
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
make.py
00001 #! /usr/bin/env python2 00002 """ 00003 mbed SDK 00004 Copyright (c) 2011-2013 ARM Limited 00005 00006 Licensed under the Apache License, Version 2.0 (the "License"); 00007 you may not use this file except in compliance with the License. 00008 You may obtain a copy of the License at 00009 00010 http://www.apache.org/licenses/LICENSE-2.0 00011 00012 Unless required by applicable law or agreed to in writing, software 00013 distributed under the License is distributed on an "AS IS" BASIS, 00014 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 See the License for the specific language governing permissions and 00016 limitations under the License. 00017 00018 00019 TEST BUILD & RUN 00020 """ 00021 import sys 00022 import json 00023 from time import sleep 00024 from shutil import copy 00025 from os.path import join, abspath, dirname 00026 from json import load, dump 00027 00028 # Be sure that the tools directory is in the search path 00029 ROOT = abspath(join(dirname(__file__), "..")) 00030 sys.path.insert(0, ROOT) 00031 00032 from tools.utils import args_error 00033 from tools.utils import NotSupportedException 00034 from tools.paths import BUILD_DIR 00035 from tools.paths import MBED_LIBRARIES 00036 from tools.paths import RPC_LIBRARY 00037 from tools.paths import USB_LIBRARIES 00038 from tools.paths import DSP_LIBRARIES 00039 from tools.tests import TESTS, Test, TEST_MAP 00040 from tools.tests import TEST_MBED_LIB 00041 from tools.tests import test_known, test_name_known 00042 from tools.targets import TARGET_MAP 00043 from tools.options import get_default_options_parser 00044 from tools.options import extract_profile 00045 from tools.options import extract_mcus 00046 from tools.build_api import build_project 00047 from tools.build_api import mcu_toolchain_matrix 00048 from tools.build_api import mcu_toolchain_list 00049 from tools.build_api import mcu_target_list 00050 from tools.build_api import merge_build_data 00051 from utils import argparse_filestring_type 00052 from utils import argparse_many 00053 from utils import argparse_dir_not_parent 00054 from tools.toolchains import mbedToolchain, TOOLCHAIN_CLASSES, TOOLCHAIN_PATHS 00055 from tools.settings import CLI_COLOR_MAP 00056 00057 if __name__ == '__main__': 00058 # Parse Options 00059 parser = get_default_options_parser(add_app_config=True) 00060 group = parser.add_mutually_exclusive_group(required=False) 00061 group.add_argument( 00062 "-p", 00063 type=argparse_many(test_known), 00064 dest="program", 00065 help="The index of the desired test program: [0-%d]" % (len(TESTS)-1)) 00066 00067 group.add_argument( 00068 "-n", 00069 type=argparse_many(test_name_known), 00070 dest="program", 00071 help="The name of the desired test program") 00072 00073 parser.add_argument( 00074 "-j", "--jobs", 00075 type=int, 00076 dest="jobs", 00077 default=0, 00078 help="Number of concurrent jobs. Default: 0/auto (based on host machine's number of CPUs)") 00079 00080 parser.add_argument( 00081 "-v", "--verbose", 00082 action="store_true", 00083 dest="verbose", 00084 default=False, 00085 help="Verbose diagnostic output") 00086 00087 parser.add_argument( 00088 "--silent", 00089 action="store_true", 00090 dest="silent", 00091 default=False, 00092 help="Silent diagnostic output (no copy, compile notification)") 00093 00094 parser.add_argument( 00095 "-D", 00096 action="append", 00097 dest="macros", 00098 help="Add a macro definition") 00099 00100 group.add_argument( 00101 "-S", "--supported-toolchains", 00102 dest="supported_toolchains", 00103 default=False, 00104 const="matrix", 00105 choices=["matrix", "toolchains", "targets"], 00106 nargs="?", 00107 help="Displays supported matrix of MCUs and toolchains") 00108 00109 parser.add_argument( 00110 '-f', '--filter', 00111 dest='general_filter_regex', 00112 default=None, 00113 help='For some commands you can use filter to filter out results') 00114 00115 parser.add_argument( 00116 "--stats-depth", 00117 type=int, 00118 dest="stats_depth", 00119 default=2, 00120 help="Depth level for static memory report") 00121 00122 # Local run 00123 parser.add_argument("--automated", action="store_true", dest="automated", 00124 default=False, help="Automated test") 00125 parser.add_argument("--host", dest="host_test", 00126 default=None, help="Host test") 00127 parser.add_argument("--extra", dest="extra", 00128 default=None, help="Extra files") 00129 parser.add_argument("--peripherals", dest="peripherals", 00130 default=None, help="Required peripherals") 00131 parser.add_argument("--dep", dest="dependencies", 00132 default=None, help="Dependencies") 00133 parser.add_argument("--source", dest="source_dir", type=argparse_filestring_type, 00134 default=None, help="The source (input) directory", action="append") 00135 parser.add_argument("--duration", type=int, dest="duration", 00136 default=None, help="Duration of the test") 00137 parser.add_argument("--build", dest="build_dir", type=argparse_dir_not_parent(ROOT), 00138 default=None, help="The build (output) directory") 00139 parser.add_argument("-N", "--artifact-name", dest="artifact_name", 00140 default=None, help="The built project's name") 00141 parser.add_argument("-d", "--disk", dest="disk", 00142 default=None, help="The mbed disk") 00143 parser.add_argument("-s", "--serial", dest="serial", 00144 default=None, help="The mbed serial port") 00145 parser.add_argument("-b", "--baud", type=int, dest="baud", 00146 default=None, help="The mbed serial baud rate") 00147 group.add_argument("-L", "--list-tests", action="store_true", dest="list_tests", 00148 default=False, help="List available tests in order and exit") 00149 00150 # Ideally, all the tests with a single "main" thread can be run with, or 00151 # without the usb, dsp 00152 parser.add_argument("--rpc", 00153 action="store_true", dest="rpc", 00154 default=False, help="Link with RPC library") 00155 00156 parser.add_argument("--usb", 00157 action="store_true", 00158 dest="usb", 00159 default=False, 00160 help="Link with USB Device library") 00161 00162 parser.add_argument("--dsp", 00163 action="store_true", 00164 dest="dsp", 00165 default=False, 00166 help="Link with DSP library") 00167 00168 parser.add_argument("--testlib", 00169 action="store_true", 00170 dest="testlib", 00171 default=False, 00172 help="Link with mbed test library") 00173 00174 parser.add_argument("--build-data", 00175 dest="build_data", 00176 default=None, 00177 help="Dump build_data to this file") 00178 00179 # Specify a different linker script 00180 parser.add_argument("-l", "--linker", dest="linker_script", 00181 type=argparse_filestring_type, 00182 default=None, help="use the specified linker script") 00183 00184 options = parser.parse_args() 00185 00186 # Only prints matrix of supported toolchains 00187 if options.supported_toolchains: 00188 if options.supported_toolchains == "matrix": 00189 print mcu_toolchain_matrix(platform_filter=options.general_filter_regex) 00190 elif options.supported_toolchains == "toolchains": 00191 toolchain_list = mcu_toolchain_list() 00192 # Only print the lines that matter 00193 for line in toolchain_list.split("\n"): 00194 if not "mbed" in line: 00195 print line 00196 elif options.supported_toolchains == "targets": 00197 print mcu_target_list() 00198 exit(0) 00199 00200 # Print available tests in order and exit 00201 if options.list_tests is True: 00202 print '\n'.join(map(str, sorted(TEST_MAP.values()))) 00203 sys.exit() 00204 00205 # force program to "0" if a source dir is specified 00206 if options.source_dir is not None: 00207 p = 0 00208 else: 00209 # Program Number or name 00210 p = options.program 00211 00212 # If 'p' was set via -n to list of numbers make this a single element integer list 00213 if type(p) != type([]): 00214 p = [p] 00215 00216 # Target 00217 if options.mcu is None : 00218 args_error(parser, "argument -m/--mcu is required") 00219 mcu = extract_mcus(parser, options)[0] 00220 00221 # Toolchain 00222 if options.tool is None: 00223 args_error(parser, "argument -t/--tool is required") 00224 toolchain = options.tool[0] 00225 00226 if (options.program is None) and (not options.source_dir): 00227 args_error(parser, "one of -p, -n, or --source is required") 00228 00229 if options.source_dir and not options.build_dir: 00230 args_error(parser, "argument --build is required when argument --source is provided") 00231 00232 00233 if options.color: 00234 # This import happens late to prevent initializing colorization when we don't need it 00235 import colorize 00236 if options.verbose: 00237 notify = mbedToolchain.print_notify_verbose 00238 else: 00239 notify = mbedToolchain.print_notify 00240 notify = colorize.print_in_color_notifier(CLI_COLOR_MAP, notify) 00241 else: 00242 notify = None 00243 00244 if not TOOLCHAIN_CLASSES[toolchain].check_executable(): 00245 search_path = TOOLCHAIN_PATHS[toolchain] or "No path set" 00246 args_error(parser, "Could not find executable for %s.\n" 00247 "Currently set search path: %s" 00248 %(toolchain,search_path)) 00249 00250 # Test 00251 build_data_blob = {} if options.build_data else None 00252 for test_no in p: 00253 test = Test(test_no) 00254 if options.automated is not None: test.automated = options.automated 00255 if options.dependencies is not None: test.dependencies = options.dependencies 00256 if options.host_test is not None: test.host_test = options.host_test; 00257 if options.peripherals is not None: test.peripherals = options.peripherals; 00258 if options.duration is not None: test.duration = options.duration; 00259 if options.extra is not None: test.extra_files = options.extra 00260 00261 if not test.is_supported(mcu, toolchain): 00262 print 'The selected test is not supported on target %s with toolchain %s' % (mcu, toolchain) 00263 sys.exit() 00264 00265 # Linking with extra libraries 00266 if options.rpc: test.dependencies.append(RPC_LIBRARY) 00267 if options.usb: test.dependencies.append(USB_LIBRARIES) 00268 if options.dsp: test.dependencies.append(DSP_LIBRARIES) 00269 if options.testlib: test.dependencies.append(TEST_MBED_LIB) 00270 00271 build_dir = join(BUILD_DIR, "test", mcu, toolchain, test.id) 00272 if options.source_dir is not None: 00273 test.source_dir = options.source_dir 00274 build_dir = options.source_dir 00275 00276 if options.build_dir is not None: 00277 build_dir = options.build_dir 00278 00279 try: 00280 bin_file = build_project(test.source_dir, build_dir, mcu, toolchain, 00281 set(test.dependencies), 00282 linker_script=options.linker_script, 00283 clean=options.clean, 00284 verbose=options.verbose, 00285 notify=notify, 00286 report=build_data_blob, 00287 silent=options.silent, 00288 macros=options.macros, 00289 jobs=options.jobs, 00290 name=options.artifact_name, 00291 app_config=options.app_config, 00292 inc_dirs=[dirname(MBED_LIBRARIES)], 00293 build_profile=extract_profile(parser, 00294 options, 00295 toolchain), 00296 stats_depth=options.stats_depth) 00297 print 'Image: %s'% bin_file 00298 00299 if options.disk: 00300 # Simple copy to the mbed disk 00301 copy(bin_file, options.disk) 00302 00303 if options.serial: 00304 # Import pyserial: https://pypi.python.org/pypi/pyserial 00305 from serial import Serial 00306 00307 sleep(TARGET_MAP[mcu].program_cycle_s) 00308 00309 serial = Serial(options.serial, timeout = 1) 00310 if options.baud: 00311 serial.setBaudrate(options.baud) 00312 00313 serial.flushInput() 00314 serial.flushOutput() 00315 00316 try: 00317 serial.sendBreak() 00318 except: 00319 # In linux a termios.error is raised in sendBreak and in setBreak. 00320 # The following setBreak() is needed to release the reset signal on the target mcu. 00321 try: 00322 serial.setBreak(False) 00323 except: 00324 pass 00325 00326 while True: 00327 c = serial.read(512) 00328 sys.stdout.write(c) 00329 sys.stdout.flush() 00330 00331 except KeyboardInterrupt, e: 00332 print "\n[CTRL+c] exit" 00333 except NotSupportedException as e: 00334 print "\nCould not compile for %s: %s" % (mcu, str(e)) 00335 except Exception,e: 00336 if options.verbose: 00337 import traceback 00338 traceback.print_exc(file=sys.stdout) 00339 else: 00340 print "[ERROR] %s" % str(e) 00341 00342 sys.exit(1) 00343 if options.build_data: 00344 merge_build_data(options.build_data, build_data_blob, "application")
Generated on Sun Jul 17 2022 08:25:27 by
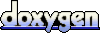