(&const_volatile_func3<T>, (const volatile Thing<T>*)&thing); 00289 Verifier<T>::verify3(callback(static_func3<T>)); 00290 00291 Callback<T(T, T, T)> cb(static_func3); 00292 Verifier<T>::verify3(cb); 00293 cb = static_func3; 00294 Verifier<T>::verify3(cb); 00295 cb.attach(&bound_func3<T>, &thing); 00296 Verifier<T>::verify3(&cb, &Callback<T(T, T, T)>::call); 00297 Verifier<T>::verify3(&Callback<T(T, T, T)>::thunk, (void*)&cb); 00298 } 00299 00300 template <typename T> 00301 void test_dispatch4() { 00302 Thing<T> thing; 00303 Verifier<T>::verify4(static_func4<T>); 00304 Verifier<T>::verify4(&thing, &Thing<T>::member_func4); 00305 Verifier<T>::verify4((const Thing<T>*)&thing, &Thing<T>::const_member_func4); 00306 Verifier<T>::verify4((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func4); 00307 Verifier<T>::verify4((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func4); 00308 Verifier<T>::verify4(&bound_func4<T>, &thing); 00309 Verifier<T>::verify4(&const_func4<T>, (const Thing<T>*)&thing); 00310 Verifier<T>::verify4(&volatile_func4<T>, (volatile Thing<T>*)&thing); 00311 Verifier<T>::verify4(&const_volatile_func4<T>, (const volatile Thing<T>*)&thing); 00312 Verifier<T>::verify4(callback(static_func4<T>)); 00313 00314 Callback<T(T, T, T, T)> cb(static_func4); 00315 Verifier<T>::verify4(cb); 00316 cb = static_func4; 00317 Verifier<T>::verify4(cb); 00318 cb.attach(&bound_func4<T>, &thing); 00319 Verifier<T>::verify4(&cb, &Callback<T(T, T, T, T)>::call); 00320 Verifier<T>::verify4(&Callback<T(T, T, T, T)>::thunk, (void*)&cb); 00321 } 00322 00323 template <typename T> 00324 void test_dispatch5() { 00325 Thing<T> thing; 00326 Verifier<T>::verify5(static_func5<T>); 00327 Verifier<T>::verify5(&thing, &Thing<T>::member_func5); 00328 Verifier<T>::verify5((const Thing<T>*)&thing, &Thing<T>::const_member_func5); 00329 Verifier<T>::verify5((volatile Thing<T>*)&thing, &Thing<T>::volatile_member_func5); 00330 Verifier<T>::verify5((const volatile Thing<T>*)&thing, &Thing<T>::const_volatile_member_func5); 00331 Verifier<T>::verify5(&bound_func5<T>, &thing); 00332 Verifier<T>::verify5(&const_func5<T>, (const Thing<T>*)&thing); 00333 Verifier<T>::verify5(&volatile_func5<T>, (volatile Thing<T>*)&thing); 00334 Verifier<T>::verify5(&const_volatile_func5<T>, (const volatile Thing<T>*)&thing); 00335 Verifier<T>::verify5(callback(static_func5<T>)); 00336 00337 Callback<T(T, T, T, T, T)> cb(static_func5); 00338 Verifier<T>::verify5(cb); 00339 cb = static_func5; 00340 Verifier<T>::verify5(cb); 00341 cb.attach(&bound_func5<T>, &thing); 00342 Verifier<T>::verify5(&cb, &Callback<T(T, T, T, T, T)>::call); 00343 Verifier<T>::verify5(&Callback<T(T, T, T, T, T)>::thunk, (void*)&cb); 00344 } 00345 00346 00347 // Test setup 00348 utest::v1::status_t test_setup(const size_t number_of_cases) { 00349 GREENTEA_SETUP(10, "default_auto"); 00350 return verbose_test_setup_handler(number_of_cases); 00351 } 00352 00353 Case cases[] = { 00354 Case("Testing callbacks with 0 uint64s", test_dispatch0<uint64_t>), 00355 Case("Testing callbacks with 1 uint64s", test_dispatch1<uint64_t>), 00356 Case("Testing callbacks with 2 uint64s", test_dispatch2<uint64_t>), 00357 Case("Testing callbacks with 3 uint64s", test_dispatch3<uint64_t>), 00358 Case("Testing callbacks with 4 uint64s", test_dispatch4<uint64_t>), 00359 Case("Testing callbacks with 5 uint64s", test_dispatch5<uint64_t>), 00360 }; 00361 00362 Specification specification(test_setup, cases); 00363 00364 int main() { 00365 return !Harness::run(specification); 00366 }
Generated on Sun Jul 17 2022 08:25:27 by
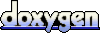