
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #define BUF_SIZE 10 00003 00004 Thread thread; 00005 CircularBuffer<char, BUF_SIZE> buf; 00006 int bytes = 0; 00007 00008 void print_string(char data_stream[] = "Thread") 00009 { 00010 while (!buf.full()) { 00011 buf.push(data_stream[bytes++ % 6]); 00012 } 00013 } 00014 00015 void print_thread() 00016 { 00017 while (true) { 00018 wait(1); 00019 print_string(); 00020 } 00021 } 00022 00023 int main() 00024 { 00025 printf("\n\n*** RTOS - CircularBuffer basic example ***\n"); 00026 00027 thread.start(print_thread); 00028 char data; 00029 while (true) { 00030 printf ("\n\rBuffer contents: "); 00031 while (!buf.empty()) { 00032 buf.pop(data); 00033 printf("%c", data); 00034 } 00035 printf("\n"); 00036 wait(1); 00037 } 00038 }
Generated on Sun Jul 17 2022 08:25:25 by
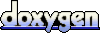