
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2016-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 #include "mbed.h" 00018 #include "rtos.h" 00019 #include "greentea-client/test_env.h" 00020 #include "unity/unity.h" 00021 #include "utest/utest.h" 00022 #include "SingletonPtr.h" 00023 #include <stdio.h> 00024 00025 #ifdef MBED_RTOS_SINGLE_THREAD 00026 #error [NOT_SUPPORTED] test not supported for single threaded enviroment 00027 #endif 00028 00029 using namespace utest::v1; 00030 00031 #define TEST_STACK_SIZE 512 00032 static uint32_t instance_count = 0; 00033 00034 class TestClass { 00035 public: 00036 TestClass() { 00037 Thread::wait(500); 00038 instance_count++; 00039 } 00040 00041 void do_something() { 00042 Thread::wait(100); 00043 } 00044 00045 ~TestClass() { 00046 instance_count--; 00047 } 00048 }; 00049 00050 static TestClass* get_test_class() 00051 { 00052 static TestClass tc; 00053 return &tc; 00054 } 00055 00056 static SingletonPtr<TestClass> test_class; 00057 00058 static void main_func_race() 00059 { 00060 get_test_class(); 00061 } 00062 00063 static void main_class_race() 00064 { 00065 test_class->do_something(); 00066 } 00067 00068 void test_case_func_race() 00069 { 00070 Callback<void()> cb(main_func_race); 00071 Thread t1(osPriorityNormal, TEST_STACK_SIZE); 00072 Thread t2(osPriorityNormal, TEST_STACK_SIZE); 00073 00074 // Start start first thread 00075 t1.start(cb); 00076 // Start second thread while the first is inside the constructor 00077 Thread::wait(250); 00078 t2.start(cb); 00079 00080 // Wait for the threads to finish 00081 t1.join(); 00082 t2.join(); 00083 00084 TEST_ASSERT_EQUAL_UINT32(1, instance_count); 00085 00086 // Reset instance count 00087 instance_count = 0; 00088 } 00089 00090 void test_case_class_race() 00091 { 00092 Callback<void()> cb(main_class_race); 00093 Thread t1(osPriorityNormal, TEST_STACK_SIZE); 00094 Thread t2(osPriorityNormal, TEST_STACK_SIZE); 00095 00096 // Start start first thread 00097 t1.start(cb); 00098 // Start second thread while the first is inside the constructor 00099 Thread::wait(250); 00100 t2.start(cb); 00101 00102 // Wait for the threads to finish 00103 t1.join(); 00104 t2.join(); 00105 00106 TEST_ASSERT_EQUAL_UINT32(1, instance_count); 00107 00108 // Reset instance count 00109 instance_count = 0; 00110 } 00111 00112 Case cases[] = { 00113 Case("function init race", test_case_func_race), 00114 Case("class init race", test_case_class_race), 00115 }; 00116 00117 utest::v1::status_t greentea_test_setup(const size_t number_of_cases) 00118 { 00119 GREENTEA_SETUP(20, "default_auto"); 00120 return greentea_test_setup_handler(number_of_cases); 00121 } 00122 00123 Specification specification(greentea_test_setup, cases, greentea_test_teardown_handler); 00124 00125 int main() 00126 { 00127 Harness::run(specification); 00128 }
Generated on Sun Jul 17 2022 08:25:27 by
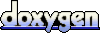